2) for both triangle and diamond, first display a triangle of requested size, and then if the choice is a diamond, add the bottom part 3) displays the shape We want to create a program that draws a diamond or a triangle with a size that the user selects using a specific character that will be entered at the keyboard. Here are two examples: To write this program, we will assume that we have the following functions: 1) void instructions() II This function describes the program and how it works A triangle of size 4, using *: 2) int menu() I // This function will return a choice to the main ; 1) draw triangle, 2) draw diamond, and 3) quit 3) draw_shape(int choice) I/ This function calls on appropriate function depending on the choice to draw a shape A diamond of size 4, using *: 4) int get_size() II This function will return the size of the shape, same function for either of the shapes *** 5) char get_char() // This function will ask users to select a character that will be used to draw the shape *** What do we need for this program? 6) void draw_triangle(int size, char c) I/ This function draws a triangle of size size using character e Problem definition We first need to clearly define the problem, the inputs and the outputs. Inputs: 1) a choice to draw one of the two shapes or to quit the program, 2) a character choice which will be used for drawing the selected shape, and 3) the size of the shape 7) void draw diamond(int size, char c) II This function first calls draw_triangle, then draw_bottom to draw the diamond 8) void draw_bottom(int size, char c) II This function actually draws an upside down triangle of size size-l as the bottom of the diamond Output: One of these will be the output: 1) a triangle, 2) a diamond, 3) quit Now that we have defined the functions, it is time to design the algorithm for the program. However, we can write the main part of the program. Analysis of the problem We need to break down the tasks that this program is supposed to complete. The main tasks are: 1) ask for the input data int main () { int choice;
2) for both triangle and diamond, first display a triangle of requested size, and then if the choice is a diamond, add the bottom part 3) displays the shape We want to create a program that draws a diamond or a triangle with a size that the user selects using a specific character that will be entered at the keyboard. Here are two examples: To write this program, we will assume that we have the following functions: 1) void instructions() II This function describes the program and how it works A triangle of size 4, using *: 2) int menu() I // This function will return a choice to the main ; 1) draw triangle, 2) draw diamond, and 3) quit 3) draw_shape(int choice) I/ This function calls on appropriate function depending on the choice to draw a shape A diamond of size 4, using *: 4) int get_size() II This function will return the size of the shape, same function for either of the shapes *** 5) char get_char() // This function will ask users to select a character that will be used to draw the shape *** What do we need for this program? 6) void draw_triangle(int size, char c) I/ This function draws a triangle of size size using character e Problem definition We first need to clearly define the problem, the inputs and the outputs. Inputs: 1) a choice to draw one of the two shapes or to quit the program, 2) a character choice which will be used for drawing the selected shape, and 3) the size of the shape 7) void draw diamond(int size, char c) II This function first calls draw_triangle, then draw_bottom to draw the diamond 8) void draw_bottom(int size, char c) II This function actually draws an upside down triangle of size size-l as the bottom of the diamond Output: One of these will be the output: 1) a triangle, 2) a diamond, 3) quit Now that we have defined the functions, it is time to design the algorithm for the program. However, we can write the main part of the program. Analysis of the problem We need to break down the tasks that this program is supposed to complete. The main tasks are: 1) ask for the input data int main () { int choice;
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.2: Returning A Single Value
Problem 5E: (General math) a. The volume, V, of a cylinder is given by this formula: V=r2L r is the cylinder’s...
Related questions
Question
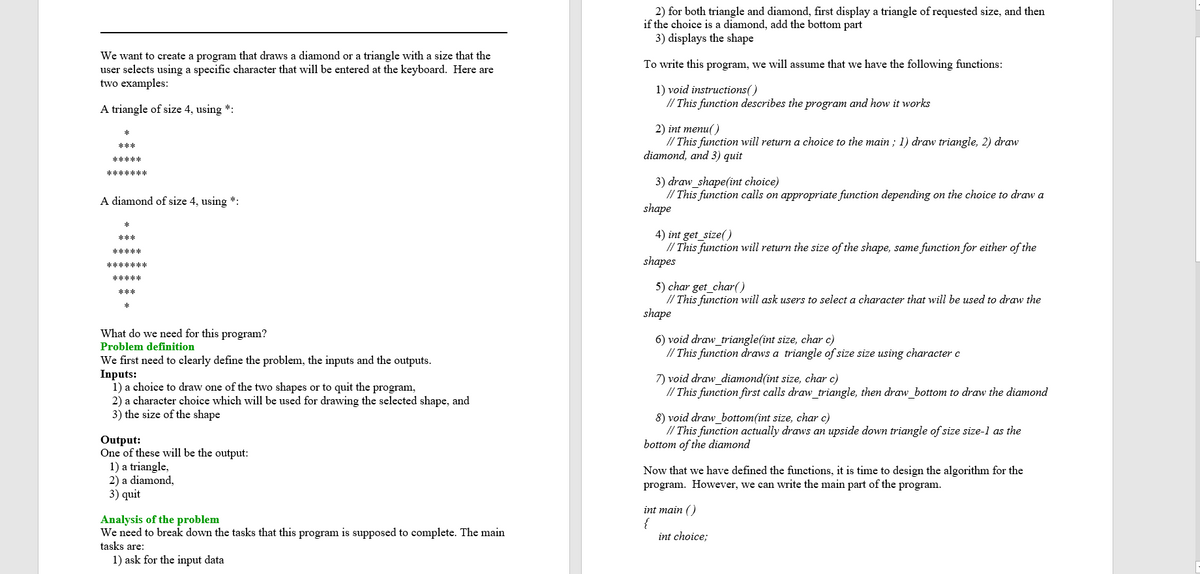
Transcribed Image Text:2) for both triangle and diamond, first display a triangle of requested size, and then
if the choice is a diamond, add the bottom part
3) displays the shape
We want to create a program that draws a diamond or a triangle with a size that the
user selects using a specific character that will be entered at the keyboard. Here are
two examples:
To write this program, we will assume that we have the following functions:
1) void instructions()
// This function describes the program and how it works
A triangle of size 4, using *:
2) int menu()
// This function will return a choice to the main ; 1) draw triangle, 2) draw
diamond, and 3) quit
***
*****
*******
3) draw_shape(int choice)
// This function calls on appropriate function depending on the choice to draw a
shape
A diamond of size 4, using *:
4) int get_size()
// This function will return the size of the shape, same function for either of the
shapes
***
*****
*******
*****
5) char get_char()
// This function will ask users to select a character that will be used to draw the
shape
***
What do we need for this program?
6) void draw_triangle(int size, char c)
// This function draws a triangle of size size using character c
Problem definition
We first need to clearly define the problem, the inputs and the outputs.
Inputs:
1) a choice to draw one of the two shapes or to quit the program,
2) a character choice which will be used for drawing the selected shape, and
3) the size of the shape
7) void draw_diamond(int size, char c)
// This function first calls draw triangle, then draw bottom to draw the diamond
8) void draw_bottom(int size, char c)
// This function actually draws an upside down triangle of size size-1 as the
bottom of the diamond
Output:
One of these will be the output:
1) a triangle,
2) a diamond,
3) quit
Now that we have defined the functions, it is time to design the algorithm for the
program. However, we can write the main part of the program.
Analysis of the problem
We need to break down the tasks that this program is supposed to complete. The main
tasks are:
1) ask for the input data
int main ()
{
int choice;
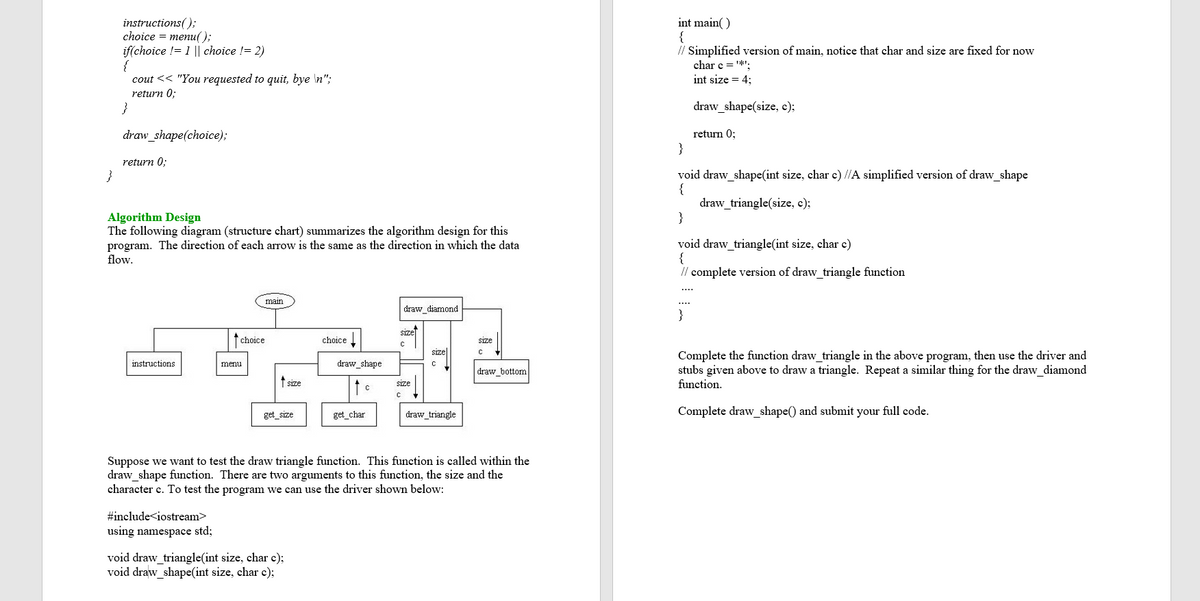
Transcribed Image Text:instructions();
choice %3D menи();
if(choice != 1 || choice != 2)
{
cout << "You requested to quit, bye \n";
int main( )
{
// Simplified version of main, notice that char and size are fixed for now
char c = *.
int size = 4;
return 0;
}
draw_shape(size, c);
draw_shape(choice);
return 0:
}
return 0:
void draw_shape(int size, char c) /IA simplified version of draw_shape
{
draw_triangle(size, c);
}
Algorithm Design
The following diagram (structure chart) summarizes the algorithm design for this
program. The direction of each arrow is the same as the direction in which the data
flow.
void draw triangle(int size, char c)
{
// complete version of draw_triangle function
....
main
draw_diamond
}
sizel
choice
choice
size
size|
Complete the function draw triangle in the above program, then use the driver and
stubs given above to draw a triangle. Repeat a similar thing for the draw_diamond
function.
instructions
draw_shape
menu
draw_bottom
t size
size
get_size
get_char
draw_triangle
Complete draw_shape() and submit your full code.
Suppose we want to test the draw triangle function. This function is called within the
draw shape function. There are two arguments to this function, the size and the
character c. To test the program we can use the driver shown below:
#include<iostream>
using namespace std;
void draw_triangle(int size, char c);
void draw_shape(int size, char c);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
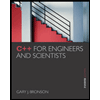
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
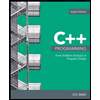
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
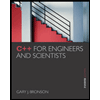
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
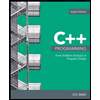
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning