Note for programmer: 1. Do this in c++ programming language and use oop concepts. 2. Look at the pic attatched first 3. Write a separate main() function as a driver for each question. The driver should demonstrate all the required functionalities of a question. 4. Your code must be generic, well-commented, and well-organized. It should not have compilation errors. Each class should have private data members. Each class should also contain constructors (default, parametrized, and copy constructor if needed), a destructor, setters, and getters. Each class should be implemented in separate .h and .cpp files. .................................................................................................................................................. Description of Program: Make sure to use the data encapsulation techniques, where necessary. The details for each class are given below. Project: Each project has a “project ID” and “project details” (write any random details about the project here). There is non-parameterized constructor to instantiate a Project object. Using getters and setters, get the values and set the “id” and “project details”. The ID must be at least 1 or greater than this. “Show details” function must return the Project ID and Project Details as a string. Employee: There is a “name”, “employee id” and a “project” associated with each employee. There are getters and setters that should be used to get and set the values of “employee name” and “id” and should validate the input too. The ID cannot be less than 1. “Show details” function must return the Employee ID, name, salary, Project ID and Project Details as a string. Salaried Employee: Getter and setter are used to set the “monthly salary”. Monthly salary must be above Rs. 30,000. “GetSalary” returns the monthly salary as an integer. “Show details” function must return the Employee ID, name, salary, Project ID and Project Details as a string. Commission Employee: Gross sales show the total sales achieved by the employee. Getters and setters are used to get and set the gross sales and base salary values. Base salary must be above 20,000. Gross sales must be a positive integer value. 1.5% Commission is only given to the employee if the gross sales exceed Rs. 100, 000 and is calculated using the formula: Commission = gross sales * 0.015 “calculateSalary” function calculates the total salary of the employee and sets the “total salary”vattribute to the calculated salary using the following formula: Total salary = base salary + commission “GetTotalSalary” returns the total salary as a floating number. “Show details” function must return the Employee ID, name, Project ID and Project Details as a string. Hourly Employee: Getters and setters are used to retrieve and set the values of “no of hours” and “hourly rate”. No of hours worked must be at least 30 and the hourly rate must be at least Rs. 150 “calculateSalary” function calculates the total salary using the given formula and sets the “total salary” attribute to the result of the calculation: Total salary = no of hours * hourly rate “GetTotalSalary” returns the total salary as an integer. “Show details” function must return the Employee ID, name, salary, Project ID and Project Details as a string. To Do: Write a driver code to test all the functions; you can hardcode the values instead of taking the inputs from the user. You can also make other utility functions as needed if that makes your program simpler and/or easier to read. Your program should not crash on the edge cases or illegal inputs.
Note for programmer:
1. Do this in c++ programming language and use oop concepts.
2. Look at the pic attatched first
3. Write a separate main() function as a driver for each question. The driver should demonstrate all the required functionalities of a question.
4. Your code must be generic, well-commented, and well-organized. It should not have compilation errors. Each class should have private data members. Each class should also contain constructors (default, parametrized, and copy constructor if needed), a destructor, setters, and getters. Each class should be implemented in separate .h and .cpp
files.
..................................................................................................................................................
Description of Program:
Make sure to use the data encapsulation techniques, where necessary. The details for each class are given below.
Project:
Each project has a “project ID” and “project details” (write any random details about the project here).
There is non-parameterized constructor to instantiate a Project object. Using getters and setters, get the values and set the “id” and “project details”. The ID must be at least 1 or greater than this. “Show details” function must return the Project ID and Project Details as a string.
Employee:
There is a “name”, “employee id” and a “project” associated with each employee.
There are getters and setters that should be used to get and set the values of “employee name” and “id” and should validate the input too. The ID cannot be less than 1. “Show details” function must return the Employee ID, name, salary, Project ID and Project Details as a string.
Salaried Employee:
Getter and setter are used to set the “monthly salary”. Monthly salary must be above Rs. 30,000.
“GetSalary” returns the monthly salary as an integer. “Show details” function must return the Employee ID, name, salary, Project ID and Project Details as a string.
Commission Employee:
Gross sales show the total sales achieved by the employee. Getters and setters are used to get and
set the gross sales and base salary values.
Base salary must be above 20,000. Gross sales must be a positive integer value.
1.5% Commission is only given to the employee if the gross sales exceed Rs. 100, 000 and is
calculated using the formula:
Commission = gross sales * 0.015
“calculateSalary” function calculates the total salary of the employee and sets the “total salary”vattribute to the calculated salary using the following formula:
Total salary = base salary + commission
“GetTotalSalary” returns the total salary as a floating number. “Show details” function must return the Employee ID, name, Project ID and Project Details as a string.
Hourly Employee:
Getters and setters are used to retrieve and set the values of “no of hours” and “hourly rate”. No of hours worked must be at least 30 and the hourly rate must be at least Rs. 150 “calculateSalary” function calculates the total salary using the given formula and sets the “total salary” attribute to the result of the calculation:
Total salary = no of hours * hourly rate
“GetTotalSalary” returns the total salary as an integer. “Show details” function must return the Employee ID, name, salary, Project ID and Project Details as a string.
To Do:
Write a driver code to test all the functions; you can hardcode the values instead of taking the inputs from the user. You can also make other utility functions as needed if that makes your program simpler and/or easier to read. Your program should not crash on the edge cases or illegal inputs.
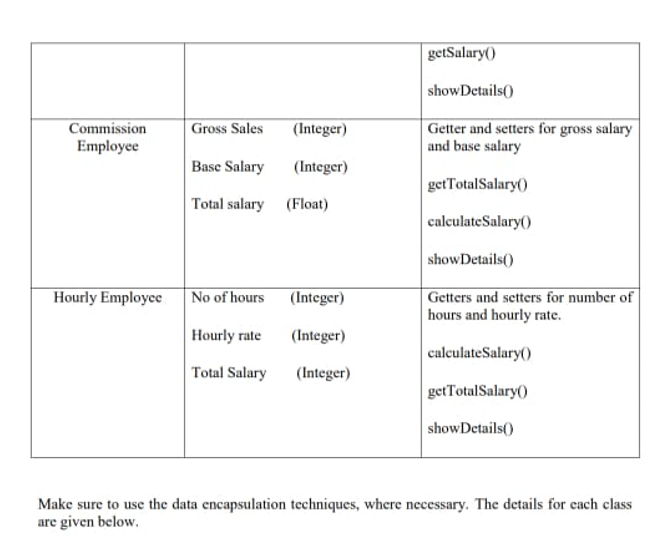
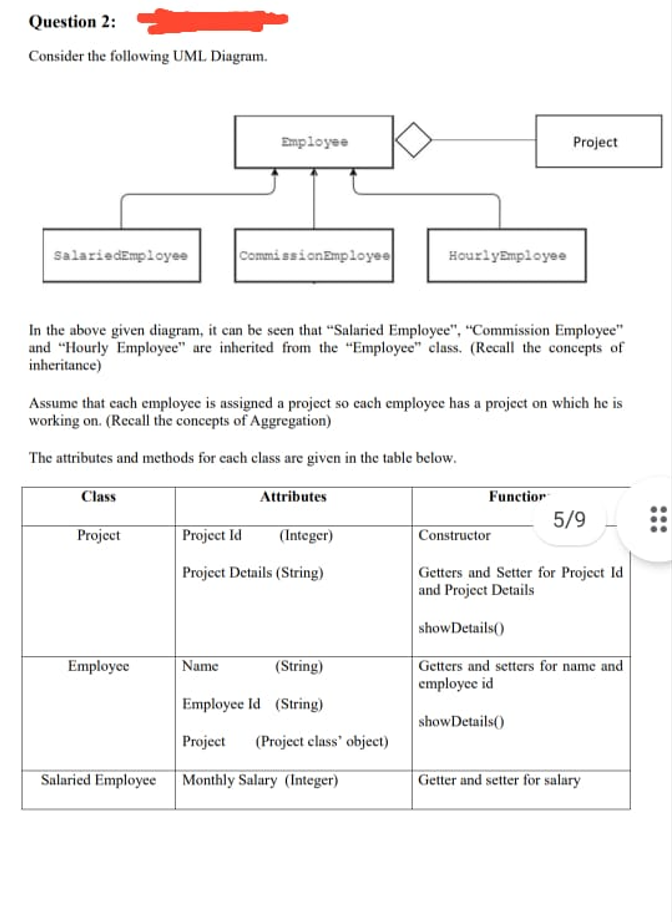

Step by step
Solved in 6 steps with 3 images

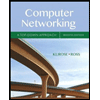
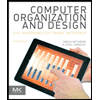
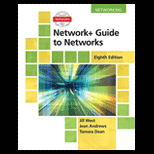
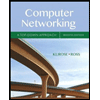
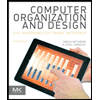
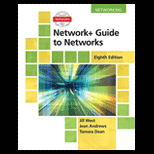
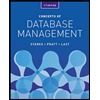
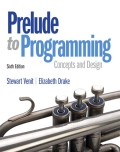
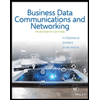