IN c++ See the specification of the HugeInt class. Complete the implementation of this class. Provide a driver to demonstrate the features of the HugeInt class.
IN c++ See the specification of the HugeInt class.
Complete the implementation of this class.
Provide a driver to demonstrate the features of the HugeInt class.
HugeInt.h
#ifndef HUGEINT_H_
#define HUGEINT_H_
enum SignType {PLUS, MINUS};
class HugeInt{
private:
struct Node
{
int digit; // SINGLE DIGIT ONLY ie: (0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
Node *next; // Next More Significant Digit
};
/* Integer 345 is stored in List: 3 <- 4 <- 5 <- digits
Integer 123 is stored in List: 1 <- 2 <- 3 <- digits
To add: add the least significant digits, potentially
keeping track of a carry value. Then move to the next
left nodes in both HugeInts. Add these and the carry.
Create a new carry value. Continue until both HugeInt
lists are empty. If one list is empty, keep processing
the non-empty list.
*/
Node *digits; //Linked list of digit nodes
SignType sign; //enum of the sign of the HugeInt
int numDigits; //Number of digits in the HugeInt
void makeEmpty(); //clear the linked list of digits
public:
HugeInt();
~HugeInt();
bool operator<(HugeInt second);//compare for less than. return true/false
bool operator==(HugeInt second);//compare for equal. return true/false
HugeInt operator+(HugeInt second);//add
HugeInt operator-(HugeInt second);//subtract
HugeInt operator*(HugeInt second);//multiply
HugeInt operator/(HugeInt second);//divide
void insertDigits(int); //insert integer number. Insert each digit separately!!!!!!!
void write(ofstream&); //Write the digits to a file. Pass ofstream as arg
};
#endif
HugeInt Class
The HugeInt class represents an integer of very many digits.
The digits are stored in a linked list of nodes.
Each node has a single integer digit and a pointer to the next, more significant digit.
A single pointer of type Node points to the least significant digit.
The digits are stored in order from the least significant digit to the most significant digit.
The HugeInt objects may be added, subtracted, multiplied, and divided. This must be done with operator overloading.
The Hugeint objects may be compared for equal or less than. This must be done with operator overloading.
A HugeInt Object may be written to a file using the write method.
There are two ways to implement multiplication and division. One is to use repeated addition (or subtraction); the other is to simulate how we do multiplication (and division) by hand. You may use either method.
HugeInt Driver
The HugeInt driver should create several HugeInt objects and demonstrate all features.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

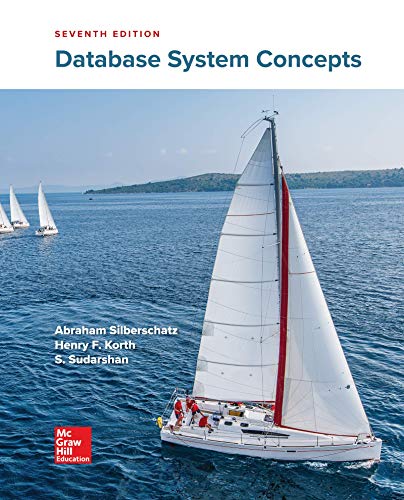
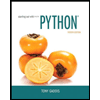
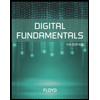
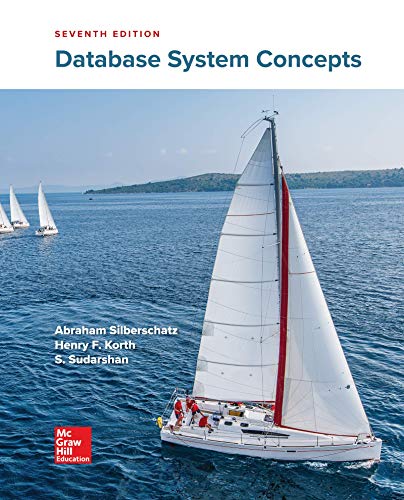
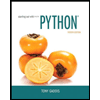
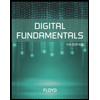
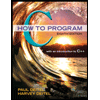
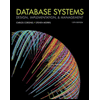
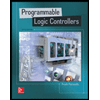