Now, modify it to do the following: modify the while loop to utilize tolower() or toupper().
Now, modify it to do the following: modify the while loop to utilize tolower() or toupper().
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
#include <iostream>
#include <cmath>
using namespace std;
// declare functions
void display_menu();
void convert_temp();
double to_celsius(double fahrenheit);
double to_fahrenheit(double celsius);
int main() {
cout << "Convert Temperatures\n\n";
display_menu();
char again = 'y';
while (again == 'y')
{
convert_temp();
cout << "Convert another temperature? (y/n): ";
cin >> again;
cout << endl;
}
cout << "Bye!\n";
}
// define functions
void display_menu()
{
cout << "MENU\n"
<< "1. Fahrenheit to Celsius\n"
<< "2. Celsius to Fahrenheit\n\n";
}
void convert_temp()
{
int option;
cout << "Enter a menu option: ";
cin >> option;
double f = 0.0;
double c = 0.0;
switch (option)
{
case 1:
cout << "Enter degrees Fahrenheit: ";
cin >> f;
c = to_celsius(f);
c = round(c * 10) / 10; // round to one decimal place
cout << "Degrees Celsius: " << c << endl;
break;
case 2:
cout << "Enter degrees Celsius: ";
cin >> c;
f = to_fahrenheit(c);
f = round(f * 10) / 10; // round to one decimal place
cout << "Degrees Fahrenheit: " << f << endl;
break;
default:
cout << "You must enter a valid menu number.\n";
break;
}
}
double to_celsius(double fahrenheit)
{
double celsius = (fahrenheit - 32.0) * 5.0 / 9.0;
return celsius;
}
double to_fahrenheit(double celsius)
{
double fahrenheit = celsius * 9.0 / 5.0 + 32.0;
return fahrenheit;
}
- Now, modify it to do the following:
- modify the while loop to utilize tolower() or toupper().
-
- Create two more functions (options #3 and #4 in your menu) by taking the to_celsius() and to_fahrenheit() functions and modifying them to use reference variables instead of normal pass by value variables. Name them:
-
-
- to_celsius_ref()
- to_fahrenheit_ref()
- Create another two functions (options #5 and #6 in your menu) by taking the to_celsius() and to_fahrenheit() functions and modifying them to use pointers instead of normal pass by value variables. Name them:
-
-
-
- to_celsius_ptr()
- to_fahrenheit_ptr()
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
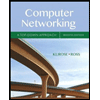
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
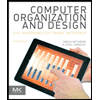
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
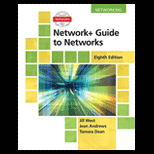
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
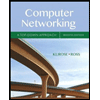
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
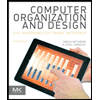
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
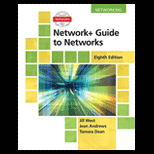
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
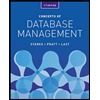
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
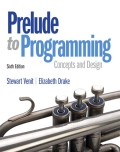
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
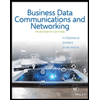
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY