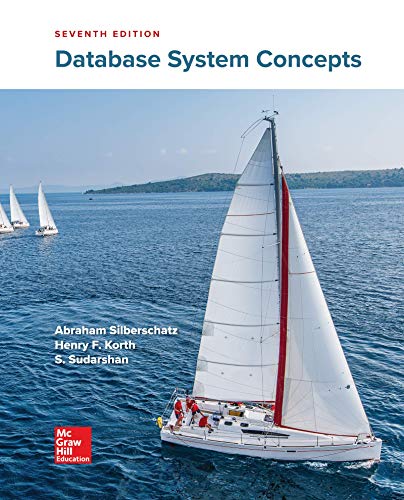
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Rewrite the code using "while Loop" instead of "for Loop". Output must remain the same.
CODE:
import java.util.*;
public class main {
public static void main(String[] args) {
int[] numbers = new int[10];
int[] numbers1 = new int[10];
for(int i=0; i < numbers.length; i++)
numbers1[i] = i * 2;
for(int i=0; i < numbers1.length; i++)
System.out.print(numbers1[i] + " ");
System.out.println();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java programming: What's the output of the following program... a.) Compilation Error b.) 97~B~C~D~E~102~G~ c.) 65~b~C~d~E~70~G~ d.) a~66~C~68~E~f~G~arrow_forwardclass Output{public static void main(String args[]){byte a[] = { 65, 66, 67, 68, 69, 70 };byte b[] = { 71, 72, 73, 74, 75, 76 };System.arraycopy(a , 0, b, 0, a.length);System.out.print(new String(a) + " " + new String(b));}} The output of the above Java code is ?arrow_forwardpublic class Main { static int findPosSum(int A[], int N) { if (N <= 0) return 0; { if(A[N-1]>0) return (findPosSum(A, N - 1) + A[N - 1]); else return findPosSum(A, N - 1); } } public static void main(String[] args) { int demo[] = { 11, -22, 33, -4, 25,12 }; System.out.println(findPosSum(demo, demo.length)); } } Consider the recursive function you wrote in the previous problem. Suppose the initial call to the function has an array of N elements, how many recursive calls will be made? How many statements are executed in each call? What is the total number of statements executed for all recursive calls? What is the big O for this function?arrow_forward
- 1. When you run the following code, what would happen?public class Question1 {public static void main(String arg[]){int i;int [] A = new int[10];int count = 0;int temp = 10;for (i=0; i < A.length; i++)if (A[i] < temp) count++;System.out.println(count);}}(a) It finds the smallest value and stores it in temp.(b) It finds the largest value and stores it in temp.(c) It counts the number of elements equal to the smallest value in list.(d) It counts the number of elements in list that are less than temp.arrow_forwardpublic class ArraySection { static void arraySection(int a[], int b[]) { int k = 0; int [] c = new int[a.length]; for(int i = 0; i < a.length; i++) { for(int j = 0; j < b.length; j++) if(a[i] == b[j]) c[k++] = a[i]; } for(int i = 0; i < k; i++) System.out.println(c[i]); System.out.println(); } public static void main(String[] args) { int a[] = { 1, 2, 3, 4, 5 }; int b[] = { 0, 2, 4,5 }; arraySection(a,b); }} Calculate the algorithm step number and algorithm time complexity of the above program?arrow_forwarddef findOccurrences(s, ch): lst = [] for i in range(0, len(s)): if a==s[i]: lst.append(i) return lst Use the code above instead of enumerate in the code posted below. n=int(input("Number of rounds of Hangman to be played:")) for i in range(0,n): word = input("welcome!") guesses = '' turns = int(input("Enter the number of failed attempts allowed:")) def hangman(word): secrete_word = "-" * len(word) print(" the secrete word " + secrete_word) user_input = input("Guess a letter: ") if user_input in word: occurences = findOccurrences(word, user_input) for index in occurences: secrete_word = secrete_word[:index] + user_input + secrete_word[index + 1:] print(secrete_word) else: user_input = input("Sorry that letter was not found, please try again: ") def findOccurrences(s, ch): return [i for i, letter in enumerate(s) if letter == ch] *** enumerate not discussed in…arrow_forward
- JAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forwardRewrite code in Javaarrow_forwardIdentify the errors in the following code segment * provide the correction for each error. Import Java.util.scanner; public class Readable { public Static void main(String[] args) { Scanner KB ; int shares; double averagePrice = 14.67; Shares = KB.nextDouble(); System.out.println("There were " , shares + " shares sold at $" + averagePrice + " per share."); } }arrow_forward
- import javax.swing.JOptionPane; public class Addition{public static void main( String args[] ){for (int i = 0; i < 10; i++) {String Number = JOptionPane.showInputDialog( "Enter a number" );int number = Integer.parseInt( Number );int sum = number;JOptionPane.showMessageDialog( null, "The sum is " + sum,"Sum of 10 numbers", JOptionPane.PLAIN_MESSAGE );}}} I want to add the number that was entered but I don't know how to do that. Do you know how to add the entered number?arrow_forwardimport java.util.* ; public class Averager { public static void main(String[] args) { //a two dimensional array int[][] a = {{5, 9, 3, 2, 14}, {77, 44, 22, 15, 99}, {14, 2, 3, 9, 5}, {88, 15, 17, 121, 33}} ; System.out.printf("Average = %.2f\n", average(a)) ; System.out.println("EXPECTED:") ; System.out.println("Average = 29.85") ; System.out.printf("Average of evens = %.2f\n", averageEvens(a)) ; System.out.println("EXPECTED:") ; System.out.println("Average of evens = 26.57") ; Random random = new Random(1) ; a = new int[100][1] ; for (int i = 0 ; i < 100 ; i++) a[i][0] = random.nextInt(1000) ; System.out.println("For an array of random values in range 0 to 999:") ; System.out.printf("Average = %.2f\n", average(a)) ; System.out.printf("Average of evens = %.2f\n", averageEvens(a)) ; } /** Find the average of all elements of a two-dimensional array @param aa the two…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
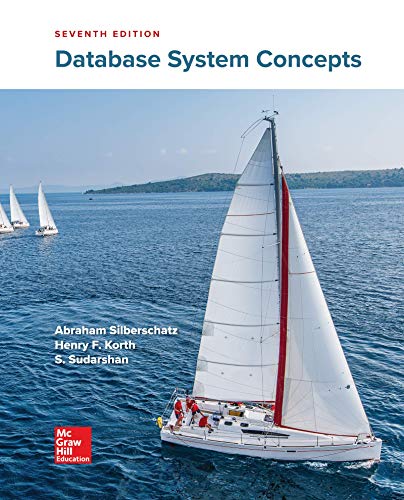
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
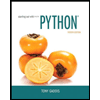
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
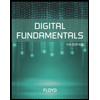
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
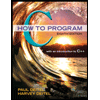
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
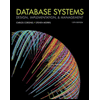
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
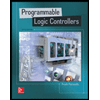
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education