Number conversion is a method that is often used in world of computing. This method aim to simplify the analysis and calculation process. Bibi, a novice data scientist, is looking at the series of data with irregular values. Each Ai value that Bibi sees can be anywhere from −10^18 to 10^18. Seeing such a large range of numeric values, Bibi asks you to do conversion on each value in the series so every new values is as minimum as possible while still maintaining larger, smaller and equal property with every other values in the initial series. The smallest number Bibi allow is 1. Help Bibi convert the number according to the criteria above. For example, there is a series with the values 99, -30, 0, 20, 1, 20. • the lowest value -30 is converted to 1. • second lowest value 0 is converted to 2. • third lowest value 1 is converted to 3. • lowest fourth value 20 is converted to 4. • the highest value 99 is converted to 5. Therefore, you get the new sequence 5, 1, 2, 4, 3, 4. Format Input There are T test cases. Each testcase contains the integer N ,which represents the amount of data in the series. On the next line there are N numbers which represents the value of each number in the series. Format Output Output T line with format “Case #X:”, where X represents the testcase number, then followed by N converted numbers. Constraints • 1 ≤ T ≤ 20 • 1 ≤ N ≤ 1000 • −10^18 ≤ Ai ≤ 10^18 Sample Input (standard input) 3 5 1 999 888 100 400 8 5 5 1 6 1 6 1 1 5 -30 -30 30 0 -10000 Sample Output (standard output) Case #1: 1 5 4 2 3 Case #2: 2 2 1 3 1 3 1 1 Case #3: 2 2 4 3 1 My code in C: #include void bubblesort(int array[1000],int n){ for(int i=1;i=i;j--){ if(array[j-1]>array[j]){ int temp = array[j]; array[j] = array[j-1]; array[j-1] = temp; } } } } binarysearch(int array[100],int n, int key, int low, int high){ int mid; low=0,high=n-1; while(low<=high){ if(array[mid]>key){ high=mid-1; } else if(array[mid]high){ return -1; } } int main(){ int tc,array[1000],low,high; int key; scanf("%d",&tc); for(int i=1;i<=tc;i++){ int n; scanf("%d",&n); for(int j=0;j
Good evening Sir. How to fix this code in C Sir? I am stuck. Don't use other library except #include<stdio.h>, use bubblesort, and use binary search. Sir, how to sort and search simultaneously? So I want, for example, 1 999 888 100 400, it's sorted into 1 100 400 888 999, so the order become 1 2 3 4 5, then at the same time what we input is considered to be searched. If we input 1 999 888 100 400, results will be 1 5 4 2 3. Thank you Sir.
Number Conversion
Number conversion is a method that is often used in world of computing. This method aim to simplify the analysis and calculation process. Bibi, a novice data scientist, is looking at the series of data with irregular values. Each
For example, there is a series with the values 99, -30, 0, 20, 1, 20.
• the lowest value -30 is converted to 1.
• second lowest value 0 is converted to 2.
• third lowest value 1 is converted to 3.
• lowest fourth value 20 is converted to 4.
• the highest value 99 is converted to 5.
Therefore, you get the new sequence 5, 1, 2, 4, 3, 4.
Format Input
There are T test cases. Each testcase contains the integer N ,which represents the amount of data in the series. On the next line there are N numbers which represents the value of each number in the series.
Format Output
Output T line with format “Case #X:”, where X represents the testcase number, then followed by N converted numbers.
Constraints
• 1 ≤ T ≤ 20
• 1 ≤ N ≤ 1000
• −10^18 ≤ Ai ≤ 10^18
Sample Input (standard input)
3
5
1 999 888 100 400
8
5 5 1 6 1 6 1 1
5
-30 -30 30 0 -10000
Sample Output (standard output)
Case #1: 1 5 4 2 3
Case #2: 2 2 1 3 1 3 1 1
Case #3: 2 2 4 3 1
My code in C:
#include<stdio.h>
void bubblesort(int array[1000],int n){
for(int i=1;i<n;i++){
for(int j=n-1;j>=i;j--){
if(array[j-1]>array[j]){
int temp = array[j];
array[j] = array[j-1];
array[j-1] = temp;
}
}
}
}
binarysearch(int array[100],int n, int key, int low, int high){
int mid;
low=0,high=n-1;
while(low<=high){
if(array[mid]>key){
high=mid-1;
}
else if(array[mid]<key){
low=mid+1;
}
else if(array[mid]==key){
return mid;
}
}
if(low>high){
return -1;
}
}
int main(){
int tc,array[1000],low,high;
int key;
scanf("%d",&tc);
for(int i=1;i<=tc;i++){
int n;
scanf("%d",&n);
for(int j=0;j<n;j++){
scanf("%d",&array[j]);
}
bubblesort(array,n);
int location=binarysearch(array,n,key,low,high);
printf("Case #%d: ",i);
for(int k=0;k<n;k++){
printf("%d ",location+1);
}
}
return 0;
}

Step by step
Solved in 3 steps with 1 images

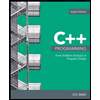
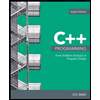