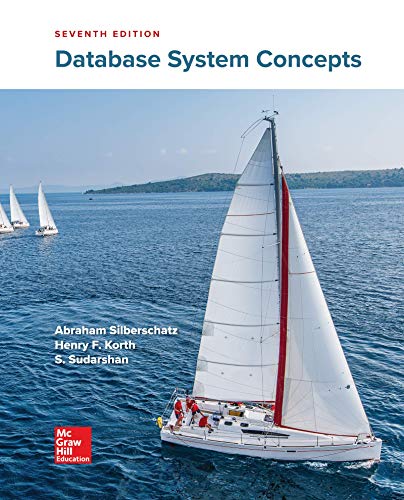
Concept explainers
Below is a recursive version of binary search:
int binarySearch(int nums[], int low, int high, int target)
{
if (low > high)
return -1;
int mid = (low + high)/2;
if (target == nums[mid])
return mid;
else if (target < nums[mid])
return binarySearch(nums, low, mid - 1, target);
else
return binarySearch(nums, mid + 1, high, target);
}
For the 4 statements below, indicate whether you think it is True or False. If you like, you
can provide a description of your answer for partial credit in case you are incorrect.
1) The code “if (low > high) return -1;” is a base case for this function
2) The code “int mid = (low + high)/2;” is a base case for this function
3) The code “if (target == nums[mid]) return mid;” is a base case for this function
4) The code “else return binarySearch(nums, mid + 1, high, target);” is a base case for
this function

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- What will the final values of count1 and count2 be in terms of n? assume that n is a power of 2 of the form 2^r.arrow_forwardExercise 1 Given the following recursive version of selection sort:public void recursiveSelectionSort(int a[], int n, int index){if (index == n)return;int k = minIndex(a, index, n-1);if (k != index)swap(a, k, index);recursiveSelectionSort(a, n, index + 1);}minIndex is a separate function that finds the smallest value in the given array “a” from“index” to “n-1” index values.swap is a separate function that swaps elements in the given array “a” between theelements at index “k” and “index” respectively.Assuming these 2 functions work as expected, there may be an error with therecursiveSelectionSort code. Answer the following:1) Design your own set of 8 unsorted integers in an array2) Determine what the given code will produce with your array by describing what thearray looks like with each recursive instance of recursiveSelectionSort3) If the code does have an error, describe the error, where it is, and how you would fixitFor example, if you said your array was [4 2 3 1 5 6 7 8], then…arrow_forwardComplete the combinations function to generate all combinations of the characters in the string s with length k recursivelyarrow_forward
- Find error #include<bits/stdc++.h> using namespace std; class Solution{ public: bool isPossible(vector<int>ank,int n,int mid) { int count=0; for(int i=0;i<rank.size()) { int val= (-1 + sqrt(1+(8*mid)/rank[i]))/2; count+=val; } return count>=n; } int findMinTime(int N, vector<int>&A, int L){ int low=*min_element(A.end()),high=1000000; int ans=high; while(low<=high) { int mid=low+(high)/2; if(isPossible(A,mid)) { ans=mid; high=mid; } else low=mid; } return ans; } }; int main() { int t; cin>>t; while(t--) { int l; cin >> l; vector<int>arr(l); for(int i = 0; i < l; i++){ cin >> arr[i]; } Solution ob; int ans = ob.findMin(n, *arr, l); cout…arrow_forwardconvert the recursion code to alterative code in java public int array11(int[] nums, int index) { if (index == nums.length) return 0; if (nums[index] == 11) return 1 + array11(nums, index + 1); return array11(nums, index + 1); }arrow_forwardpublic class ArraySection { static void arraySection(int a[], int b[]) { int k = 0; int [] c = new int[a.length]; for(int i = 0; i < a.length; i++) { for(int j = 0; j < b.length; j++) if(a[i] == b[j]) c[k++] = a[i]; } for(int i = 0; i < k; i++) System.out.println(c[i]); System.out.println(); } public static void main(String[] args) { int a[] = { 1, 2, 3, 4, 5 }; int b[] = { 0, 2, 4,5 }; arraySection(a,b); }} Calculate the algorithm step number and algorithm time complexity of the above program?arrow_forward
- void func(vector<string>& names){ sort(names.begin(), names.end()); //(a) for(int i = names.size() - 1; i > 0; --i){. //(b) if(names[i] == names[i - 1]){ names[i] = move(names.back()); names.pop_back(); } } } What are the reasons or consequences for the programmer’s choice to loop backwards in the line marked (b), and under what circumstances would the algorithm behave differently if that line were replaced with: for (int i = 1; i < names.size(); ++i) ? What would change?arrow_forwardIN JAVA Write recursive code and iterative code for binary search.arrow_forwardint sum, k, i, j; int x[4] [4]={1,2,3,4}, {5,6,7,8},{9,8,7,3},{2, 1,7,1}; sum=x[0] [0]; for (k=1; k<=3;k++) sum+=x[k] [k]; Give the value in sum after the statements are executed:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
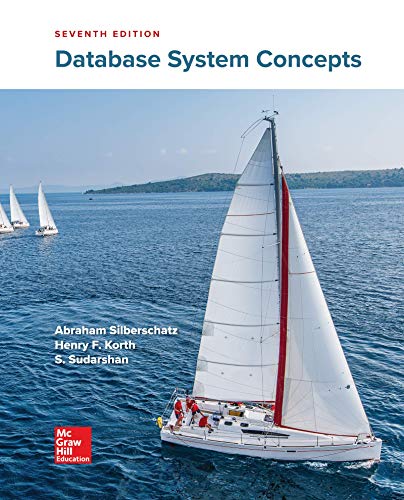
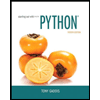
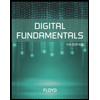
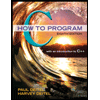
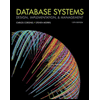
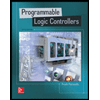