Number guessing Game Write a C program that implements the “guess my number” game. The computer chooses a random number using the following random generator function srand(time(NULL)); int r = rand() % 100 + 1; that creates a random number between 1 and 100 and puts it in the variable r. (Note that you have to include ) Then it asks the user to make a guess. Each time the user makes a guess, the program tells the user if the entered number is larger or smaller than its number. The user then keeps guessing till he/she finds the number. If the user doesn’t find the number after 10 guesses, a proper game over message will be shown and the actual guess is revealed. If the user makes a correct guess in its allowed 10 guesses, then a proper message will be shown and the number of guesses the user made to get the correct answer is also printed. After each correct guess or game over, the user decides to play again or quit and based on the user choice, the computer will make another guess and goes on or prints a proper goodbye message and quits A sample run is as follows (user inputs are in red): Welcome to the Number Guess Game! I choose a number between 1 and 100 and you have only 10 chanced to guess it! OK, I made my mind! What is your guess? > 5 My number is larger than 5! 9 guesses left. What is your guess? > 75 My number is smaller than 75! 8 guesses left. What is your guess? > 40 My number is smaller than 40! 7 guesses left. What is your guess? > 23 My number is larger than 23! 6 guesses left. What is your guess? > 30 My number is larger than 30! 5 guesses left. What is your guess? > 35 You did it! My number is 35! You found it with just 6 guesses. Do you want to play again? (Y/N) > Y OK, I made my mind! What is your guess? > 15 My number is larger than 15! 9 guesses left. … And if the user makes 10 guesses without a success … What is your guess? > 405 Please enter a number between 1 and 100 What is your guess? > 45 My number is larger than 45! 1 guess left. What is your guess? > 47 SORRY! You couldn’t find it with 10 guesses! My number was 46. Maybe next time! Do you want to play again? (Y/N) > N Thanks for playing! See you later.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
-
Number guessing Game
Write a C program that implements the “guess my number” game. The computer chooses a random number using the following random generator function
srand(time(NULL));
int r = rand() % 100 + 1;
that creates a random number between 1 and 100 and puts it in the variable r. (Note that you have to include <time.h>) Then it asks the user to make a guess. Each time the user makes a guess, the program tells the user if the entered number is larger or smaller than its number. The user then keeps guessing till he/she finds the number.
If the user doesn’t find the number after 10 guesses, a proper game over message will be shown and the actual guess is revealed. If the user makes a correct guess in its allowed 10 guesses, then a proper message will be shown and the number of guesses the user made to get the correct answer is also printed.
After each correct guess or game over, the user decides to play again or quit and based on the user choice, the computer will make another guess and goes on or prints a proper goodbye message and quits A sample run is as follows (user inputs are in red):
Welcome to the Number Guess Game!
I choose a number between 1 and 100 and you have only 10 chanced to guess it!
OK, I made my mind!
What is your guess? > 5
My number is larger than 5!
9 guesses left.
What is your guess? > 75
My number is smaller than 75!
8 guesses left.
What is your guess? > 40
My number is smaller than 40!
7 guesses left.
What is your guess? > 23
My number is larger than 23!
6 guesses left.
What is your guess? > 30
My number is larger than 30!
5 guesses left.
What is your guess? > 35
You did it! My number is 35!
You found it with just 6 guesses.
Do you want to play again? (Y/N) > Y
OK, I made my mind!
What is your guess? > 15
My number is larger than 15!
9 guesses left.
…
And if the user makes 10 guesses without a success
…
What is your guess? > 405
Please enter a number between 1 and 100
What is your guess? > 45
My number is larger than 45!
1 guess left.
What is your guess? > 47
SORRY! You couldn’t find it with 10 guesses!
My number was 46. Maybe next time!
Do you want to play again? (Y/N) > N
Thanks for playing! See you later.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

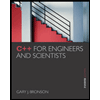
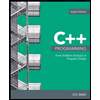
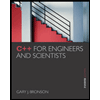
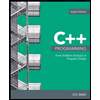