Objective Create a base class named Circle with data members and functions related to circles and a derived class named Cylinder with data members and functions related to cylinders. There are no extra .h or .cpp needed for this assignment. Create a program named LastnameFirstname21.cpp, that does the following: Note: There is no user input for this program. Define a symbolic constant named PI with the value 3.1415926. Use this symbolic constant when dealing with calculations involving PI. Define a base class named Circle for storing the radius and calculating the area of a circle. Circle class contains the following: 1 protected double data member for the radius. 1 default constructor that creates a circle with radius 1. 1 overloaded constructor with a parameter that creates a circle with a given radius. 1 accessor function named getRadius for the radius. 1 mutator function named setRadius for the radius. 1 member function named area that calculates and returns the area of the circle. area = πr2 Any member functions that do NOT modify the radius data member use the const keyword to ensure those functions do not accidentally modify the data member. Any member functions that do modify the radius data member implements the following error correction: If the radius will be 0, make it 1 instead. If the radius will be negative, make it positive instead. Define a derived class named Cylinder (which inherits all data members and member functions of class Circle) for storing the radius (in base class Circle) and height and calculating the area (surface area) and volume of a cylinder. Cylinder class contains the following: 1 private double data member for the height. 1 constructor that creates a Cylinder given a radius and height and uses the inherited Circle constructor to initialize the radius data member. 1 accessor function named getHeight for the height. 1 mutator function named setHeight for the height. 1 member function named area that calculates and returns the surface area of the cylinder. surface area of a cylinder = 2πrh + 2πr2 This member function will override the inherited member function from the Circle class. 1 member function named volume that calculates and returns the volume of the cylinder volume of a cylinder = πr2h Any member functions that do NOT modify the height data member use the const keyword to ensure those functions do not accidentally modify the data member. Any member functions that do modify the height data member implements the following error correction: If the height will be 0, make it 1 instead. If the height will be negative, make it positive instead. Use my main() to test your classes to get the same output shown below: https://laulima.hawaii.edu/x/ndIl9Y There should be no issues compiling or running with the provided main. Be sure to have a program description at the top and in-line comments. Ensure each member function has a description above as well as in-line comments. example output attached
Objective Create a base class named Circle with data members and functions related to circles and a derived class named Cylinder with data members and functions related to cylinders. There are no extra .h or .cpp needed for this assignment. Create a program named LastnameFirstname21.cpp, that does the following: Note: There is no user input for this program. Define a symbolic constant named PI with the value 3.1415926. Use this symbolic constant when dealing with calculations involving PI. Define a base class named Circle for storing the radius and calculating the area of a circle. Circle class contains the following: 1 protected double data member for the radius. 1 default constructor that creates a circle with radius 1. 1 overloaded constructor with a parameter that creates a circle with a given radius. 1 accessor function named getRadius for the radius. 1 mutator function named setRadius for the radius. 1 member function named area that calculates and returns the area of the circle. area = πr2 Any member functions that do NOT modify the radius data member use the const keyword to ensure those functions do not accidentally modify the data member. Any member functions that do modify the radius data member implements the following error correction: If the radius will be 0, make it 1 instead. If the radius will be negative, make it positive instead. Define a derived class named Cylinder (which inherits all data members and member functions of class Circle) for storing the radius (in base class Circle) and height and calculating the area (surface area) and volume of a cylinder. Cylinder class contains the following: 1 private double data member for the height. 1 constructor that creates a Cylinder given a radius and height and uses the inherited Circle constructor to initialize the radius data member. 1 accessor function named getHeight for the height. 1 mutator function named setHeight for the height. 1 member function named area that calculates and returns the surface area of the cylinder. surface area of a cylinder = 2πrh + 2πr2 This member function will override the inherited member function from the Circle class. 1 member function named volume that calculates and returns the volume of the cylinder volume of a cylinder = πr2h Any member functions that do NOT modify the height data member use the const keyword to ensure those functions do not accidentally modify the data member. Any member functions that do modify the height data member implements the following error correction: If the height will be 0, make it 1 instead. If the height will be negative, make it positive instead. Use my main() to test your classes to get the same output shown below: https://laulima.hawaii.edu/x/ndIl9Y There should be no issues compiling or running with the provided main. Be sure to have a program description at the top and in-line comments. Ensure each member function has a description above as well as in-line comments. example output attached
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- Objective
- Create a base class named Circle with data members and functions related to circles and a derived class named Cylinder with data members and functions related to cylinders.
-
- There are no extra .h or .cpp needed for this assignment.
- Create a program named LastnameFirstname21.cpp, that does the following:
- Note: There is no user input for this program.
- Define a symbolic constant named PI with the value 3.1415926. Use this symbolic constant when dealing with calculations involving PI.
- Define a base class named Circle for storing the radius and calculating the area of a circle.
- Circle class contains the following:
- 1 protected double data member for the radius.
- 1 default constructor that creates a circle with radius 1.
- 1 overloaded constructor with a parameter that creates a circle with a given radius.
- 1 accessor function named getRadius for the radius.
- 1 mutator function named setRadius for the radius.
- 1 member function named area that calculates and returns the area of the circle.
- area = πr2
- Any member functions that do NOT modify the radius data member use the const keyword to ensure those functions do not accidentally modify the data member.
- Any member functions that do modify the radius data member implements the following error correction:
- If the radius will be 0, make it 1 instead.
- If the radius will be negative, make it positive instead.
- Define a derived class named Cylinder (which inherits all data members and member functions of class Circle) for storing the radius (in base class Circle) and height and calculating the area (surface area) and volume of a cylinder.
- Cylinder class contains the following:
- 1 private double data member for the height.
- 1 constructor that creates a Cylinder given a radius and height and uses the inherited Circle constructor to initialize the radius data member.
- 1 accessor function named getHeight for the height.
- 1 mutator function named setHeight for the height.
- 1 member function named area that calculates and returns the surface area of the cylinder.
- surface area of a cylinder = 2πrh + 2πr2
- This member function will override the inherited member function from the Circle class.
- 1 member function named volume that calculates and returns the volume of the cylinder
- volume of a cylinder = πr2h
- Any member functions that do NOT modify the height data member use the const keyword to ensure those functions do not accidentally modify the data member.
- Any member functions that do modify the height data member implements the following error correction:
- If the height will be 0, make it 1 instead.
- If the height will be negative, make it positive instead.
- Use my main() to test your classes to get the same output shown below: https://laulima.hawaii.edu/x/ndIl9Y
- There should be no issues compiling or running with the provided main.
- Be sure to have a program description at the top and in-line comments.
- Ensure each member function has a description above as well as in-line comments.
example output attached
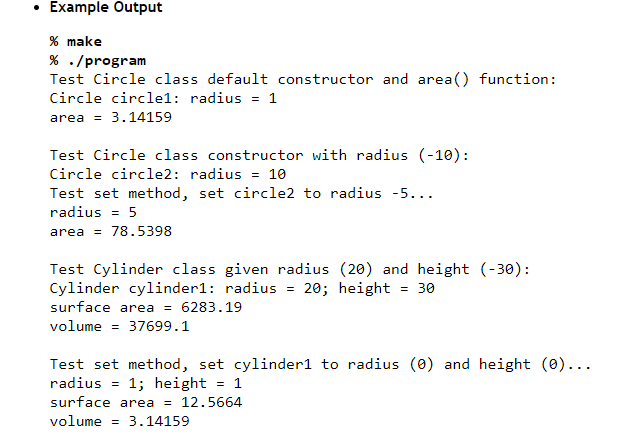
Transcribed Image Text:Example Output
% make
% ./program
Test Circle class default constructor and area() function:
Circle circle1: radius = 1
area 3.14159
Test Circle class constructor with radius (-10):
Circle circle2: radius = 10
Test set method, set circle2 to radius -5...
radius = 5
area = 78.5398
Test Cylinder class given radius (20) and height (-30):
Cylinder cylinder1: radius = 20; height
= 30
surface area = 6283.19
volume = 37699.1
Test set method, set cylinder1 to radius (0) and height (0)...
radius= 1; height
= 1
surface area = 12.5664
volume = 3.14159
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
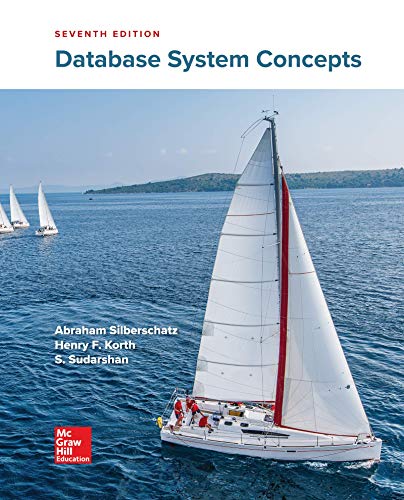
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
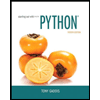
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
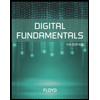
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
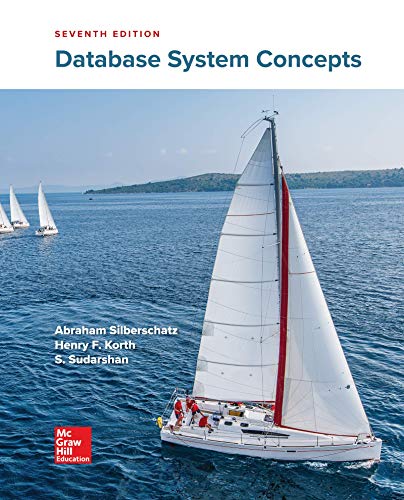
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
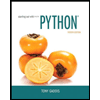
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
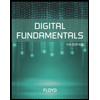
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
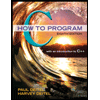
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
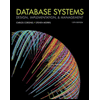
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
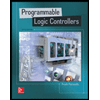
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education