Objectives By completing this lab, studonts should be able to. • Write programs using conditional operatorS • Write programs using logical expressions • Write programs using getline () Background Relational operators allow you to compare numerie, charactor, and string valucs to determine whether ome is greater than, less than, equal to, or not equal to another. A relatiomal expressioın evaluates one or more variables using relational operators to arrive at a Boolean (true or falve) outeome. Consider the fallowing relational operators and expresion: Relational Operator Relational Expression Meaning x grester hun y bx les than y Iax grester thun or oual a y lax les than or equal to y! Isx oqual to y Isx not equai to y Control structures are commands used in C++ to control the hehavior of a program based on certain eriteria, such as user input. In this luh, you are heing intraduced to the "i" statement. There ure three different uses ofthe ": f" statement in C- if, it/alne, und iflelso-if/alse. In their basic form, the following skeleton code templates implement these statements: if (re'ational axprassiori /* Execate Laia code il the rela.ional expresaion la tre */ if frelationsl expreasian) /* Execute code here it the expression is true / * Execule cude heze 1E Lhe expreusion iu faise */ If (selational expreasion 11 /A Exacuta oode rare if axpresaton s true / else if trelational expression 2) /* Execute code here if expzession 2 is true / else /* Execute code here if ALL expressions are false /
Objectives By completing this lab, studonts should be able to. • Write programs using conditional operatorS • Write programs using logical expressions • Write programs using getline () Background Relational operators allow you to compare numerie, charactor, and string valucs to determine whether ome is greater than, less than, equal to, or not equal to another. A relatiomal expressioın evaluates one or more variables using relational operators to arrive at a Boolean (true or falve) outeome. Consider the fallowing relational operators and expresion: Relational Operator Relational Expression Meaning x grester hun y bx les than y Iax grester thun or oual a y lax les than or equal to y! Isx oqual to y Isx not equai to y Control structures are commands used in C++ to control the hehavior of a program based on certain eriteria, such as user input. In this luh, you are heing intraduced to the "i" statement. There ure three different uses ofthe ": f" statement in C- if, it/alne, und iflelso-if/alse. In their basic form, the following skeleton code templates implement these statements: if (re'ational axprassiori /* Execate Laia code il the rela.ional expresaion la tre */ if frelationsl expreasian) /* Execute code here it the expression is true / * Execule cude heze 1E Lhe expreusion iu faise */ If (selational expreasion 11 /A Exacuta oode rare if axpresaton s true / else if trelational expression 2) /* Execute code here if expzession 2 is true / else /* Execute code here if ALL expressions are false /
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please provide a code that runs in visual studio with the output.
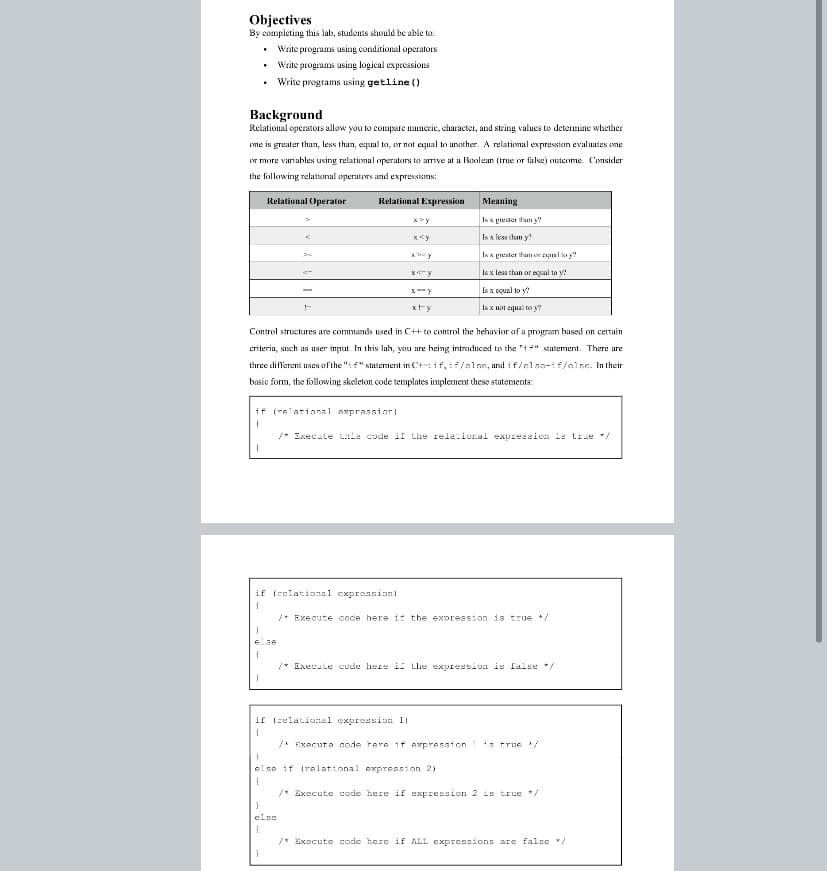
Transcribed Image Text:Objectives
By completing this lab, students should be able to.
Write programs using conditional operators
Write programs using logical expressions
Write programs using getline ()
Background
Relational operators allow you to compare numerie, character, and string values to determine whether
me is greater than, less than, equal ta, or nat equal to another. A relational expressian evaluates one
or more variables using relational operatars to arrive at a Bšoolean (true or false) outcome. Consider
the following relatiomal operators and expressions:
Relational Operator
Relational Expression
Meaning
Is x grester thun y
Is x less than y?
Is x grester thum or l ay
Is x les than or equal to y!
Is x oqual to y?
x!-y
Is x not equal to y
Control structures are commands used in C++ to control the behavior of a program based on certain
criteria, such as user input. In this lah, you are being intraduced to the 'if" statement. There are
three different uses ofthe "i f" statement in C+: if, if/alse, and i f/else-if/else. In their
basic form, the following skeleton code templates implement these statements:
if (relatianal axprassior!
* Execate tale code il the rela ion.al expreesicn le true */
if (rclational expression)
* Execute code here if the expreasion is true /
else
/* Execule cude here if Lhe expression is false */
if (relational expreasion 11
A Fxecuta code tere if expression 1is true /
else if (relational expression 2)
/* Execute code here if expression 2 is true */
clse
/* Execute code here if ALL expressions are false /
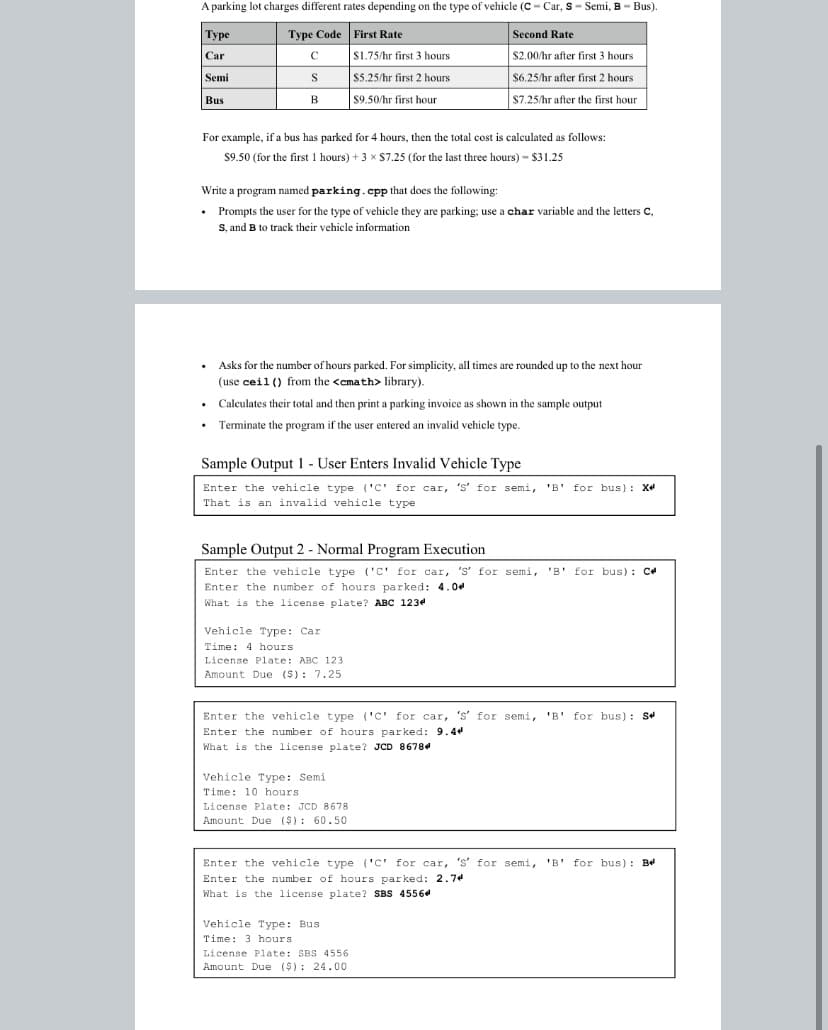
Transcribed Image Text:A parking lot charges different rates depending on the type of vehicle (C - Car, S- Semi, B - Bus).
|Туре
Type Code First Rate
Second Rate
Car
S1.75/hr first 3 hours
$2.00/hr after first 3 hours
S5.25/hr first 2 hours
$6.25/hr after first 2 hours
Semi
Bus
B
$9.50/hr first hour
$7.25/hr after the first hour
For example, if a bus has parked for 4 hours, then the total cost is calculated as follows:
S9.50 (for the first 1 hours) + 3 x S7.25 (for the last three hours) - $31.25
Write a program named parking.cpp that does the following:
Prompts the user for the type of vehicle they are parking; use a char variable and the letters C,
S, and B to track their vehicle information
Asks for the number of hours parked. For simplicity, all times are rounded up to the next hour
(use ceil () from the <emath> library).
Calculates their total and then print a parking invoice as shown in the sample output
Terminate the program if the user entered an invalid vehicle type.
Sample Output 1 - User Enters Invalid Vehicle Type
Enter the vehicle type ('C' for car, 's' for semi, 'B' for bus) : X4
That is an invalid vehicle type
Sample Output 2 - Normal Program Execution
Enter the vehicle type ('C' for car, 's' for semi, 'B' for bus): Ce
Enter the number of hours parked: 4.04
What is the license plate? ABC 123e
Vehicle Type: Car
Time: 4 hours
License Plate: ABC 123
Amount Due ($): 7.25
Enter the vehicle type ('C' for car, 's' for semi, 'B' for bus): S*
Enter the number of hours parked: 9.44
What is the license plate? JCD 86784
Vehicle Type: Semi
Time: 10 hours
License Plate: JCD 8678
Amount Due ($): 60.50
Enter the vehicle type ('C' for car, 's' for semi, 'B' for bus): Be
Enter the number of hours parked: 2.74
What is the license plate? SBS 45564
Vehicle Type: Bus
Time: 3 hours
License Plate: SBS 4556
Amount Due ($): 24.00
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 5 images

Recommended textbooks for you
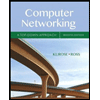
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
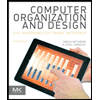
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
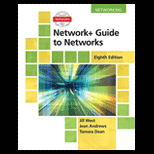
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
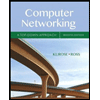
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
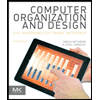
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
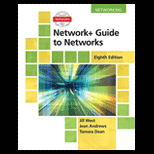
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
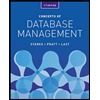
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
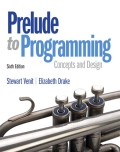
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
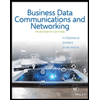
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY