hello, I have errors in my code, and i'm not sure on how fix my some of my myethods. I have the outline for my code bellow, as well as the rubric: description: Write a java application that will open an input file and open an output file. The file names can be entered fromthe command line, if a command line parameter is included, it will be used as the input file name. If a second command line parameter is included, it will be used as the output file name. Any missing parameters will be prompted for and read by the program. When the input file does not exist the user will be prompted to re-enter an input file name. When nothing is entered for the input file name, on a prompt, the program will proceed to the end of the program and terminate. When the output file exists the user will havea choice of entering a new output file name, backing up the existing output file first, overwriting the existingoutput file, or quitting the program without opening any files. Again, not entering a file name will alsoterminate the program. The program will catch any file open error and print a message. Using StringTokenizer, the program will parse the input file and identify words and integers. Words are notcase sensitive and can only contain letters, apostrophes, hyphens, and digits but must start with a letter. The numbers are a sequence of digits. They can be positive or negative integers. If the number is negative, there is no space between the negative sign, -, and the first digit. A sum will be made form the integers contained in the input file.
hello, I have errors in my code, and i'm not sure on how fix my some of my myethods. I have the outline for my code bellow, as well as the rubric:
description:
Write a java application that will open an input file and open an output file. The file names can be entered fromthe command line, if a command line parameter is included, it will be used as the input file name. If a second command line parameter is included, it will be used as the output file name. Any missing parameters will be
prompted for and read by the program. When the input file does not exist the user will be prompted to re-enter
an input file name. When nothing is entered for the input file name, on a prompt, the program will proceed to
the end of the program and terminate. When the output file exists the user will havea choice of entering a new output file name, backing up the existing output file first, overwriting the existingoutput file, or quitting the program without opening any files. Again, not entering a file name will alsoterminate the program. The program will catch any file open error and print a message.
Using StringTokenizer, the program will parse the input file and identify words and integers. Words are notcase sensitive and can only contain letters, apostrophes, hyphens, and digits but must start with a letter. The
numbers are a sequence of digits. They can be positive or negative integers. If the number is negative, there is
no space between the negative sign, -, and the first digit. A sum will be made form the integers contained in the
input file.
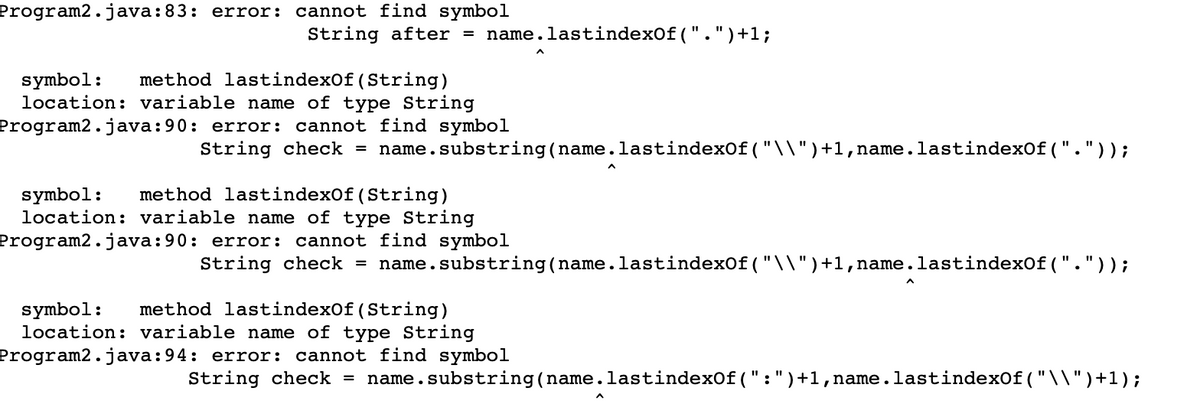
![Program2.java:36: error: non-static method openout(String) cannot be referenced from a static context
outfile=IOFile.openout(ionames[1]);
Program2.java:66: error: cannot find symbol
dummy.exists();
exists =
variable exists
symbol:
location: class IOFile
Program2.java:67: error: cannot find symbol
return exists;
symbol:
location: class IOFile
variable exists
Program2.java:72: error: cannot find symbol
BKP= name.substring(0, name.lastindexOf ("."))+"." +ext;
method lastindexOf(String)
symbol:
location: variable name of type String](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcbc5c5ff-3f02-45f2-bc7a-efd5c3d6b891%2F538132a5-4f2d-43df-a85a-82b98476d155%2F69ygevl_processed.png&w=3840&q=75)

package bartleby;
import java.io.*;
import java.nio.file.Files;
import java.util.StringTokenizer;
public class Program2 {
public static void main(String[] args) throws IOException {
IOFile myFilesObj = new IOFile();
int i = 0; // Array index
int count = 0; // number of Word objects in words array
int sum = 0; // sum of integers
// Word[] words = new Word[100]; // Array of word objects
StringTokenizer inline; // Stringtokenizer of current line
BufferedReader infile; // input file handle
PrintWriter outfile; // output file handle
myFilesObj.validateNames(args);
infile = myFilesObj.openIn(myFilesObj.inputFileName);
outfile = myFilesObj.openOut(myFilesObj.outputFileName);
}
}
class IOFile {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String inputFileName, outputFileName;
void validateNames(String[] args) {
switch (args.length) {
case 0:
getInputFileName();
getOutputFileName();
break;
case 1:
getInputFileName(args[0]);
getOutputFileName();
break;
case 2:
getInputFileName(args[0]);
getOutputFileName(args[1]);
break;
}
}
private void getOutputFileName() {
try {
System.out.print("enter output file : ");
outputFileName = br.readLine();
if (outputFileName.equals(""))
System.exit(0);
if (!fileExists(outputFileName)) {
createFile(outputFileName);
} else {
backup(outputFileName, getBackupFileName(outputFileName));
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void getOutputFileName(String outputFileName) {
this.outputFileName = outputFileName;
if (!fileExists(outputFileName)) {
createFile(outputFileName);
} else {
backup(outputFileName, getBackupFileName(outputFileName));
}
}
private void createFile(String outputFileName) {
File fileObj = new File(outputFileName);
try {
fileObj.createNewFile();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private String getBackupFileName(String outputFileName) {
return outputFileName.substring(0, outputFileName.lastIndexOf('.')) + "-backup"
+ outputFileName.substring(outputFileName.lastIndexOf('.'));
}
private void getInputFileName(String inputFileName) {
this.inputFileName = inputFileName;
if (!fileExists(inputFileName)) {
System.out.println("File " + inputFileName + " does not exist.");
getInputFileName();
}
}
private void getInputFileName() {
try {
System.out.print("enter input file : ");
inputFileName = br.readLine();
if (inputFileName.equals(""))
System.exit(0);
if (!fileExists(inputFileName)) {
System.out.println("File " + inputFileName + " does not exist.");
getInputFileName();
}
} catch (IOException e) {
e.printStackTrace();
}
}
boolean fileExists(String name) {
File dummy = new File(name);
return dummy.exists();
}
void backup(String fileName, String backupFileName) {
fileBackup(fileName, backupFileName);
System.out.println("Backup File created: " + new File(fileName).getName());
}
void fileBackup(String fileName, String backupName) {
try {
Files.copy(new File(fileName).toPath(), new File(backupName).toPath());
} catch (IOException e) {
e.printStackTrace();
}
}
BufferedReader openIn(String name) {
BufferedReader in = null;
try {
in = new BufferedReader(new FileReader(name));
} catch (FileNotFoundException e) {
System.out.println("error, file not found");
}
return in;
}
PrintWriter openOut(String name) {
PrintWriter out = null;
try {
out = new PrintWriter(new FileWriter(name));
} catch (IOException e) {
System.out.println("error, file not found");
}
return out;
}
}
//
//class Word {
// Word(String word) {
// }
//
// int getCount() {
// }
//
// String getWord() {
// }
//
// Boolean isWord(String word) {
// }
//
// Boolean isWordIgnoreCase(String word) {
// }
//
// Void addOne() {
// }
//
// Void print(PrintWriter out) {
// }
//
// int FindWord(Word[] list, String Word, int n) {
// }
//}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

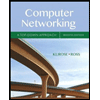
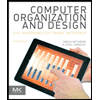
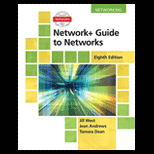
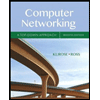
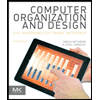
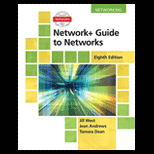
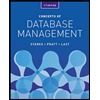
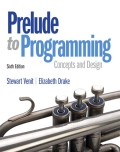
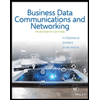