Objectives: Construct and use 1D and 2D arrays Send arrays to methods Iterate through an array to perform a calculation Details: This assignment will be completed using the Eclipse IDE. Attach your .java file to the Assignment page. Which of the following five people is the most interesting famous computer scientist? Grace Hopper Ada Lovelace Katherine Johnson Frances Allen Shafi Goldwasser Let’s say that we ask a group of 20 people to vote for which one of the computer scientists is most interesting. Each voter votes for one candidate and, of course, we are interested in who wins. Our data is a matrix with each row representing one of the five computer scientists and each column representing one of the 20 voters. The data in the matrix consists of 1s and 0s. The number 1 in each column represents a vote for the candidate represented by that row. Here is an example matrix (the data matrix is just the numbers). Next to each row is the name of the computer scientist represented by that row. 10001001000000000000 Grace Hopper 00010000100111000010 Ada Lovelace 00100100001000000000 Katherine Johnson 01000010000000111101 Francis Allen 00000000010000000000 Shafi Goldwasser The way we read this is that the first voter (the first column) voted for Grace Hopper (the first row) because a 1 appears in that row x column combination. The rest of the numbers in that first column are zeros. The second voter voted for Francis Allen. The third voter voted for Katherine Johnson, and so on. Every column should have a single 1 and the rest 0s. By counting across the columns, we can see how many votes each candidate received from the 20 voters. For example, in this case Grace Hopper received three votes, Ada Lovelace received six votes, and so on. In your assignment, you will examine data from three voter groups like the one above. The ultimate goal is to determine who wins in each group. Here are the two sets of data you will use: group1: 10001001000000000000 00010000100111000010 00100100001000000000 01000010000000111101 00000000010000000000 group2: 00100000000110000000 00010000010001110001 11001000100000000010 00000110000000000100 00000001001000001000 For each of the two groups, your code will: Count the number of votes for each candidate and print them out. Determine who the winner is and print out their name. Finally, just to show off, print the raw data in the row x column format as shown above, and then print the transpose of the matrix in column x row format. Your code will be “verbose” about what it is doing – printing out progress as it is processing the data. Here is the code that you should put in the main method in order to produce the output above: System.out.println("Voting Group 1"); winner = winnerIndex(group1,name); System.out.println("The winner is " + name[winner]); System.out.println("Raw data row x column: "); printAllData(group1); System.out.println("Raw data column x row: "); printTransposeData(group1); System.out.println("\nVoting Group 2"); winner = winnerIndex(group2,name); System.out.println("The winner is " + name[winner]); System.out.println("Raw data row x column: "); printAllData(group2); System.out.println("Raw data column x row: "); printTransposeData(group2); In order to get this to work, you will need to do the following: In the main method, create an array to hold the names of the five computer scientists. Refer to it by a variable called name. Assign each computer scientist’s name to successive elements of the name array. (Hint: Remember that you can declare an array and populate it with values in a single statement.) In the main method, create arrays to hold the values of the two voting matrices. Refer to them by variable names group1 and group2. Assign the values from the two voting matrices to the appropriate elements in the group1 and group2 arrays. (Hint: Remember that you can declare an array and populate it with values in a single statement.) Write a method called winnerIndex that tallies the votes for each candidate, determines who has the most votes, and returns the index of the winner. As you can see from the main method code, winnerIndex should accept references to a vote array and to the name array as arguments. winnerIndex should: Count the votes for each candidate row. For each row, print “Votes for” followed by the name of the candidate being tallied (using the name array), followed by “=”, followed by the sum of votes for that candidate. Keep track of which candidate has the most votes and return the array index value for that candidate. Write a method called printAllData that simply prints the vote array in row x column format. Write a method called printTransposeData that prints the transpose of the matrix, column x row format.
Objectives:
- Construct and use 1D and 2D arrays
- Send arrays to methods
- Iterate through an array to perform a calculation
Details:
This assignment will be completed using the Eclipse IDE. Attach your .java file to the Assignment page.
Which of the following five people is the most interesting famous computer scientist?
- Grace Hopper
- Ada Lovelace
- Katherine Johnson
- Frances Allen
- Shafi Goldwasser
Let’s say that we ask a group of 20 people to vote for which one of the computer scientists is most interesting. Each voter votes for one candidate and, of course, we are interested in who wins.
Our data is a matrix with each row representing one of the five computer scientists and each column representing one of the 20 voters. The data in the matrix consists of 1s and 0s. The number 1 in each column represents a vote for the candidate represented by that row.
Here is an example matrix (the data matrix is just the numbers). Next to each row is the name of the computer scientist represented by that row.
10001001000000000000 Grace Hopper
00010000100111000010 Ada Lovelace
00100100001000000000 Katherine Johnson
01000010000000111101 Francis Allen
00000000010000000000 Shafi Goldwasser
The way we read this is that the first voter (the first column) voted for Grace Hopper (the first row) because a 1 appears in that row x column combination. The rest of the numbers in that first column are zeros. The second voter voted for Francis Allen. The third voter voted for Katherine Johnson, and so on. Every column should have a single 1 and the rest 0s. By counting across the columns, we can see how many votes each candidate received from the 20 voters. For example, in this case Grace Hopper received three votes, Ada Lovelace received six votes, and so on.
In your assignment, you will examine data from three voter groups like the one above. The ultimate goal is to determine who wins in each group.
Here are the two sets of data you will use:
group1:
10001001000000000000
00010000100111000010
00100100001000000000
01000010000000111101
00000000010000000000
group2:
00100000000110000000
00010000010001110001
11001000100000000010
00000110000000000100
00000001001000001000
For each of the two groups, your code will:
- Count the number of votes for each candidate and print them out.
- Determine who the winner is and print out their name.
- Finally, just to show off, print the raw data in the row x column format as shown above, and then print the transpose of the matrix in column x row format.
Your code will be “verbose” about what it is doing – printing out progress as it is processing the data.
Here is the code that you should put in the main method in order to produce the output above:
System.out.println("Voting Group 1");
winner = winnerIndex(group1,name);
System.out.println("The winner is " + name[winner]);
System.out.println("Raw data row x column: ");
printAllData(group1);
System.out.println("Raw data column x row: ");
printTransposeData(group1);
System.out.println("\nVoting Group 2");
winner = winnerIndex(group2,name);
System.out.println("The winner is " + name[winner]);
System.out.println("Raw data row x column: ");
printAllData(group2);
System.out.println("Raw data column x row: ");
printTransposeData(group2);
In order to get this to work, you will need to do the following:
- In the main method, create an array to hold the names of the five computer scientists. Refer to it by a variable called name. Assign each computer scientist’s name to successive elements of the name array. (Hint: Remember that you can declare an array and populate it with values in a single statement.)
- In the main method, create arrays to hold the values of the two voting matrices. Refer to them by variable names group1 and group2. Assign the values from the two voting matrices to the appropriate elements in the group1 and group2 arrays. (Hint: Remember that you can declare an array and populate it with values in a single statement.)
- Write a method called winnerIndex that tallies the votes for each candidate, determines who has the most votes, and returns the index of the winner. As you can see from the main method code, winnerIndex should accept references to a vote array and to the name array as arguments. winnerIndex should:
- Count the votes for each candidate row. For each row, print “Votes for” followed by the name of the candidate being tallied (using the name array), followed by “=”, followed by the sum of votes for that candidate.
- Keep track of which candidate has the most votes and return the array index value for that candidate.
- Write a method called printAllData that simply prints the vote array in row x column format.
- Write a method called printTransposeData that prints the transpose of the matrix, column x row format.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

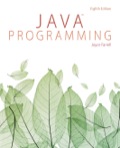
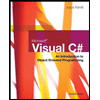
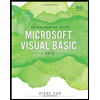
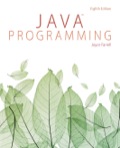
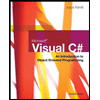
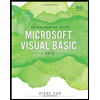