odule to do the translation. 1. Download the morse module to your folder. 2. Create your step_1.py program and import morse --> morse.py Download morse.py 3. Create your menu driven interface using a while loop with the following menu:
Here is step one in my python project
In this step we'll be translating a single letter to Morse Code and a single Morse Code to a letter. The only input validation will be on the menu selection to handle the case where the user has entered something other than 0, 1 or 2. We'll import a module of our own (provided for you) and we'll use the dictionaries in that module to do the translation.
1. Download the morse module to your folder.
2. Create your step_1.py program and import morse --> morse.py Download morse.py
3. Create your menu driven interface using a while loop with the following menu:
Morse Code Translator
0: Exit
1: Translate a word into Morse Code
2: Translate Morse Code to text.
4. Use an if-elif-else construct, with 'else' explaining to the user that the selection was invalid and that only 0, 1 and 2 are acceptable inputs.
5. If the user enters "0", exit the program.
6. If the user enters "1":
- Prompt the user for a letter with the input() command and convert it to upper case with the upper() string method.
- Use that letter as a key in the alpha_to_morse dictionary (from the morse module) to fetch the Morse Code value.
- Display a message to the user showing the Morse Code associated with the letter provided.
7. If the user enters "2":
- Prompt the user for a Morse Code with the input()
- Use the Morse Code as a key to the morse_to_alpha dictionary (morse module) to fetch the corresponding letter value.
- Display a message to the user showing the letter associated with the Morse Code.
Step 2 in my python project
Now that you have the while loop and the dictionary translations working, the next task is to create two functions, translate_to_morse() and translate_to_alpha().
- Make a copy of step_1. py and save it as step_2.py, as a starting point.
- At the top of the program, underneath the header comments, create both functions. translate_to_morse() should have one parameter, a string which consists of a letter. Move the code used in Menu Selection 1 to translate the letter to a Morse Code into the function. Put the resulting Morse Code into the return statement.
- In the same fashion, create the translate_to_alpha() function and move the translation code from the while loop into the function. Note that the parameter for translate_to_alpha() is a Morse Code, not a letter.
- In Menu Selection 1, call the translate_to_morse() function, passing the letter as an argument and storing the return value in a variable. Both the input() and print() statements from Step 1 should remain inside the while loop an should NOT be part of the function. The function's job is to do the translation, not to communicate with the user.
- The changes to Menu Selection 2 are basically the same. Call the translate_to_alpha() function, passing the Morse Code as an argument and storing the return value in a variable. Similarly, the input() and print() statements should not be part of the function.
Note that for testing purposes, you can take the output of Menu Selection 1 and copy-and-paste it as input to Menu Selection 2 and the result should be the results should mirror each other. That is, entering "S" for Menu Selection 1 should result in "..." as a Morse Code. Use this as input for Menu Selection 2 and the result should be "S".
Here's the code I have for step one in the python on project
def morse_code(text):
tranlation_dic = {
"A": ".-",
"B": "-...",
"C": "-.-.",
"D": "-..",
"E": ".",
"F": "..-.",
"G": "--.",
"H": "....",
"I": "..",
"J": ".---",
"K": "-.-",
"L": ".-..",
"M": "--",
"N": "-.",
"O": "---",
"P": ".--.",
"Q": "--.-",
"R": ".-.",
"S": "...",
"T": "-",
"U": "..-",
"V": "...-",
"W": ".--",
"X": "-..-",
"Y": "-.--",
"Z": "--..",
".-": "A",
"-...": "B",
"-.-.": "C",
"-..": "D",
".": "E",
"..-.": "F",
"--.": "G",
"....": "H",
"..": "I",
".---": "J",
"-.-": "K",
".-..": "L",
"--": "M",
"-.": "N",
"---": "O",
".--.": "P",
"--.-": "Q",
".-.": "R",
"...": "S",
"-": "T",
"..-": "U",
"...-": "V",
".--": "W",
"-..-": "X",
"-.--": "Y",
"--..": "Z"
}
return tranlation_dic[text]
menu = """
Morse Code Translator
0: Exit
1: Translate a word into Morse Code
2: Translate Morse Code to text.
"""
done = False
while not done:
print(menu)
selection = input('Please make a selection: ')
if selection == "0":
done = True
elif selection == "1":
morseCode = input('Please enter a word to be translated to Morse Code: ')
morseCode = morseCode.upper()
print("Morse Code for the provided letter is: ", morse_code(morseCode))
elif selection == "2":
morseCode = input('Please enter a word from the Morse Code Tranlator menu: ')
print("Text for the provided Morse Code is: ", morse_code(morseCode))
else:
print("Selection is invalid. Only 0, 1, and 2 are the acceptable inputs.")
Please help me with step 2 on python project.

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

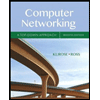
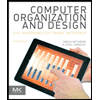
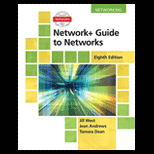
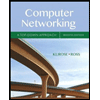
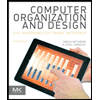
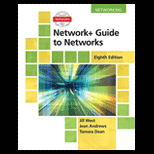
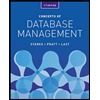
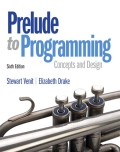
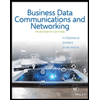