Often times after a big meal, we may find ourselves considering how much we need to exercise to work off what we just ate. In this exercise we will actually compute it. 1) Oxygen consumption (VO2) can be used as an indicator of exercise intensity, which is closely tied to energy expenditure. In other words, VO2 can be used to approximate how many calories are burned during an activity. The approximate VO2 for walking (on flat land) at a given speed (mph) can be computed as: VO, for walking = 2.68224 x speed + 3.5 The approximate VO, for running (on flat land) at a given speed (mph) can be computed as: VO, for running = 5.36448 x speed + 3.5 2) The calorie burn rate (CBR) is the number of calories burned per minute when doing a certain activity. A simplified way to compute the CBR from VO, and a person's weight (Ibs) is given by the formula: CBR = (2.268 x 10) x VO2 x weight %3D You need to write three (3) versions of a program that calculates how many minutes one needs to exercise to burn off a given amount of food they ate. To calculate the number of minutes required to burn off a given number of calories, simply divide the total calories by the calorie burn rate. In the descriptions for PART A, PART B, and PART C, I use "chicken nuggets" as the food of choice. Assume that chicken nugget has 47 calories each. However, in your program you may use whatever type of food you want. Be sure to look up the amount of calories for that food type (you may try: www.calorieking.com). Disclaimer: Note that these calculations are simple approximations and do not account for all the different individual factors for each person. This exercise (pardon the pun) is intended to help students learn how to write computer programs, not to inform their decisions on how to take care of their physical health. Therefore, do not base any real-life health or fitness program (pun intended) on these calculations. PART B. Make a copy of the previous program and rename the class to FitnessPlanner_2. This time, before asking the user to enter a walking or running speed, ask the user if he/she prefers walking or running by asking the user to input "W" for walking or "R" for running. You then should only ask the user to enter the walking speed OR the running speed according to the user's response to this query. Likewise, use the appropriate formula (for walking or for running). When you read the letters w/W or r/R from the user, save them as strings. The method to read a string is next(). Example: Scanner keyboard System.out.println("Enter your gender: "); String gender = new Scanner (System.in); = keyboard.next(); *** This Fitness Planner program will calculate how long *** you need to exercise to burn off what you just ate. *** *** What is your weight in pounds? 150 How many chicken nuggets did you eat? 12 Do you prefer walking or running (W or R)? What is your average WALKING speed in mph (3 is typical, a 20-min/mi pace)? 2 You need to walk for 187 minutes to burn off the 12 chicken nuggets you ate.
Often times after a big meal, we may find ourselves considering how much we need to exercise to work off what we just ate. In this exercise we will actually compute it. 1) Oxygen consumption (VO2) can be used as an indicator of exercise intensity, which is closely tied to energy expenditure. In other words, VO2 can be used to approximate how many calories are burned during an activity. The approximate VO2 for walking (on flat land) at a given speed (mph) can be computed as: VO, for walking = 2.68224 x speed + 3.5 The approximate VO, for running (on flat land) at a given speed (mph) can be computed as: VO, for running = 5.36448 x speed + 3.5 2) The calorie burn rate (CBR) is the number of calories burned per minute when doing a certain activity. A simplified way to compute the CBR from VO, and a person's weight (Ibs) is given by the formula: CBR = (2.268 x 10) x VO2 x weight %3D You need to write three (3) versions of a program that calculates how many minutes one needs to exercise to burn off a given amount of food they ate. To calculate the number of minutes required to burn off a given number of calories, simply divide the total calories by the calorie burn rate. In the descriptions for PART A, PART B, and PART C, I use "chicken nuggets" as the food of choice. Assume that chicken nugget has 47 calories each. However, in your program you may use whatever type of food you want. Be sure to look up the amount of calories for that food type (you may try: www.calorieking.com). Disclaimer: Note that these calculations are simple approximations and do not account for all the different individual factors for each person. This exercise (pardon the pun) is intended to help students learn how to write computer programs, not to inform their decisions on how to take care of their physical health. Therefore, do not base any real-life health or fitness program (pun intended) on these calculations. PART B. Make a copy of the previous program and rename the class to FitnessPlanner_2. This time, before asking the user to enter a walking or running speed, ask the user if he/she prefers walking or running by asking the user to input "W" for walking or "R" for running. You then should only ask the user to enter the walking speed OR the running speed according to the user's response to this query. Likewise, use the appropriate formula (for walking or for running). When you read the letters w/W or r/R from the user, save them as strings. The method to read a string is next(). Example: Scanner keyboard System.out.println("Enter your gender: "); String gender = new Scanner (System.in); = keyboard.next(); *** This Fitness Planner program will calculate how long *** you need to exercise to burn off what you just ate. *** *** What is your weight in pounds? 150 How many chicken nuggets did you eat? 12 Do you prefer walking or running (W or R)? What is your average WALKING speed in mph (3 is typical, a 20-min/mi pace)? 2 You need to walk for 187 minutes to burn off the 12 chicken nuggets you ate.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 19PE
Related questions
Question
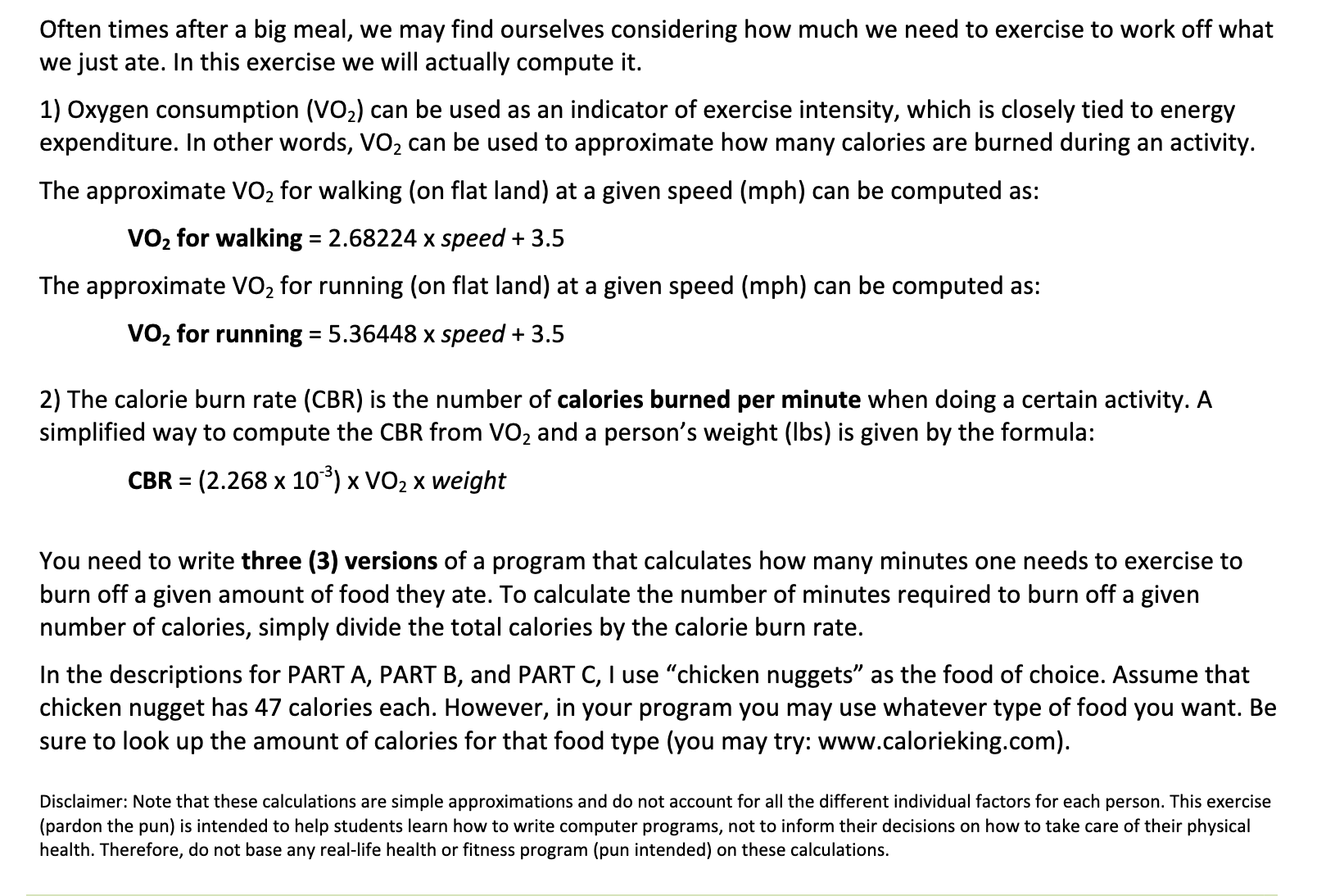
Transcribed Image Text:Often times after a big meal, we may find ourselves considering how much we need to exercise to work off what
we just ate. In this exercise we will actually compute it.
1) Oxygen consumption (VO2) can be used as an indicator of exercise intensity, which is closely tied to energy
expenditure. In other words, VO2 can be used to approximate how many calories are burned during an activity.
The approximate VO2 for walking (on flat land) at a given speed (mph) can be computed as:
VO, for walking = 2.68224 x speed + 3.5
The approximate VO, for running (on flat land) at a given speed (mph) can be computed as:
VO, for running = 5.36448 x speed + 3.5
2) The calorie burn rate (CBR) is the number of calories burned per minute when doing a certain activity. A
simplified way to compute the CBR from VO, and a person's weight (Ibs) is given by the formula:
CBR = (2.268 x 10) x VO2 x weight
%3D
You need to write three (3) versions of a program that calculates how many minutes one needs to exercise to
burn off a given amount of food they ate. To calculate the number of minutes required to burn off a given
number of calories, simply divide the total calories by the calorie burn rate.
In the descriptions for PART A, PART B, and PART C, I use "chicken nuggets" as the food of choice. Assume that
chicken nugget has 47 calories each. However, in your program you may use whatever type of food you want. Be
sure to look up the amount of calories for that food type (you may try: www.calorieking.com).
Disclaimer: Note that these calculations are simple approximations and do not account for all the different individual factors for each person. This exercise
(pardon the pun) is intended to help students learn how to write computer programs, not to inform their decisions on how to take care of their physical
health. Therefore, do not base any real-life health or fitness program (pun intended) on these calculations.
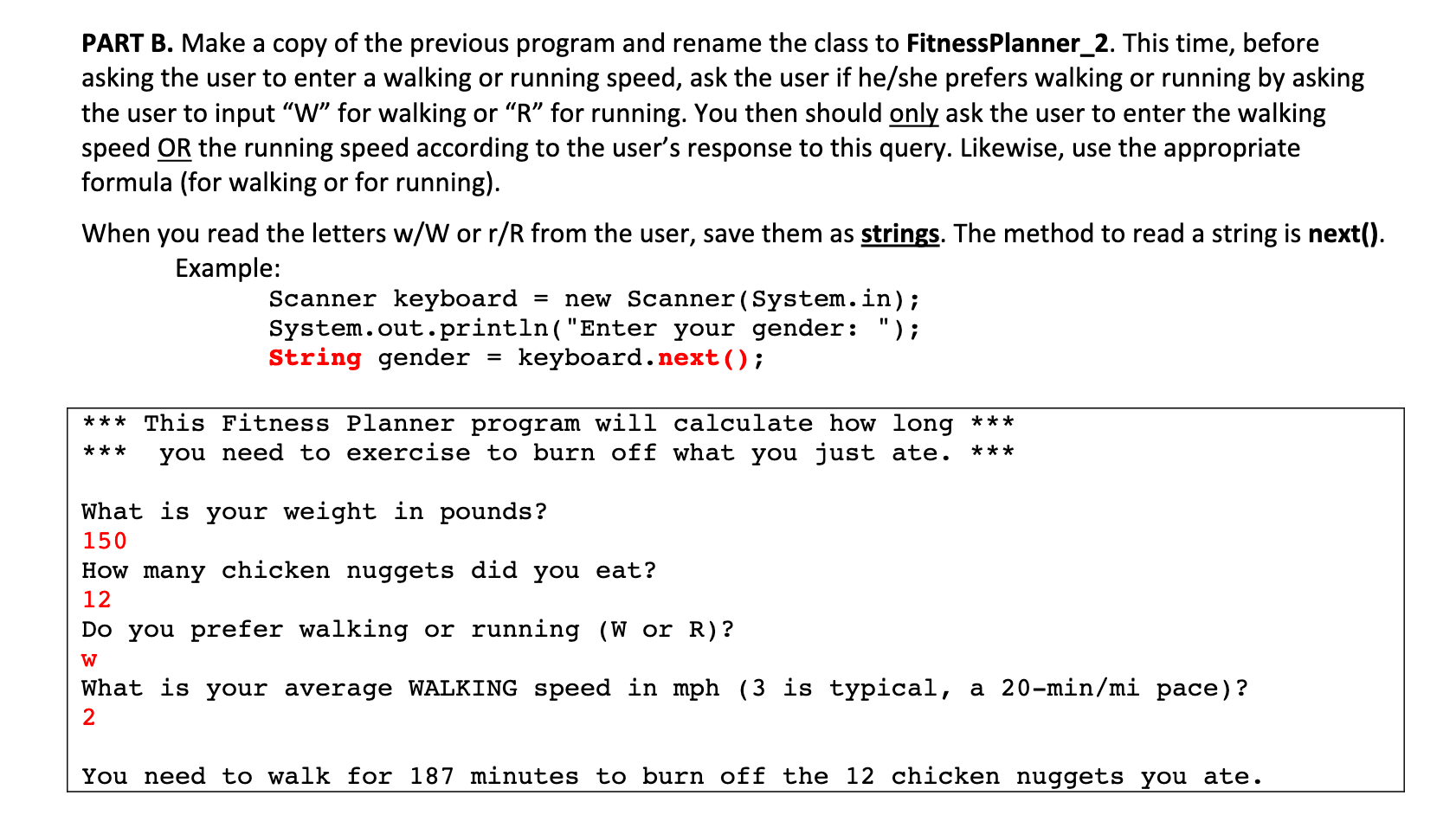
Transcribed Image Text:PART B. Make a copy of the previous program and rename the class to FitnessPlanner_2. This time, before
asking the user to enter a walking or running speed, ask the user if he/she prefers walking or running by asking
the user to input "W" for walking or "R" for running. You then should only ask the user to enter the walking
speed OR the running speed according to the user's response to this query. Likewise, use the appropriate
formula (for walking or for running).
When you read the letters w/W or r/R from the user, save them as strings. The method to read a string is next().
Example:
Scanner keyboard
System.out.println("Enter your gender: ");
String gender
= new Scanner (System.in);
= keyboard.next();
*** This Fitness Planner program will calculate how long ***
you need to exercise to burn off what you just ate.
***
***
What is your weight in pounds?
150
How many chicken nuggets did you eat?
12
Do you prefer walking or running (W or R)?
What is your average WALKING speed in mph (3 is typical, a 20-min/mi pace)?
2
You need to walk for 187 minutes to burn off the 12 chicken nuggets you ate.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
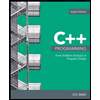
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
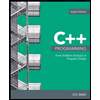
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning