| The code in Part 1 counts the crossings and nestings for a given matching. We now want to use this to analyze the distribution of crossings and nestings for arc diagrams with 100 arcs. Using your function random_matching from Exercise [1.4], generate 104 such arc diagrams and create histograms for the numbers of crossings and nestings, respectively. Display them in a single plot. Also generate a two-dimensional histogram for the joint distribution of crossings and nestings. What do you observe? [1.4] The numpy module contains functions to generate random permutations. Given a non-negative integer n, every permutation of 2n numbers can be turned into a matching by taking the first half of the permutation entries (i.e., the first n numbers) and connecting each of them to the corresponding entry in the second half (i.e., the last n numbers). Use this method to write a function random_matching that takes n and returns a randomly selected matching of 2n numbers in the form of a list of n tuples, where each tuple contains two integers. Note: Ensure that the tuples are returned such that the first integer is less than the second integer. To demonstrate that your code works, draw the arc diagrams for five randomly chosen matchings of 20 numbers. import numpy as np def random_matching(n): # Generate a random permutation of 2n numbers permutation = np.random. permutation (2*n) # Create the matching from the permutation matching = [(min(permutation[i], permutation[i+n]), max(permutation[i], permutation [i+n])) for i in range(n)] return matching Now, let's use this function to generate five randomly chosen matchings of 20 numbers and draw their arc diagrams: import matplotlib.pyplot as plt def draw_arc_diagram (matching): for pair in matching: plt.plot([pair[0], pair[1]], [1, 1], 'k-') plt.plot(pair[0], 1, 'ro') plt.plot(pair[1], 1, 'ro') plt.yticks([]) plt.show() # Generate and draw five random matchings for i in range(5): matching = random_matching (10) # 10 for 20 numbers print("Matching", i+1, ":", matching) draw_arc_diagram (matching)
| The code in Part 1 counts the crossings and nestings for a given matching. We now want to use this to analyze the distribution of crossings and nestings for arc diagrams with 100 arcs. Using your function random_matching from Exercise [1.4], generate 104 such arc diagrams and create histograms for the numbers of crossings and nestings, respectively. Display them in a single plot. Also generate a two-dimensional histogram for the joint distribution of crossings and nestings. What do you observe? [1.4] The numpy module contains functions to generate random permutations. Given a non-negative integer n, every permutation of 2n numbers can be turned into a matching by taking the first half of the permutation entries (i.e., the first n numbers) and connecting each of them to the corresponding entry in the second half (i.e., the last n numbers). Use this method to write a function random_matching that takes n and returns a randomly selected matching of 2n numbers in the form of a list of n tuples, where each tuple contains two integers. Note: Ensure that the tuples are returned such that the first integer is less than the second integer. To demonstrate that your code works, draw the arc diagrams for five randomly chosen matchings of 20 numbers. import numpy as np def random_matching(n): # Generate a random permutation of 2n numbers permutation = np.random. permutation (2*n) # Create the matching from the permutation matching = [(min(permutation[i], permutation[i+n]), max(permutation[i], permutation [i+n])) for i in range(n)] return matching Now, let's use this function to generate five randomly chosen matchings of 20 numbers and draw their arc diagrams: import matplotlib.pyplot as plt def draw_arc_diagram (matching): for pair in matching: plt.plot([pair[0], pair[1]], [1, 1], 'k-') plt.plot(pair[0], 1, 'ro') plt.plot(pair[1], 1, 'ro') plt.yticks([]) plt.show() # Generate and draw five random matchings for i in range(5): matching = random_matching (10) # 10 for 20 numbers print("Matching", i+1, ":", matching) draw_arc_diagram (matching)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
![| The code in Part 1 counts the crossings and nestings for a given matching. We now want to use this to analyze the distribution of crossings and
nestings for arc diagrams with 100 arcs.
Using your function random_matching from Exercise [1.4], generate 104 such arc diagrams and create histograms for the numbers of crossings and nestings,
respectively. Display them in a single plot. Also generate a two-dimensional histogram for the joint distribution of crossings and nestings.
What do you observe?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F033c0d82-90fb-4c58-9b03-1325bfffdb8d%2F712509ea-c395-4fc6-9ea5-a14f893997c0%2F74punu_processed.png&w=3840&q=75)
Transcribed Image Text:| The code in Part 1 counts the crossings and nestings for a given matching. We now want to use this to analyze the distribution of crossings and
nestings for arc diagrams with 100 arcs.
Using your function random_matching from Exercise [1.4], generate 104 such arc diagrams and create histograms for the numbers of crossings and nestings,
respectively. Display them in a single plot. Also generate a two-dimensional histogram for the joint distribution of crossings and nestings.
What do you observe?
![[1.4]
The numpy module contains functions to generate random permutations. Given a non-negative integer n, every permutation of 2n numbers can be
turned into a matching by taking the first half of the permutation entries (i.e., the first n numbers) and connecting each of them to the corresponding entry in the second
half (i.e., the last n numbers). Use this method to write a function random_matching that takes n and returns a randomly selected matching of 2n numbers in the form
of a list of n tuples, where each tuple contains two integers.
Note: Ensure that the tuples are returned such that the first integer is less than the second integer.
To demonstrate that your code works, draw the arc diagrams for five randomly chosen matchings of 20 numbers.
import numpy as np
def random_matching(n):
# Generate a random permutation of 2n numbers
permutation = np.random. permutation (2*n)
# Create the matching from the permutation
matching = [(min(permutation[i], permutation[i+n]), max(permutation[i], permutation [i+n])) for i in range(n)]
return matching
Now, let's use this function to generate five randomly chosen matchings of 20 numbers and draw their arc diagrams:
import matplotlib.pyplot as plt
def draw_arc_diagram (matching):
for pair in matching:
plt.plot([pair[0], pair[1]], [1, 1], 'k-')
plt.plot(pair[0], 1, 'ro')
plt.plot(pair[1], 1, 'ro')
plt.yticks([])
plt.show()
# Generate and draw five random matchings
for i in range(5):
matching = random_matching (10) # 10 for 20 numbers
print("Matching", i+1, ":", matching)
draw_arc_diagram (matching)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F033c0d82-90fb-4c58-9b03-1325bfffdb8d%2F712509ea-c395-4fc6-9ea5-a14f893997c0%2Fjtwibrv_processed.png&w=3840&q=75)
Transcribed Image Text:[1.4]
The numpy module contains functions to generate random permutations. Given a non-negative integer n, every permutation of 2n numbers can be
turned into a matching by taking the first half of the permutation entries (i.e., the first n numbers) and connecting each of them to the corresponding entry in the second
half (i.e., the last n numbers). Use this method to write a function random_matching that takes n and returns a randomly selected matching of 2n numbers in the form
of a list of n tuples, where each tuple contains two integers.
Note: Ensure that the tuples are returned such that the first integer is less than the second integer.
To demonstrate that your code works, draw the arc diagrams for five randomly chosen matchings of 20 numbers.
import numpy as np
def random_matching(n):
# Generate a random permutation of 2n numbers
permutation = np.random. permutation (2*n)
# Create the matching from the permutation
matching = [(min(permutation[i], permutation[i+n]), max(permutation[i], permutation [i+n])) for i in range(n)]
return matching
Now, let's use this function to generate five randomly chosen matchings of 20 numbers and draw their arc diagrams:
import matplotlib.pyplot as plt
def draw_arc_diagram (matching):
for pair in matching:
plt.plot([pair[0], pair[1]], [1, 1], 'k-')
plt.plot(pair[0], 1, 'ro')
plt.plot(pair[1], 1, 'ro')
plt.yticks([])
plt.show()
# Generate and draw five random matchings
for i in range(5):
matching = random_matching (10) # 10 for 20 numbers
print("Matching", i+1, ":", matching)
draw_arc_diagram (matching)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
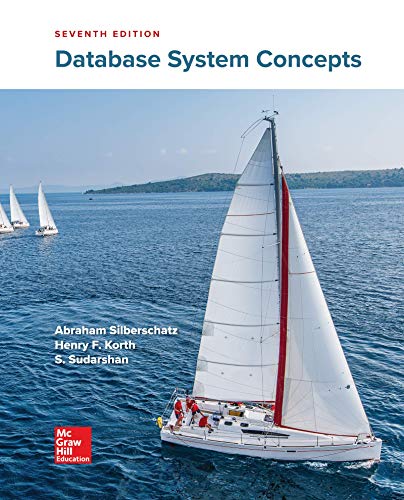
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
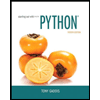
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
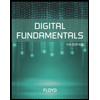
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
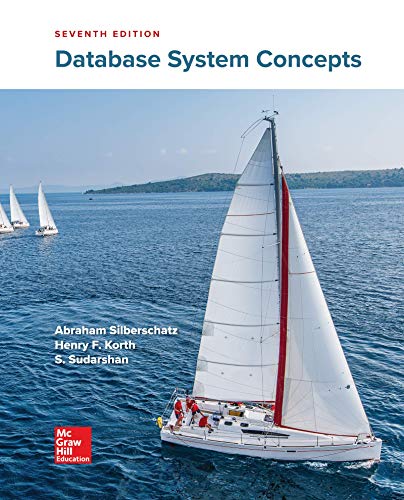
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
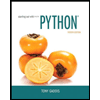
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
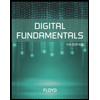
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
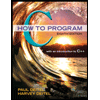
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
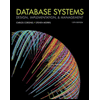
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
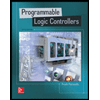
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education