or this assignment, you will write a program that reads employee work data from a text file, and then calculates payroll information based on this file. The text file will contain one line of text for each employee. Each line consists of the following data (delimited by spaces or tabs): 1) employee’s id 2) employee’s hourly wage rate 3) hours worked Monday 4) hours worked Tuesday 5) hours worked Wednesday 6) hours worked Thursday 7) hours worked Friday ex) txt file attached Your program should first prompt the user to get the location of the input file. Then it will read the data from the file, put it in appropriate arrays (as described below), and output to the screen the following information: (1) the employee ID, total number of hours worked, regular pay, overtime hours, overtime pay, and total paid to each employee (2) the total hours worked by all employees throughout the week; (3) the total amount paid out to all employees for the week; (4) the employee who received the highest pay for the week, as well as the amount that employee was paid; (5) the employees who worked less than the average number of hours; and (6) a list of the employees in reverse order. You must use standard input stream (easily done using Scanner) and standard output stream for your user IO in this assignment. When the program begins, you will see a prompt for the file path and the user should enter the value using the standard input stream (in Netbeans this is the output screen). After the user enters the input path of the file, the results for the data file shown above should look like this: attached. Note: overtime pay is time-and-a-half. So, the per hour payment for time above 40 hours should be calculated accordingly. This is shown with emp4, who worked six hours of overtime at 50% over their normal $13.75 per hour. You can see that, in addition to each employee’s details, also displayed are the totals, highest paid employee, employees working less than average hours, and employees in reverse order. Your program must be flexible enough to handle a variety of input files. There may be as many as 100 employees in the file. But in all cases, each line of the text file will be structured as described above. To make this work, you will need to create three arrays. One array will contain the employee names. A second array will contain the hourly wages earned by each employee. The third contains the total number of hours worked by each employee. These are parallel arrays. This means that the same index in each array pertains to the same entity. These arrays must be large enough to handle an unknown number of employees. As stated above, there can be as many as 100 employees in the file. But there may also be less than 100. Therefore, although these arrays must be large enough for the maximum amount, many of the elements of the arrays may be empty. So, you should also have a variable to keep track of how many employees are actually read from the file. This total employees variable will also be used to keep track of the next available element in the names and wages arrays, and can be used to index into these arrays. For example, the total employees variable starts at 0, which means that you will index into element 0 of these arrays. For each employee that you read from the file, you can increment the total employees variable. Thus, after you have processed the first employee, the total employees variable will be 1, so you will index into element #1 of the arrays. After you’ve processed the second employee from the file, the total employees variable will be 2, so you will index into element #2 of the arrays. Thus, the total employees variable serves two purposes: keeping a running count of the employees and indexing into the arrays. You will also need other variables for keeping track of totals, inputting data from the file, finding the maximum value in the array, etc. Program Logic: The logic of the program works something like this: 1) Prompt the user for the name of the file, and read response. 2) Open the input file specified by the user 3) Loop continuously: a. Read the name and wage b. Read the five days hours work and add them together c. Place these values into the appropriate arrays at the next available position d. add one to the count of total employees. You can use the total employee count to index into the arrays. 4) At this point you have accumulated all the data into the arrays. Now it is simply a matter of displaying the results, determining the max-paid employee, displaying the employees working less than average hours, and printing the names in reverse order. Each of these will be done in a loop. Keep in mind that when you calculate the pay for an employee, you need to account for overtime. Any hours over 40 that an employee works within the week should be paid at 1.5 times their normal pay rate. For the above data, emp4 shows an example of this.
For this assignment, you will write a
employee. Each line consists of the following data (delimited by spaces or tabs):
1) employee’s id
2) employee’s hourly wage rate
3) hours worked Monday
4) hours worked Tuesday
5) hours worked Wednesday
6) hours worked Thursday
7) hours worked Friday
ex) txt file attached
Your program should first prompt the user to get the location of the input file. Then it will read the data from the file, put it in appropriate arrays (as described below), and output to the screen the following information: (1) the employee ID, total number of hours worked, regular pay, overtime hours, overtime pay, and total paid to each employee (2) the total hours worked by all employees throughout the week; (3) the total amount paid out to all employees for the week; (4) the employee who received the highest pay for the week, as well as the amount that employee was paid; (5) the employees who worked less than the
average number of hours; and (6) a list of the employees in reverse order. You must use standard input stream (easily done using Scanner) and standard output stream for your user IO in this assignment.
When the program begins, you will see a prompt for the file path and the user should enter the value using the standard input stream (in Netbeans this is the output screen). After the user enters the input path of the file, the results for the data file shown above should look like this: attached.
Note: overtime pay is time-and-a-half. So, the per hour payment for time above 40 hours should be calculated accordingly. This is shown with emp4, who worked six hours of overtime at 50% over their normal $13.75 per hour. You can see that, in addition to each employee’s details, also displayed are the totals, highest paid employee, employees working less than average hours, and employees in reverse order. Your program must be flexible enough to handle a variety of input files. There may be as many as 100
employees in the file. But in all cases, each line of the text file will be structured as described above.
To make this work, you will need to create three arrays.
One array will contain the employee names. A second array will contain the hourly wages earned by each employee. The third contains the total number of hours worked by each employee. These are parallel arrays. This means that the same index in each array pertains to the same entity.
These arrays must be large enough to handle an unknown number of employees. As stated above, there can be as many as 100 employees in the file. But there may also be less than 100. Therefore, although these arrays must be large enough for the maximum amount, many of the elements of the arrays may be empty. So, you should also have a variable to keep track of how many employees are actually read from the file. This total employees variable will also be used to keep track of the next available element in the names and wages arrays, and can be used to index into these arrays. For example, the total employees variable starts at 0, which means that you will index into element 0 of these arrays. For each employee that you read from the file, you can increment the total employees variable. Thus, after you have processed the first employee, the total employees variable will be 1, so you will index into element #1 of the arrays. After you’ve processed the second employee from the file, the total employees variable will be 2, so you will index into element #2 of the arrays. Thus, the total employees variable serves two purposes: keeping a running count of the employees and indexing into the arrays. You will also need other variables for keeping track of totals, inputting data from the file, finding the maximum value in the array, etc.
Program Logic:
The logic of the program works something like this:
1) Prompt the user for the name of the file, and read response.
2) Open the input file specified by the user
3) Loop continuously:
a. Read the name and wage
b. Read the five days hours work and add them together
c. Place these values into the appropriate arrays at the next available position
d. add one to the count of total employees. You can use the total employee count to index
into the arrays.
4) At this point you have accumulated all the data into the arrays. Now it is simply a matter of displaying the results, determining the max-paid employee, displaying the employees working less than average hours, and printing the names in reverse order. Each of these will be done in a loop. Keep in mind that when you calculate the pay for an employee, you need to account for overtime. Any hours over 40 that an employee works within the week should be paid at 1.5 times their normal pay rate. For the above data, emp4 shows an example of this.
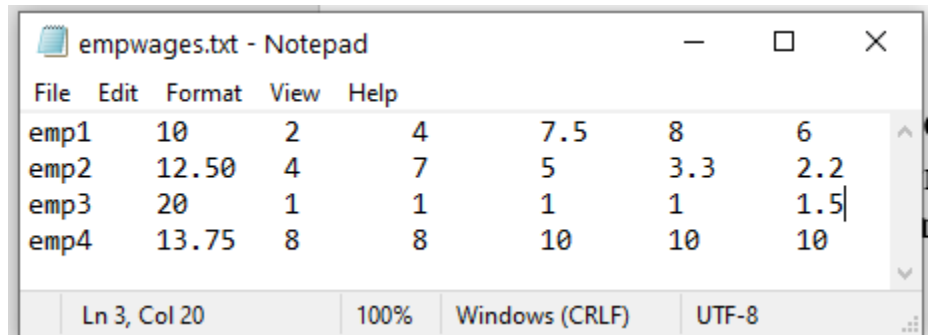
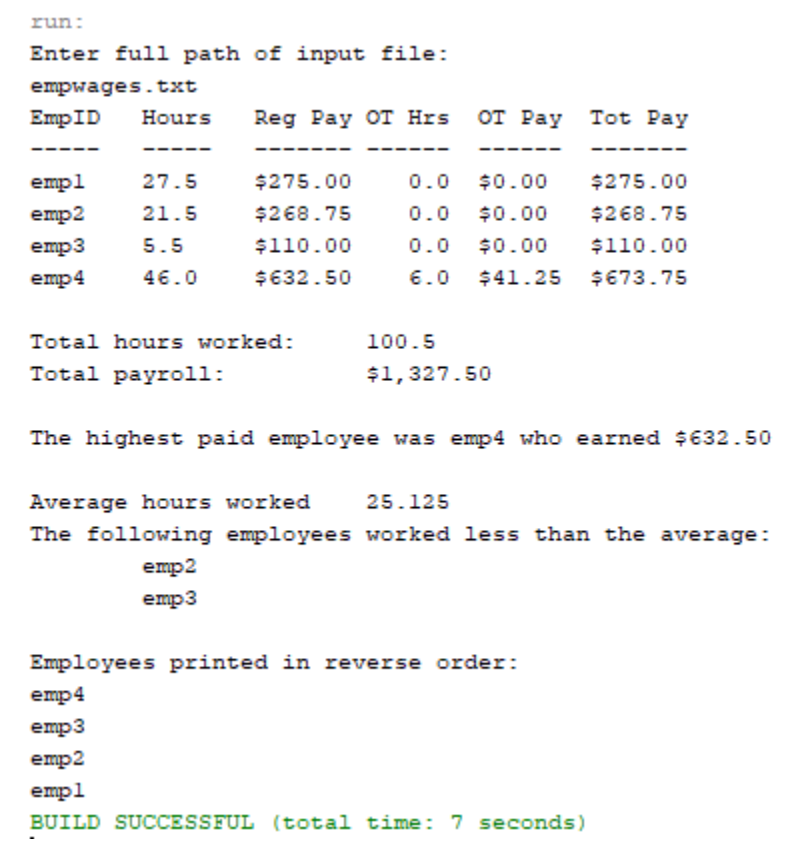

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

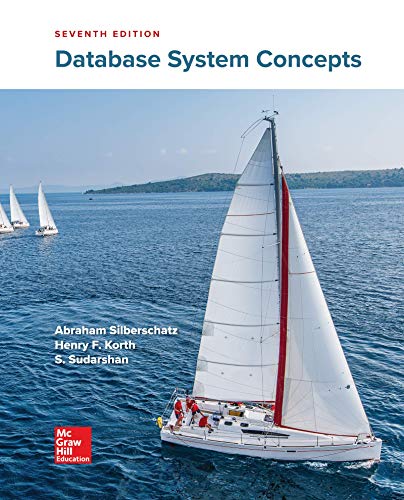
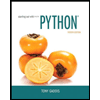
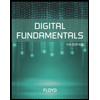
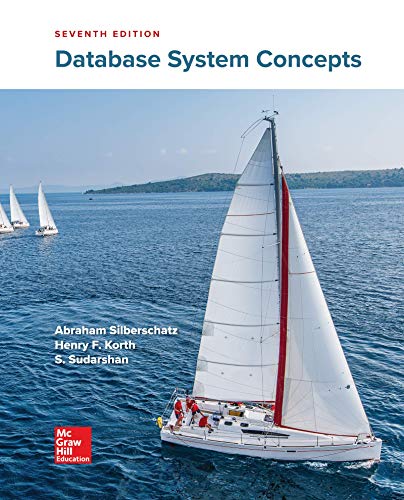
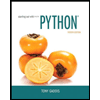
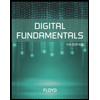
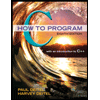
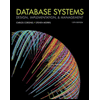
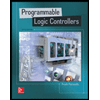