P1 Suppose we want to create a method for the class BinaryTree (file BinaryTree.java) that counts the number of times an object occurs in the tree. a. Write the method public int count1(T anObject) which calls the private recursive method private int count1(BinaryNode rootNode, T anObject) to count the number of occurrences of anObject b. Write the method public int count2(T anObject) that counts the number of occurrences of anObject and that uses one of the iterators of the binary tree. Compare the efficiencies of the previous the two methods count1 and count2 using big O notation. Add your answer as a comment before the function definition
P1 Suppose we want to create a method for the class BinaryTree (file BinaryTree.java) that counts the number of times an object occurs in the tree. a. Write the method public int count1(T anObject) which calls the private recursive method private int count1(BinaryNode rootNode, T anObject) to count the number of occurrences of anObject b. Write the method public int count2(T anObject) that counts the number of occurrences of anObject and that uses one of the iterators of the binary tree. Compare the efficiencies of the previous the two methods count1 and count2 using big O notation. Add your answer as a comment before the function definition
Related questions
Question
P1
Suppose we want to create a method for the class BinaryTree (file BinaryTree.java) that
counts the number of times an object occurs in the tree.
a. Write the method
public int count1(T anObject)
which calls the private recursive method
private int count1(BinaryNode<T> rootNode, T anObject)
to count the number of occurrences of anObject
b. Write the method
public int count2(T anObject)
that counts the number of occurrences of anObject and that uses one of the iterators of the
binary tree.
Compare the efficiencies of the previous the two methods count1 and count2 using big O
notation. Add your answer as a comment before the function definition
Suppose we want to create a method for the class BinaryTree (file BinaryTree.java) that
counts the number of times an object occurs in the tree.
a. Write the method
public int count1(T anObject)
which calls the private recursive method
private int count1(BinaryNode<T> rootNode, T anObject)
to count the number of occurrences of anObject
b. Write the method
public int count2(T anObject)
that counts the number of occurrences of anObject and that uses one of the iterators of the
binary tree.
Compare the efficiencies of the previous the two methods count1 and count2 using big O
notation. Add your answer as a comment before the function definition
P2
Suppose we want to create a method for the class BinaryTree that decides whether two trees
have the same structure. Two trees t1 and t2 have the same structure if:
- If one has a left child, then both have left children and the left children are isomorphic,
AND
- if one has a right child, then both have right children and the right children are
isomorphic
The header of the method is:
public boolean isIsomorphic(BinaryTree<T> otherTree)
Write this method, using a private recursive method of the same name.
P3
Design an algorithm that produces a binary expression tree from a given infix expression. You
can assume that the infix expression is a string that has only the binary operators +, -, *, /,
parantheses, and one-letter operands. Implement the solution as a construction in
ExpressionTree that takes a string argument:
public ExpressionTree(String infix)
that calls the private method
private ExpressionTree formTree(String expr, int first, int last)
to construct the tree. formTree() builds the tree recursively where first and last are the first and
last index in the string corresponding to the tree you want to construc
isomorphic
The header of the method is:
public boolean isIsomorphic(BinaryTree<T> otherTree)
Write this method, using a private recursive method of the same name.
P3
Design an algorithm that produces a binary expression tree from a given infix expression. You
can assume that the infix expression is a string that has only the binary operators +, -, *, /,
parantheses, and one-letter operands. Implement the solution as a construction in
ExpressionTree that takes a string argument:
public ExpressionTree(String infix)
that calls the private method
private ExpressionTree formTree(String expr, int first, int last)
to construct the tree. formTree() builds the tree recursively where first and last are the first and
last index in the string corresponding to the tree you want to construc
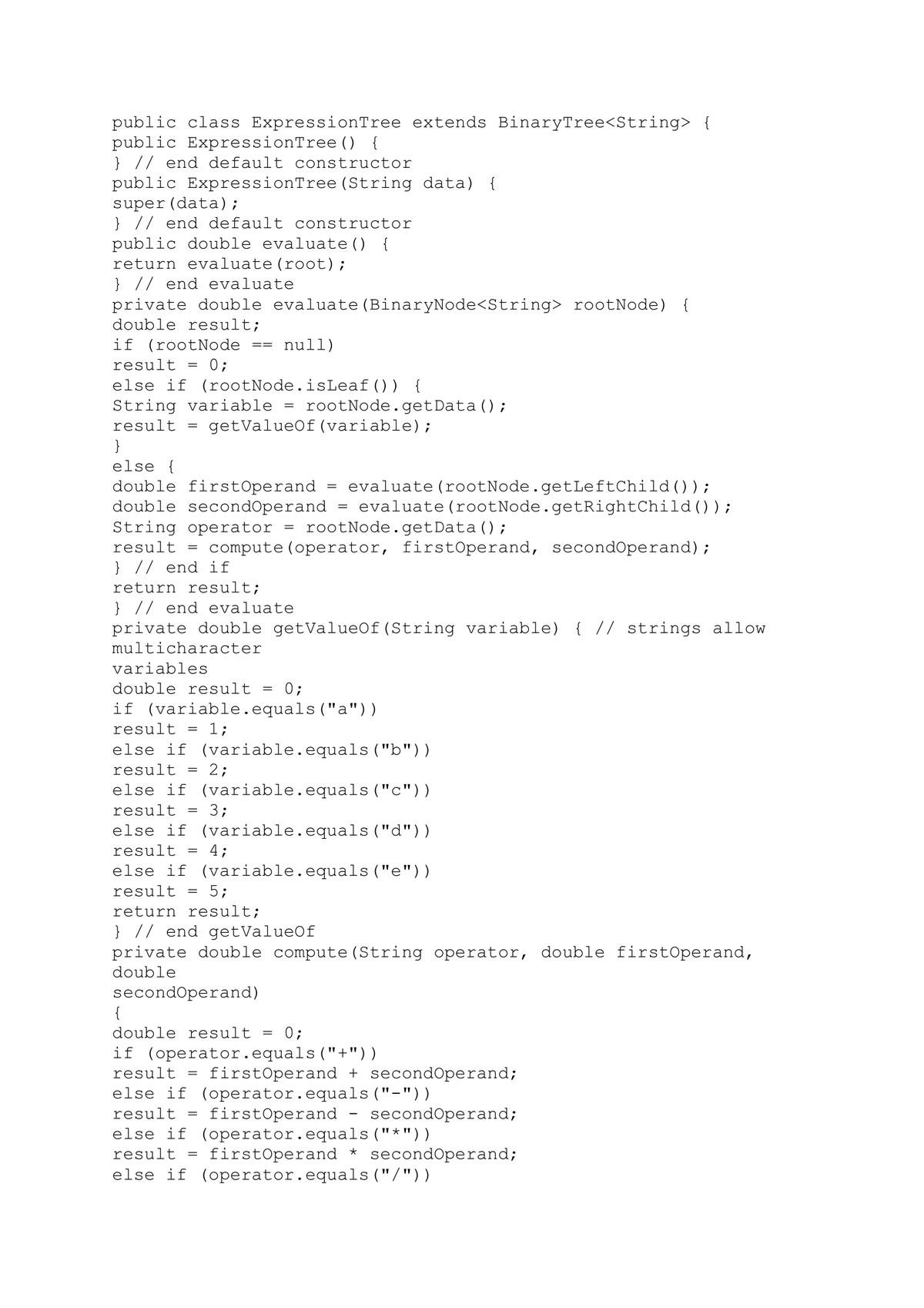
Transcribed Image Text:public class ExpressionTree extends BinaryTree<String> {
public ExpressionTree () {
} // end default constructor
public ExpressionTree (String data) {
super (data);
} // end default constructor
public double evaluate() {
return evaluate (root);
} // end evaluate
private double evaluate (BinaryNode<String> rootNode) {
double result;
null)
if (rootNode
result = 0;
else if (rootNode.isLeaf ()) {
String variable
result = getValueOf (variable);
==
}
else {
double first operand evaluate (rootNode.getLeft Child());
double secondOperand evaluate (rootNode.getRightChild());
String operator = rootNode.getData();
result = compute (operator, firstOperand, secondOperand);
} // end if
return result;
} // end evaluate
private double getValueOf (String variable) { // strings allow
=
=
=
multicharacter
variables
double result =
if (variable.equals("a"))
3;
rootNode.getData();
result = = 1;
else if (variable.equals("b"))
result = 2;
else if (variable.equals("c"))
result
else if (variable.equals("d"))
result =
4;
else if (variable.equals("e"))
result
5;
return result;
} // end getValueOf
private double compute (String operator, double firstOperand,
double
secondOperand)
0;
=
{
double result = 0;
if (operator.equals("+"))
result = firstOperand + secondOperand;
else if (operator.equals("-"))
result = firstOperand
secondOperand;
else if (operator.equals("*"))
result = firstOperand * secondOperand;
else if (operator.equals("/"))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps
