Palindromes A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad" and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of leng one. Write some code in the main () function to accomplish the following tasks: Having written code to extract data from the keyboard (stdin) in Lab 3, reuse that co to prompt the user to enter a string between 0 and 255 characters in length. You shou ignore whitespace and punctuation, and all comparisons should be case-insensitive. Process the entries from the keyboard and store them in the queue ADT provided in tl lab 5 code package. Use the is_palindrome () function ADT provided to implement a simple palindrome verification of the data entered. Here is the signature and documentation for the functi bool is_palindrome (char *text) Return true if text is a palindrome, false otherwise. Turn in your commented program and a screen shot (script)of the execution run of the program. Include some sample tests in your captured code run. Examples of valid palindrom "a" "aa" "aaa" "aba" "abba" "Тасо cat" "Madam, I'm Adam" "A man, a plan, a canal: Panama" "Doc, note: I dissent. A fast never prevents a fatness. I diet on cod."
Palindromes A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad" and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of leng one. Write some code in the main () function to accomplish the following tasks: Having written code to extract data from the keyboard (stdin) in Lab 3, reuse that co to prompt the user to enter a string between 0 and 255 characters in length. You shou ignore whitespace and punctuation, and all comparisons should be case-insensitive. Process the entries from the keyboard and store them in the queue ADT provided in tl lab 5 code package. Use the is_palindrome () function ADT provided to implement a simple palindrome verification of the data entered. Here is the signature and documentation for the functi bool is_palindrome (char *text) Return true if text is a palindrome, false otherwise. Turn in your commented program and a screen shot (script)of the execution run of the program. Include some sample tests in your captured code run. Examples of valid palindrom "a" "aa" "aaa" "aba" "abba" "Тасо cat" "Madam, I'm Adam" "A man, a plan, a canal: Panama" "Doc, note: I dissent. A fast never prevents a fatness. I diet on cod."
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C
How do I code the main.c file code so that it completes the tasking assigned?
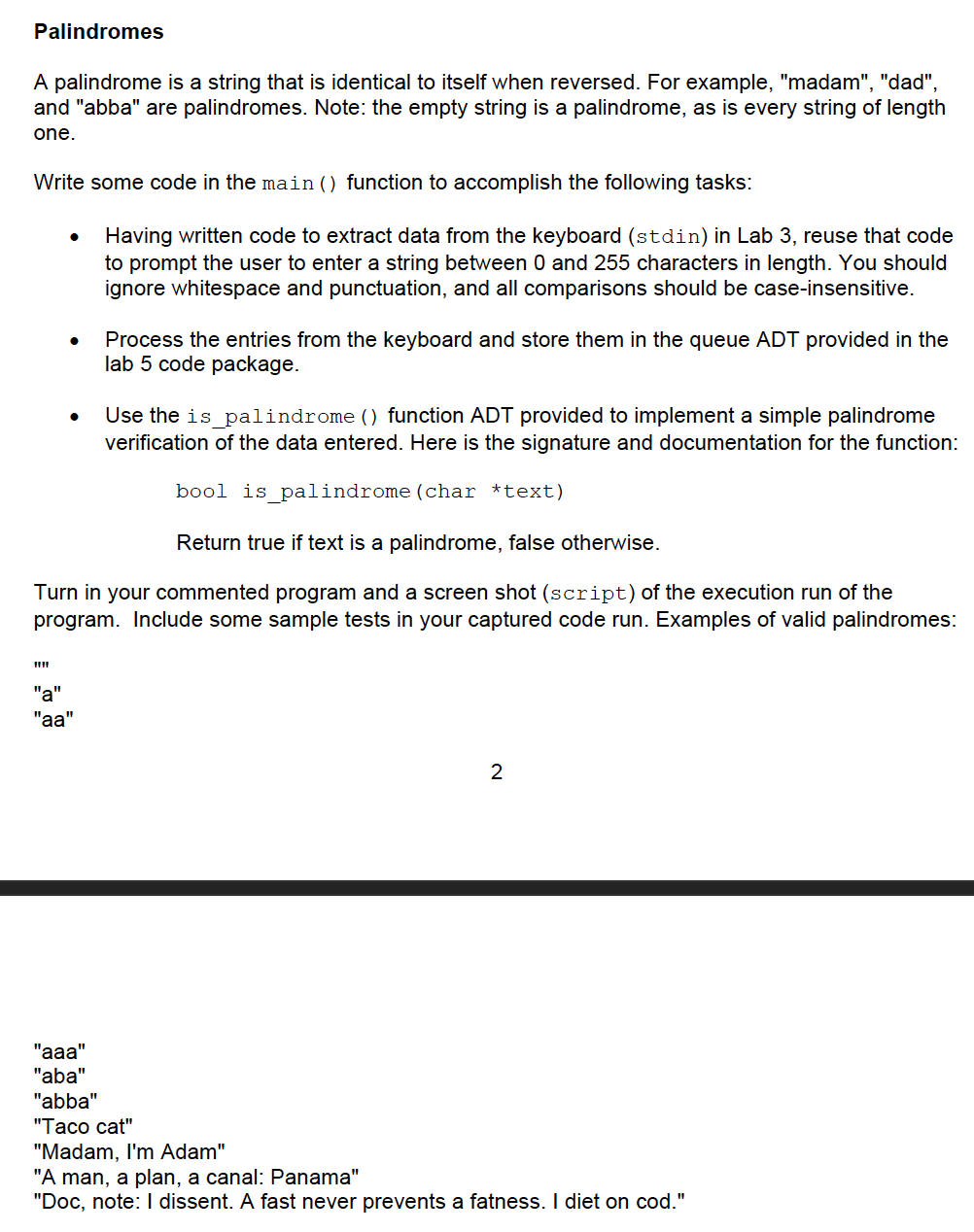
Transcribed Image Text:Palindromes
A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad",
and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of length
one.
Write some code in the main () function to accomplish the following tasks:
Having written code to extract data from the keyboard (stdin) in Lab 3, reuse that code
to prompt the user to enter a string between 0 and 255 characters in length. You should
ignore whitespace and punctuation, and all comparisons should be case-insensitive.
Process the entries from the keyboard and store them in the queue ADT provided in the
lab 5 code package.
Use the is_palindrome () function ADT provided to implement a simple palindrome
verification of the data entered. Here is the signature and documentation for the function:
bool is palindrome (char *text)
Return true if text is a palindrome, false otherwise.
Turn in your commented program and a screen shot (script) of the execution run of the
program. Include some sample tests in your captured code run. Examples of valid palindromes:
"a"
"aa"
"aaa"
"aba"
"abba"
"Тасо сat"
"Madam, I'm Adam"
"A man, a plan, a canal: Panama"
"Doc, note: I dissent. A fast never prevents a fatness. I diet on cod."
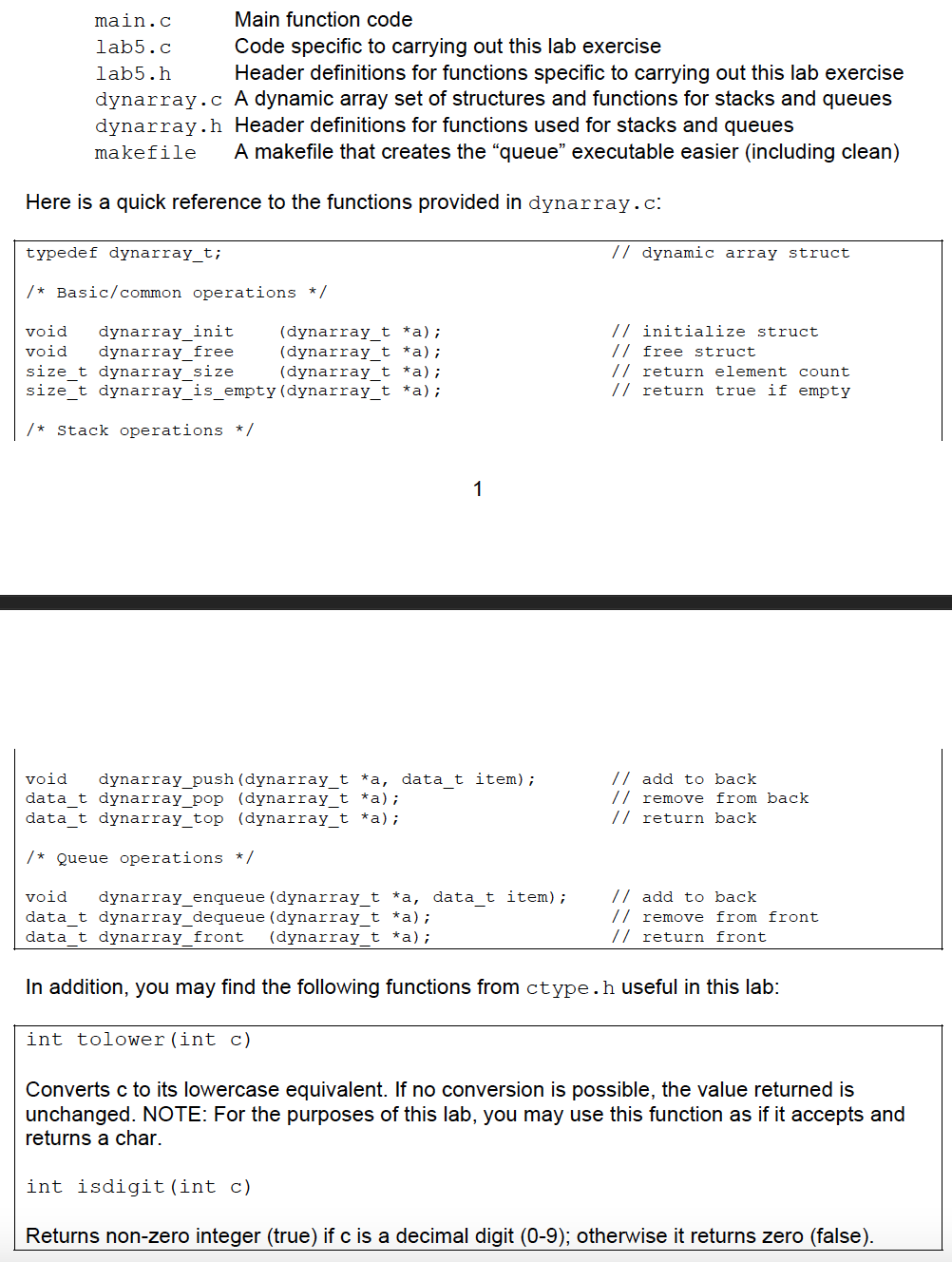
Transcribed Image Text:main.c
Main function code
lab5.c
Code specific to carrying out this lab exercise
lab5.h
Header definitions for functions specific to carrying out this lab exercise
dynarray.c A dynamic array set of structures and functions for stacks and queues
dynarray.h Header definitions for functions used for stacks and queues
makefile
A makefile that creates the "queue" executable easier (including clean)
Here is a quick reference to the functions provided in dynarray.c:
typedef dynarray_t;
// dynamic array struct
/* Basic/common operations */
// initialize struct
// free struct
void
dynarray_init
dynarray_free
size_t dynarray_size
(dynarray_t *a);
(dynarray_t *a);
(dynarray_t *a);
size_t dynarray_is_empty(dynarray_t *a);
void
// return element count
// return true if empty
/* Stack operations * /
1
// add to back
// remove from back
// return back
void
dynarray_push (dynarray_t *a, data_t item);
data_t dynarray_pop (dynarray_t *a);
data_t dynarray_top (dynarray_t *a);
/* Queue operations * /
// add to back
// remove from front
// return front
void
dynarray_enqueue (dynarray_t *a, data_t item);
data_t dynarray_dequeue (dynarray_t *a);
data_t dynarray_front
(dynarray_t *a);
In addition, you may find the following functions from ctype.h useful in this lab:
int tolower (int c)
Converts c to its lowercase equivalent. If no conversion is possible, the value returned is
unchanged. NOTE: For the purposes of this lab, you may use this function as if it accepts and
returns a char.
int isdigit (int c)
Returns non-zero integer (true) if c is a decimal digit (0-9); otherwise it returns zero (false).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
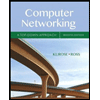
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
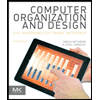
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
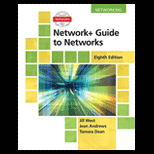
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
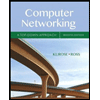
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
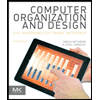
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
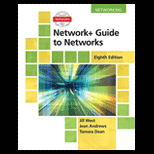
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
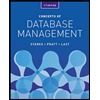
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
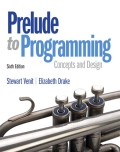
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
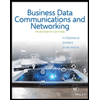
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY