screenchot of input and output import string pp = '''Peter Piper picked a peck of pickled peppers. A peck of pickled peppers Peter Piper picked. If Peter Piper picked a peck of pickled peppers Where's the peck of pickled peppers Peter Piper picked?''' def remove_punc(s): '''Returns a copy of string s with all punctuation removed''' #This function is already written - don't change it! for char in string.punctuation: s = s.replace(char, '') return s def count_words(text): '''Returns a dictionary with words and their counts''' pass #Create an empty dictionary #Split the text into a list of words #For each word in the list #If the word is not in the dictionary #Add it with a count of 1 #Otherwise #Add 1 to the count #Return the dictionary def show_counts(word_dict): '''Display words and their counts''' pass #Get a list of the keys from word_dict #Put the words in alphabetical order #For each word in the list #Print the word and its count def main(): '''Controls the program''' text = remove_punc(pp.lower()) #text is the name of the string with no punctuation #Use count_words to get a dictionary with words and counts #Use show_counts() to display words and counts main()
screenchot of input and output
import string
pp = '''Peter Piper picked a peck of pickled peppers.
A peck of pickled peppers Peter Piper picked.
If Peter Piper picked a peck of pickled peppers
Where's the peck of pickled peppers Peter Piper picked?'''
def remove_punc(s):
'''Returns a copy of string s with all punctuation removed'''
#This function is already written - don't change it!
for char in string.punctuation:
s = s.replace(char, '')
return s
def count_words(text):
'''Returns a dictionary with words and their counts'''
pass
#Create an empty dictionary
#Split the text into a list of words
#For each word in the list
#If the word is not in the dictionary
#Add it with a count of 1
#Otherwise
#Add 1 to the count
#Return the dictionary
def show_counts(word_dict):
'''Display words and their counts'''
pass
#Get a list of the keys from word_dict
#Put the words in alphabetical order
#For each word in the list
#Print the word and its count
def main():
'''Controls the program'''
text = remove_punc(pp.lower()) #text is the name of the string with no punctuation
#Use count_words to get a dictionary with words and counts
#Use show_counts() to display words and counts
main()
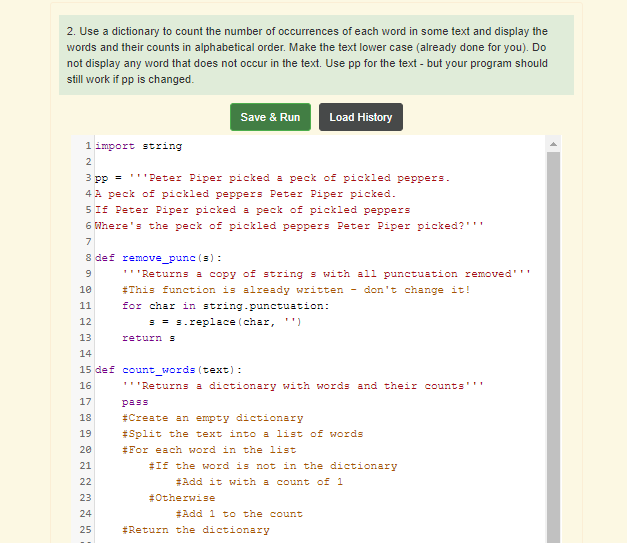
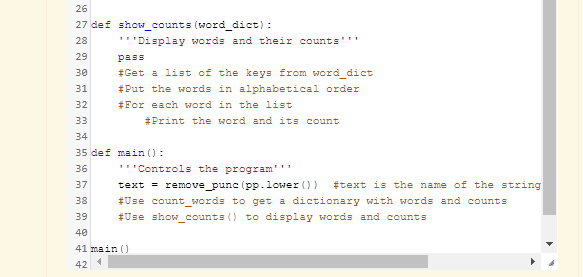

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

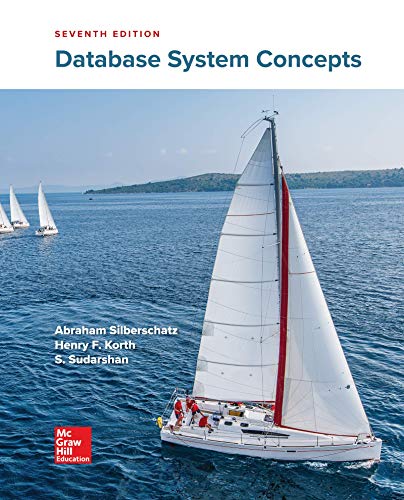
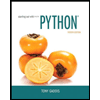
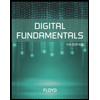
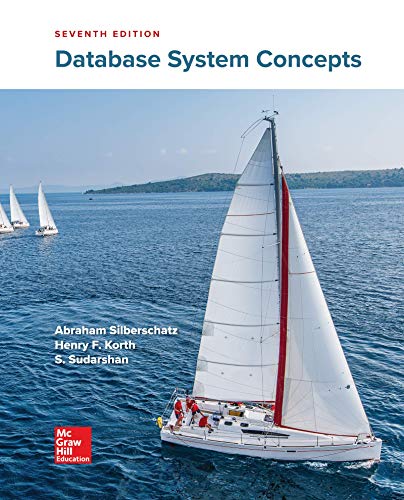
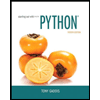
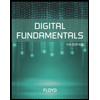
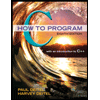
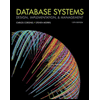
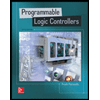