Part 1: Binary Representation (Base 2) As the textbook mentions, binary is a convenient number system for representing the electronic charges of a computer. At their core, all computers store all of their data in binary. That means whenever you see numbers on your screen or in your programs, they are actually being stored internally in binary. The number system we use on a day-to-day basis is called decimal, or base 10. This means that each digit of our normal number system has 10 possible values (0-9) and the position of each digit going from right to left represents increasing powers of 10. For example, the number 1,083 can be broken down like so: 1 0 8 3 1000's place 100's place 10's place 1's place 10^3 10^2 10^1 10^0 (10^3 * 1) + (10^2 * 0) + (10^1 * 8) + (10^0 * 3) = (1000 * 1) + (100 * 0) + (10 * 8) + (1 * 3) = 1000 + 0 + 80 + 3 = 1083 The same process is used for binary numbers, except that binary is the base 2 number system. So each digit has 2 possible values (0 and 1), and the position of each digit going from right to left represents powers of 2. Take the binary number 10110 for example: 1 0 1 1 0 16's place 8's place 4's place 2's place 1's place 2^4 2^3 2^2 2^1 2^0 (2^4 *1) + (2^3 * 0) + (2^2 * 1) + (2^1 * 1) + (2^0 * 0) = (16 * 1) + (8 * 0) + (4 * 1) + (2 * 1) + (1 * 0) = 16 + 0 + 4 + 2 + 0 = 22 So the number 22 is represented in binary as 10110. Exercise 1: Convert the binary number 1000 into decimal. What is that number? Exercise 2: Convert the binary number 1110101 into decimal. What is that number? Converting decimal to binary is a bit trickier. We need to split our decimal number into its components that make up the powers of 2. The easiest way to do this is by repeatedly dividing by 2 and keeping track of the remainder. Once your quotient reaches 0, the remainders in reverse order will be the binary number. Here is an example showing how to represent the number 43 in binary: 43 / 2 = 21, remainder 1 21 / 2 = 10, remainder 1 10 / 2 = 5, remainder 0 5 / 2 = 2, remainder 1 2 / 2 = 1, remainder 0 1 / 2 = 0, remainder 1 By writing the remainders in reverse order we get 101011. Therefore, 43 in binary is 101011. Exercise 3: Convert the number 73 into binary. What is that number? Any computer's memory can be represented using just binary numbers. A single binary digit is called a bit, and 8 bits together is called a byte. A byte is the smallest size of data that most computers store, which means the most common size of binary number we will see is 8 digits long. With a single byte we can represent numbers from 0 to 255. We will usually write our binary numbers in groups of 8 digits.
Part 1: Binary Representation (Base 2)
As the textbook mentions, binary is a convenient number system for representing the electronic charges of a computer. At their core, all computers store all of their data in binary. That means whenever you see numbers on your screen or in your programs, they are actually being stored internally in binary.
The number system we use on a day-to-day basis is called decimal, or base 10. This means that each digit of our normal number system has 10 possible values (0-9) and the position of each digit going from right to left represents increasing powers of 10. For example, the number 1,083 can be broken down like so:
1 | 0 | 8 | 3 |
1000's place | 100's place | 10's place | 1's place |
10^3 | 10^2 | 10^1 | 10^0 |
(10^3 * 1) + (10^2 * 0) + (10^1 * 8) + (10^0 * 3) =
(1000 * 1) + (100 * 0) + (10 * 8) + (1 * 3) =
1000 + 0 + 80 + 3 =
1083
The same process is used for binary numbers, except that binary is the base 2 number system. So each digit has 2 possible values (0 and 1), and the position of each digit going from right to left represents powers of 2. Take the binary number 10110 for example:
1 | 0 | 1 | 1 | 0 |
16's place | 8's place | 4's place | 2's place | 1's place |
2^4 | 2^3 | 2^2 | 2^1 | 2^0 |
(2^4 *1) + (2^3 * 0) + (2^2 * 1) + (2^1 * 1) + (2^0 * 0) =
(16 * 1) + (8 * 0) + (4 * 1) + (2 * 1) + (1 * 0) =
16 + 0 + 4 + 2 + 0 =
22
So the number 22 is represented in binary as 10110.
Exercise 1: Convert the binary number 1000 into decimal. What is that number?
Exercise 2: Convert the binary number 1110101 into decimal. What is that number?
Converting decimal to binary is a bit trickier. We need to split our decimal number into its components that make up the powers of 2. The easiest way to do this is by repeatedly dividing by 2 and keeping track of the remainder. Once your quotient reaches 0, the remainders in reverse order will be the binary number. Here is an example showing how to represent the number 43 in binary:
43 / 2 = 21, remainder 1
21 / 2 = 10, remainder 1
10 / 2 = 5, remainder 0
5 / 2 = 2, remainder 1
2 / 2 = 1, remainder 0
1 / 2 = 0, remainder 1
By writing the remainders in reverse order we get 101011.
Therefore, 43 in binary is 101011.
Exercise 3: Convert the number 73 into binary. What is that number?
Any computer's memory can be represented using just binary numbers. A single binary digit is called a bit, and 8 bits together is called a byte. A byte is the smallest size of data that most computers store, which means the most common size of binary number we will see is 8 digits long. With a single byte we can represent numbers from 0 to 255. We will usually write our binary numbers in groups of 8 digits.

Step by step
Solved in 4 steps

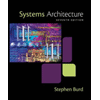
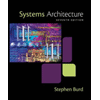