Part 1: I need to define a binary search tree data structure and need to implement the following functions: Insert Sorted BSTREE insert(BSTREE root, int num): root points to a node in a binary search tree; num is a number to be inserted in the tree rooted at “root”. This function returns the root of the modified tree. Print Elements void inorderTraversal(BSTREE root, FILE *fp): root points to a node in a binary search tree. This function does not return anything, but prints out, to the file specified, the nodes in the tree rooted at “root” by performing an inorder traversal. Part 2: Test the performance of the designed data structure using theoretical and experimental approaches as follows: Dataset 1-Dataset is sorted- Add code to insert the numbers 1...n in that order in an initially empty doubly linked list and a binary search tree. Run it on different values of n where : n = 20,000 n = 50,000 n = 100,000 Do an in-order traversal of the tree, printing out the contents to a file (named “sorted”) to verify that you indeed built the data structure correctly. Note the time for each program executions to just build the data structure (i.e., don’t include printing in the time) and include the timings in your report. For each n, you must run it 3 times and then take the average time. Don’t forget to submit all your data though. Report the results of the analyzed algorithms for each data structure using the Big-O and the timings. Dataset 2-Dataset is random - Add code to read in values from “dataToBuildDS.txt” and properly insert them in an initially empty doubly linked list and binary search tree. Run your program on different values of n by doing the following: Read in the first 20,000 entries only found in “dataToBuildDS.txt” Read in the first 50,000 entries only found in “dataToBuildDS.txt” Read in the first 100,000 entries only found in “dataToBuildDS.txt” Do an in-order traversal of the tree, printing out the contents to a file (named “unsorted”) to verify that you indeed built the data structure correctly. Note the time to build the data structure. For each n, you must run it at least 3 times and then take the average. Include all timings as well as the average in your report. Report the results of the analyzed algorithms for each data structure using the Big-O and the timings. The answer should include the following parts : Implementation of the data structures that shows the experimental study in timings for the datasets for insertion and print algorithms for each data set. Report: Describe the data structure [BST] and algorithms [insert, find and print] in pseudocode. Comparison in terms of: Big-O and datasets for insert and print Timings and datasets for insert and print
Part 1: I need to define a binary search tree data structure and need to implement the following functions: Insert Sorted BSTREE insert(BSTREE root, int num): root points to a node in a binary search tree; num is a number to be inserted in the tree rooted at “root”. This function returns the root of the modified tree. Print Elements void inorderTraversal(BSTREE root, FILE *fp): root points to a node in a binary search tree. This function does not return anything, but prints out, to the file specified, the nodes in the tree rooted at “root” by performing an inorder traversal. Part 2: Test the performance of the designed data structure using theoretical and experimental approaches as follows: Dataset 1-Dataset is sorted- Add code to insert the numbers 1...n in that order in an initially empty doubly linked list and a binary search tree. Run it on different values of n where : n = 20,000 n = 50,000 n = 100,000 Do an in-order traversal of the tree, printing out the contents to a file (named “sorted”) to verify that you indeed built the data structure correctly. Note the time for each program executions to just build the data structure (i.e., don’t include printing in the time) and include the timings in your report. For each n, you must run it 3 times and then take the average time. Don’t forget to submit all your data though. Report the results of the analyzed algorithms for each data structure using the Big-O and the timings. Dataset 2-Dataset is random - Add code to read in values from “dataToBuildDS.txt” and properly insert them in an initially empty doubly linked list and binary search tree. Run your program on different values of n by doing the following: Read in the first 20,000 entries only found in “dataToBuildDS.txt” Read in the first 50,000 entries only found in “dataToBuildDS.txt” Read in the first 100,000 entries only found in “dataToBuildDS.txt” Do an in-order traversal of the tree, printing out the contents to a file (named “unsorted”) to verify that you indeed built the data structure correctly. Note the time to build the data structure. For each n, you must run it at least 3 times and then take the average. Include all timings as well as the average in your report. Report the results of the analyzed algorithms for each data structure using the Big-O and the timings. The answer should include the following parts : Implementation of the data structures that shows the experimental study in timings for the datasets for insertion and print algorithms for each data set. Report: Describe the data structure [BST] and algorithms [insert, find and print] in pseudocode. Comparison in terms of: Big-O and datasets for insert and print Timings and datasets for insert and print
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Part 1: I need to define a binary search tree data structure and need to implement the following functions:
- Insert Sorted
- BSTREE insert(BSTREE root, int num): root points to a node in a binary search tree; num is a number to be inserted in the tree rooted at “root”. This function returns the root of the modified tree.
- Print Elements
- void inorderTraversal(BSTREE root, FILE *fp): root points to a node in a binary search tree. This function does not return anything, but prints out, to the file specified, the nodes in the tree rooted at “root” by performing an inorder traversal.
Part 2: Test the performance of the designed data structure using theoretical and experimental approaches as follows:
- Dataset 1-Dataset is sorted- Add code to insert the numbers 1...n in that order in an initially empty doubly linked list and a binary search tree.
- Run it on different values of n where :
- n = 20,000
- n = 50,000
- n = 100,000
- Do an in-order traversal of the tree, printing out the contents to a file (named “sorted”) to verify that you indeed built the data structure correctly.
- Note the time for each program executions to just build the data structure (i.e., don’t include printing in the time) and include the timings in your report. For each n, you must run it 3 times and then take the average time. Don’t forget to submit all your data though.
- Report the results of the analyzed
algorithms for each data structure using the Big-O and the timings.
- Run it on different values of n where :
- Dataset 2-Dataset is random - Add code to read in values from “dataToBuildDS.txt” and properly insert them in an initially empty doubly linked list and binary search tree.
- Run your program on different values of n by doing the following:
- Read in the first 20,000 entries only found in “dataToBuildDS.txt”
- Read in the first 50,000 entries only found in “dataToBuildDS.txt”
- Read in the first 100,000 entries only found in “dataToBuildDS.txt”
- Run your program on different values of n by doing the following:
- Do an in-order traversal of the tree, printing out the contents to a file (named “unsorted”) to verify that you indeed built the data structure correctly.
- Note the time to build the data structure. For each n, you must run it at least 3 times and then take the average. Include all timings as well as the average in your report.
- Report the results of the analyzed algorithms for each data structure using the Big-O and the timings.
The answer should include the following parts :
- Implementation of the data structures that shows the experimental study in timings for the datasets for insertion and print algorithms for each data set.
- Report:
- Describe the data structure [BST] and algorithms [insert, find and print] in pseudocode.
- Comparison in terms of:
- Big-O and datasets for insert and print
- Timings and datasets for insert and print
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
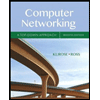
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
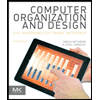
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
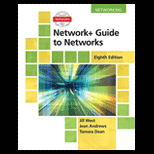
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
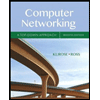
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
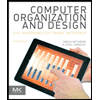
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
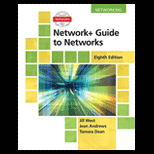
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
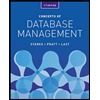
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
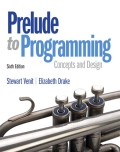
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
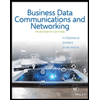
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY