F# Exercise, BST write the following binary search tree functions in F# for a binary search tree of integers. Use the following type definition for a BST (copy this into your solution): F# system functions (such as min) and the method in the List module such as List.map. are not allowed // Tree definition for problem: type BST = | Empty | TreeNode of int * BST * BST insert value tree: Inserts the value into the tree and returns the resulting tree. The resulting tree does NOT need to be balanced. If the value already exists in the tree, return the tree without inserting the value. search value tree: Returns true if the value is in the tree and false otherwise. count func tree: The parameter func is a Boolean function that takes a single parameter and returns true or false. The function tests the value of each node with func and returns the number of nodes that evaluate to true. evenCount tree: Returns the number of nodes that contain even integers. REQUIREMENT: This function must be a single call to count (part 3C) using a lambda function. Examples: > let bt1 = insert 10 Empty;; val bt1 : BST = TreeNode (10, Empty, Empty) > let bt2 = insert 5 bt1;; val bt2 : BST = TreeNode (10, TreeNode (5, Empty, Empty), Empty) > let bt3 = insert 3 bt2;; val bt3 : BST = TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), Empty) > let bt4 = insert 17 bt3;; val bt4 : BST = TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), TreeNode (17, Empty, Empty)) > let bt5 = insert 12 bt4;; val bt5 : BST = TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), TreeNode (17, TreeNode (12, Empty, Empty), Empty)) > search 17 bt5;; val it : bool = true > search 4 bt5;; val it : bool = false > evenCount bt5;; val it : int = 2
F# Exercise, BST write the following binary search tree functions in F# for a binary search tree of integers. Use the following type definition for a BST (copy this into your solution): F# system functions (such as min) and the method in the List module such as List.map. are not allowed // Tree definition for problem: type BST = | Empty | TreeNode of int * BST * BST insert value tree: Inserts the value into the tree and returns the resulting tree. The resulting tree does NOT need to be balanced. If the value already exists in the tree, return the tree without inserting the value. search value tree: Returns true if the value is in the tree and false otherwise. count func tree: The parameter func is a Boolean function that takes a single parameter and returns true or false. The function tests the value of each node with func and returns the number of nodes that evaluate to true. evenCount tree: Returns the number of nodes that contain even integers. REQUIREMENT: This function must be a single call to count (part 3C) using a lambda function. Examples: > let bt1 = insert 10 Empty;; val bt1 : BST = TreeNode (10, Empty, Empty) > let bt2 = insert 5 bt1;; val bt2 : BST = TreeNode (10, TreeNode (5, Empty, Empty), Empty) > let bt3 = insert 3 bt2;; val bt3 : BST = TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), Empty) > let bt4 = insert 17 bt3;; val bt4 : BST = TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), TreeNode (17, Empty, Empty)) > let bt5 = insert 12 bt4;; val bt5 : BST = TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), TreeNode (17, TreeNode (12, Empty, Empty), Empty)) > search 17 bt5;; val it : bool = true > search 4 bt5;; val it : bool = false > evenCount bt5;; val it : int = 2
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
F# Exercise, BST
write the following binary search tree functions in F# for a binary search tree of integers. Use the following type definition for a BST (copy this into your solution):
F# system functions (such as min) and the method in the List module such as List.map. are not allowed
// Tree definition for problem:
type BST =
| Empty
| TreeNode of int * BST * BST
- insert value tree: Inserts the value into the tree and returns the resulting tree. The resulting tree does NOT need to be balanced. If the value already exists in the tree, return the tree without inserting the value.
- search value tree: Returns true if the value is in the tree and false otherwise.
- count func tree: The parameter func is a Boolean function that takes a single parameter and returns true or false. The function tests the value of each node with func and returns the number of nodes that evaluate to true.
- evenCount tree: Returns the number of nodes that contain even integers. REQUIREMENT: This function must be a single call to count (part 3C) using a lambda function.
Examples:
> let bt1 = insert 10 Empty;;
val bt1 : BST = TreeNode (10, Empty, Empty)
> let bt2 = insert 5 bt1;;
val bt2 : BST = TreeNode (10, TreeNode (5, Empty, Empty), Empty)
> let bt3 = insert 3 bt2;;
val bt3 : BST =
TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), Empty)
> let bt4 = insert 17 bt3;;
val bt4 : BST =
TreeNode
(10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), TreeNode (17, Empty, Empty))
> let bt5 = insert 12 bt4;;
val bt5 : BST =
TreeNode
(10, TreeNode (5, TreeNode (3, Empty, Empty), Empty),
TreeNode (17, TreeNode (12, Empty, Empty), Empty))
> search 17 bt5;;
val it : bool = true
> search 4 bt5;;
val it : bool = false
> evenCount bt5;;
val it : int = 2
val bt1 : BST = TreeNode (10, Empty, Empty)
> let bt2 = insert 5 bt1;;
val bt2 : BST = TreeNode (10, TreeNode (5, Empty, Empty), Empty)
> let bt3 = insert 3 bt2;;
val bt3 : BST =
TreeNode (10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), Empty)
> let bt4 = insert 17 bt3;;
val bt4 : BST =
TreeNode
(10, TreeNode (5, TreeNode (3, Empty, Empty), Empty), TreeNode (17, Empty, Empty))
> let bt5 = insert 12 bt4;;
val bt5 : BST =
TreeNode
(10, TreeNode (5, TreeNode (3, Empty, Empty), Empty),
TreeNode (17, TreeNode (12, Empty, Empty), Empty))
> search 17 bt5;;
val it : bool = true
> search 4 bt5;;
val it : bool = false
> evenCount bt5;;
val it : int = 2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
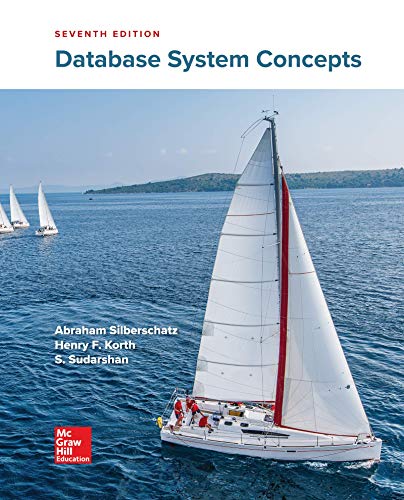
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
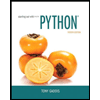
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
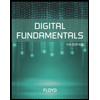
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
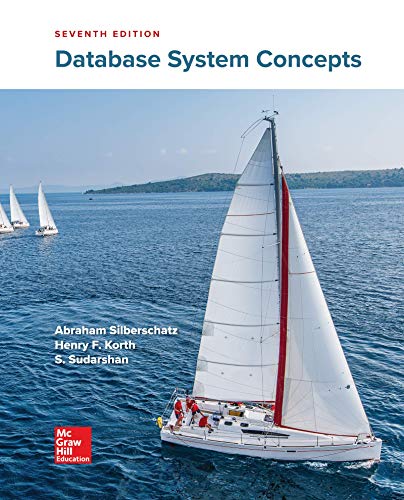
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
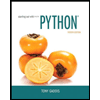
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
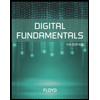
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
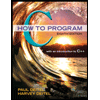
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
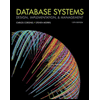
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
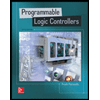
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education