Part 1: Text Surgeon Problem statement: You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters) from the user, store it as a C-style string. Then the program gives the user several options for operations to perform on the text or quit. After performing an operation, the user should be prompted to select another option to operate on the original string until they select the option to quit. The program must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for each choice are listed below: 1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in the text with the second letter. Example: User enters "off we go, into the wild blue yonder!", then characters 'o' and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder! (Note: capitalization is preserved) 2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with exactly the size of the user's input string (not the max length 1023!), and then reverse it. Remember to clean up your heap! Example: User enters "ban ana54!"; program creates a new string and outputs: "!45ana nab" Example: User enters "zip"; program creates a new string and outputs: "piz" 3. Words frequency: Prompt the user for an integer N, which represents for N words that the user wants to search for in the input string. Then prompt the user for these N words. Use a dynamic C++ string array allocated on the heap to store N words and output the frequency of each given words in the input string. Example: User enters "This is a test sentence, is this?", then 3 words, "this", "text", and "test". The program outputs: this: 2 text: 0 test: 1 (Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special characters in the string are ignored, which means "wor32rd", "wo]]]rd343.34", and "word" are the same) Suggested functions: a. char * purge_string (char *str); llaccepts a c-string, and returns a version where all numbers, spaces, and special characters removed (Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once we leave this function) OR you may use this:
Part 1: Text Surgeon Problem statement: You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters) from the user, store it as a C-style string. Then the program gives the user several options for operations to perform on the text or quit. After performing an operation, the user should be prompted to select another option to operate on the original string until they select the option to quit. The program must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for each choice are listed below: 1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in the text with the second letter. Example: User enters "off we go, into the wild blue yonder!", then characters 'o' and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder! (Note: capitalization is preserved) 2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with exactly the size of the user's input string (not the max length 1023!), and then reverse it. Remember to clean up your heap! Example: User enters "ban ana54!"; program creates a new string and outputs: "!45ana nab" Example: User enters "zip"; program creates a new string and outputs: "piz" 3. Words frequency: Prompt the user for an integer N, which represents for N words that the user wants to search for in the input string. Then prompt the user for these N words. Use a dynamic C++ string array allocated on the heap to store N words and output the frequency of each given words in the input string. Example: User enters "This is a test sentence, is this?", then 3 words, "this", "text", and "test". The program outputs: this: 2 text: 0 test: 1 (Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special characters in the string are ignored, which means "wor32rd", "wo]]]rd343.34", and "word" are the same) Suggested functions: a. char * purge_string (char *str); llaccepts a c-string, and returns a version where all numbers, spaces, and special characters removed (Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once we leave this function) OR you may use this:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Allowed libraries:
![Part 1: Text Surgeon
Problem statement:
You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters)
from the user, store it as a C-style string. Then the program gives the user several options for
operations to perform on the text or quit. After performing an operation, the user should be prompted to
select another option to operate on the original string until they select the option to quit. The program
must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for
each choice are listed below:
1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in
the text with the second letter.
Example: User enters "Off we go, into the wild blue yonder!", then characters 'o'
and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder!
(Note: capitalization is preserved)
2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with
exactly the size of the user's input string (not the max length 1023!), and then reverse it.
Remember to clean up your heap!
Example: User enters "ban ana54!"; program creates a new string and outputs: "!45ana
nab"
Example: User enters "zip"; program creates a new string and outputs: "piz"
3. Words frequency: Prompt the user for an integer N, which represents for N words that the user
wants to search for in the input string. Then prompt the user for these N words. Use a dynamic
C++ string array allocated on the heap to store N words and output the frequency of each given
words in the input string.
Example: User enters “This is a test sentence, is this?", then 3 words, “this",
"text", and "test". The program outputs:
this:
2
text: 0
test: 1
(Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special
characters in the string are ignored, which means "wor32rd", "wo]]rd343.34", and "word" are the
same)
Suggested functions:
a. char * purge_string (char *str); llaccepts a c-string, and returns a version where
all numbers, spaces, and special characters removed
(Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once
we leave this function)
OR you may use this:
void purge_string (char *str, char* str_new); llaccepts two c-strings, remove
all spaces and special characters in str, and store the new string into str_new
*Important note:
You are not allowed to convert a C-style string to a C++ string object. In other words, you have to treat
the input sentence (paragraph) as a Č-style string and parse through it.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8759b09c-a99c-4616-917a-1eb84cf5071d%2F47262640-5160-414b-a55d-89690c1ce2cd%2F0jehzvt_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part 1: Text Surgeon
Problem statement:
You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters)
from the user, store it as a C-style string. Then the program gives the user several options for
operations to perform on the text or quit. After performing an operation, the user should be prompted to
select another option to operate on the original string until they select the option to quit. The program
must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for
each choice are listed below:
1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in
the text with the second letter.
Example: User enters "Off we go, into the wild blue yonder!", then characters 'o'
and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder!
(Note: capitalization is preserved)
2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with
exactly the size of the user's input string (not the max length 1023!), and then reverse it.
Remember to clean up your heap!
Example: User enters "ban ana54!"; program creates a new string and outputs: "!45ana
nab"
Example: User enters "zip"; program creates a new string and outputs: "piz"
3. Words frequency: Prompt the user for an integer N, which represents for N words that the user
wants to search for in the input string. Then prompt the user for these N words. Use a dynamic
C++ string array allocated on the heap to store N words and output the frequency of each given
words in the input string.
Example: User enters “This is a test sentence, is this?", then 3 words, “this",
"text", and "test". The program outputs:
this:
2
text: 0
test: 1
(Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special
characters in the string are ignored, which means "wor32rd", "wo]]rd343.34", and "word" are the
same)
Suggested functions:
a. char * purge_string (char *str); llaccepts a c-string, and returns a version where
all numbers, spaces, and special characters removed
(Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once
we leave this function)
OR you may use this:
void purge_string (char *str, char* str_new); llaccepts two c-strings, remove
all spaces and special characters in str, and store the new string into str_new
*Important note:
You are not allowed to convert a C-style string to a C++ string object. In other words, you have to treat
the input sentence (paragraph) as a Č-style string and parse through it.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
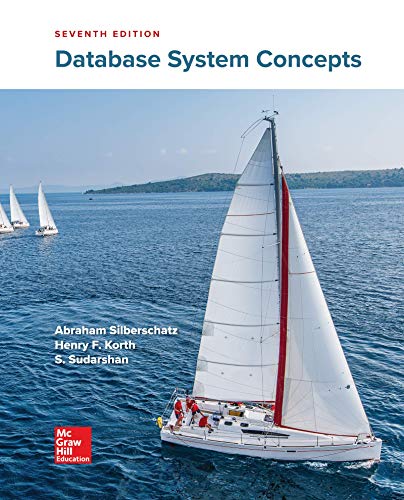
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
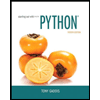
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
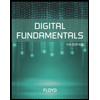
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
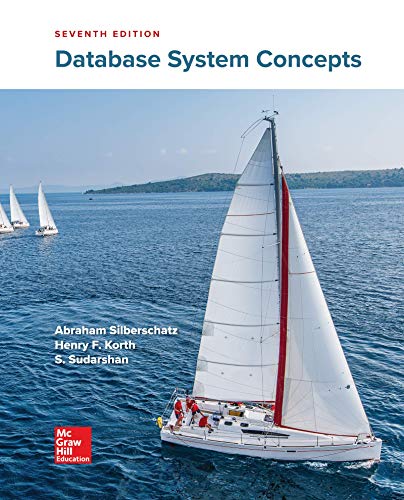
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
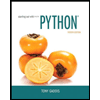
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
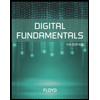
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
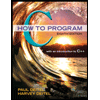
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
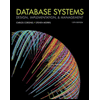
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
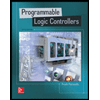
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education