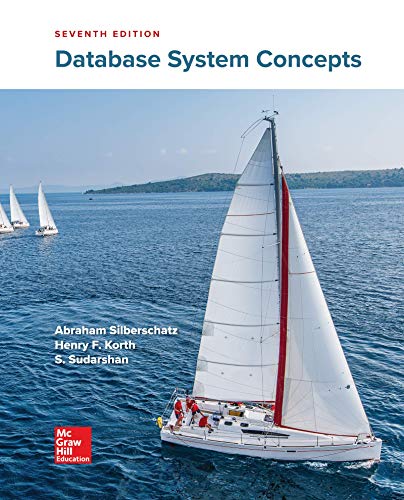
Part 1: Thread creation (30%)
a. Using Java multithreading library, write a Java program that calculates the sum of the numbers
from 1 to 100,000,000. Split the numbers between four threads equally where each thread
calculates the sum of one fourth of the numbers. For example, the 1st thread will calculate the sum
of the numbers from 1 to 25,000,000 whereas the 2nd thread will calculate the sum of the numbers
from 25,000,001 to 50,000,000 and so forth. The main thread will have to print out the sum after
gathering the results. Note that you have the choice to create threads by either implementing
Runnable interface or extending Thread class.
b. Now, write a sequential version of the program described above using a single main thread (i.e.,
without multithreading). Make sure to record and print out the time spent during the execution of
both sequential and multithreaded versions (hint: you may consider using
System.currentTimeMillis() to record execution time).
Part 2: Synchronization (40%)
Assume that we have a file named “sharable.txt” which can be shared among several threads. We
want to write a program that controls the access to that file in a way that only one thread at a time
is allowed to access it (i.e., for writing/appending purposes). Using Java multithreading, write a
program that creates three threads and assigns a number to each thread. Then, each thread will start
running by executing a code for opening the file “sharable.txt” and writing the following lines:
Thread x started writing
Thread x is currently writing
Thread x finished writing – Student Name
Your program should allow only one thread -at any given time-to access the file and write in it. It
also should keep away any thread from overwriting the lines written by any other threads (hint:
use synchronized methods/blocks). Finally, when the execution of your program is completed, the
output stored in “sharable.txt” should look like the following – put your name in place “Your
Name”:
Thread x started writing
Thread x is currently writing
Thread x finished writing – Your Name
Thread y started writing
Thread y is currently writing
Thread y finished writing – Your Name
Thread z started writing
Thread z is currently writing
Thread z finished writing – Your Name
Part 3: Interrupt handling (30%)
In Java multithreading environment, one thread can send an interrupt to another by calling the
interrupt() method on the Thread object for the target thread (i.e., the thread to be
interrupted). To handle interrupts in a target thread, Java allows two approaches. One is performed
by writing an exception handler for InterruptedException (only applicable if the target
thread is invoking methods which throw that exception such as sleep). The other approach is
performed by periodically checking the interrupt status flag Thread.interrupted and
performing the handling routine when that flag is set to true.
Write a Java program that illustrates the use of the two approaches described above. Your program
should start by creating two threads, each thread should use different interrupt handling approach.
Then, the program needs to send interrupts to each one of the created threads such that a thread
needs to return (i.e., stop execution) after receiving an interrupt from the main thread. Make sure
to output (print out) the status of each thread before and after being interrupted.
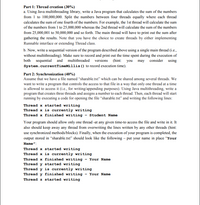
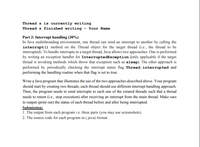

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- calculate the number of memory bytes accessed by this program: void my_dgemv(int n, double* A, double* x, double* y) { #pragma omp parallel { int nthreads = omp_get_num_threads(); int thread_id = omp_get_thread_num(); printf("Hello world: thread %d of %d checking in. \n", thread_id, nthreads); } // insert your dgemv code here. you may need to create additional parallel regions, // and you may want to comment out the above parallel code block that prints out // nthreads and thread_id so as to not taint your timings #pragma omp parallel for // insert your code here: implementation of basic matrix multiply for(int i = 0; i < n; i++) { #pragma omp parallel for for(int j = 0; j < n; j++) { y[i] += A[i * n + j] * x[j]; } } }arrow_forwardProblem 1: Thread creation (50%) a. Using Java multithreading library, write a Java program that calculates the sum of the numbers from 1 to 100,000,000. Split the numbers between four threads equally where each thread calculates the sum of one fourth of the numbers. For example, the 1st thread will calculate the sum of the numbers from 1 to 25,000,000 whereas the 2nd thread will calculate the sum of the numbers from 25,000,001 to 50,000,000 and so forth. The main thread will have to print out the sum after gathering the results. Note that you have the choice to create threads by either implementing Runnable interface or extending Thread class. b. Now, write a sequential version of the program described above using a single main thread (i.e., without multithreading). Make sure to record and print out the time spent during the execution of both sequential and multithreaded versions (hint: you may consider using System.currentTimeMillis() to record execution time).arrow_forwardJAVA PROGRAMMING You are required to create a main class to apply ExecuterService with SingleThreadExecuter. The variable i is integer number and MUST be input from the keyboard. Output should be like this: Please input i: 3 pool-1-thread-1: 1 pool-3-thread-1: 1 pool-3-thread-1: 2 pool-3-thread-1: 3 pool-2-thread-1: 1 pool-2-thread-1: 2 pool-2-thread-1: 3 pool-1-thread-1: 2 pool-1-thread-1: 3 Total = 9Below is the part of code.class Counter public class Counter { private int counter; public void increment(){ counter++; } public void decrement(){ counter--; } synchronized public void myIncrement(){ counter++; } synchronized public void myDecrement(){ counter--; } }arrow_forward
- USE PYTHON MULTI THREADING TO COMPLETE DO NOT USE PROCESSES ONLY COMPLETE PART 2 using MULTI THREADING 1) Basic version with two levels of threads (master and slaves) One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please program to generate 1000 random integers to populate the array). ************ONLY COMPLETE THIS PART BELOW*************** 2) Advanced version with more than two levels of threadsThe master thread creates two slave-threads where each slave-thread is responsible to sum half segment of the array. Each slave thread will fork/spawn two new slave-threads where each new slave-threadsums half of the array segment received by its parent. Each slave thread will return the subtotal to its parent thread and the parent thread aggregates and returns the total to its parent thread. Start with 7 nodes thread tree, when you are comfortable, you can extend it to a full…arrow_forwardHello Can you please help me with this code because I am struggling how do to this, can you please help me this code has to be in C. Write a multithreaded program that calculates various statistical values for a list of numbers. This program will be passed a series of numbers on the command line and will then create three separate worker threads. One thread will determine the average of the numbers, the second will determine the maximum value, and the third will determine the minimum value. For example, suppose your program is passed the integers 90 81 78 95 79 72 85 The program will report The average value is 82 The minimum value is 72 The maximum value is 95 The variables representing the average, minimum, and maximum values will be stored globally. The worker threads will set these values, and the parent thread will output the values once the workers have exited. (We could obviously expand this program by creating additional threads that determine other statistical values, such as…arrow_forwardUSE SIMPLE PYTHON CODE TO COMPLETE Basic version with two levels of threads (master and slaves) One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please program to generate 1000 random integers to populate the array). The number of slave-threads is a parameter which the user can change. For example, if the user chooses 4 slave threads, each slave thread will sum 1000/4 = 250 numbers. If the user chooses 3 slave threads, the first two may each sum 333 numbers and the third slave threadsums the rest 334 numbers. 2) Advanced version with more than two levels of threadsThe master thread creates two slave-threads where each slave-thread is responsible to sum half segment of the array. Each slave thread will fork/spawn two new slave-threads where each new slave-threadsums half of the array segment received by its parent. Each slave thread will return the subtotal to its parent…arrow_forward
- Write java code to create a thread by (extending), theprogram create 3 thread that displaying “fatmah” and thenumber of thread that is running.Rewrite the above program by implementing the RunnableInterfacearrow_forwardModify this threading example to use, exclusively, multiprocessing, instead of threading. import threadingimport time class BankAccount(): def __init__(self, name, balance): self.name = name self.balance = balance def __str__(self): return self.name # These accounts are our shared resourcesaccount1 = BankAccount("account1", 100)account2 = BankAccount("account2", 0) class BankTransferThread(threading.Thread): def __init__(self, sender, receiver, amount): threading.Thread.__init__(self) self.sender = sender self.receiver = receiver self.amount = amount def run(self): sender_initial_balance = self.sender.balance sender_initial_balance -= self.amount # Inserting delay to allow switch between threads time.sleep(0.001) self.sender.balance = sender_initial_balance receiver_initial_balance = self.receiver.balance receiver_initial_balance += self.amount # Inserting delay to allow switch between threads time.sleep(0.001)…arrow_forwardComputer Science #include<cmath>#include<stdio.h>__global__voidprocess_kernel1(float *input1,float *input2,float *output,int datasize){int idx = threadIdx.x + blockIdx.x * blockDim.x;int idy = threadIdx.y + blockIdx.y * blockDim.y;int idz = threadIdx.z + blockIdx.z * blockDim.z;int index = idz * (gridDim.x * blockDim.x) * (gridDim.y*blockDim.y) + idy * (gridDim.x * blockDim.x) +idx;if(index<datasize)output[index] = sinf(input1[index]) + cosf(input2[index]);}__global__voidprocess_kernel2(float *input,float *output,int datasize){int idx = threadIdx.x + blockIdx.x * blockDim.x;int idy = threadIdx.y + blockIdx.y * blockDim.y;int idz = threadIdx.z + blockIdx.z * blockDim.z;int index = idz * (gridDim.x * blockDim.x) * (gridDim.y*blockDim.y) + idy * (gridDim.x * blockDim.x) +idx;if(index<datasize)output[index] = logf(input[index]);}_global__voidprocess_kernel3(float *input,float *output,int datasize){int idx = threadIdx.x + blockIdx.x *…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
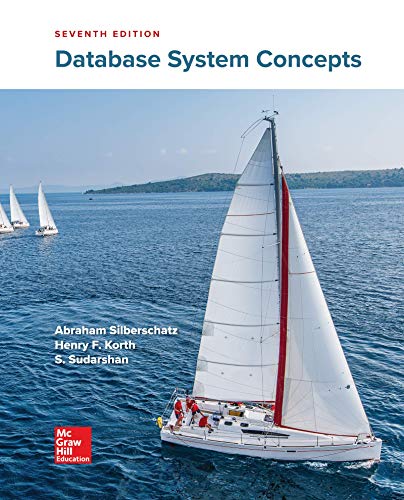
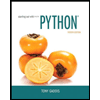
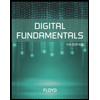
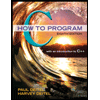
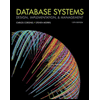
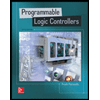