Part 1 You will implement the following three methods to find the GCD of two numbers. You may not change any of these method signatures. int gcd_iterative(int m, int n); int gcd_recursive(int m, int n); int main(int argc, char **argv); In both gcd_iterative and gcd_recursive, you will implement Euclid's method (feel free to search for the algorithm on Google). The iterative version will use a while loop, while the recursive will, of course, use recursion. The function header for gcd_iterative can be found in iterative.h and the function header for gcd_recursive can be found in recursive.h. The function definitions must be in iterative.c and recursive.c respectively (which you will create). The main method in gcd.c will parse the command line arguments and call both gcd_iterative and gcd_recursive. Don't forget that gcd (m, n) is equivalent to gcd (m, n) so you should never return a negative value. If more than or less than two command line arguments are provided, you should print the following error message to stderr then exit in failure. Usage: ./gcd If two command line arguments are provided, you may assume that they will be valid integers. If the two integers are 0, you should print the following message to stdout then exit in success. gcd(0, 0) = undefined Otherwise, your output should match the sample executions below. Then, you should exit in success. Your Makefile should generate an executable called gcd. Sample Executions mes (41 sloc) 2.6 KB Kecursive: gca(24, 12) = 12 $ ./gcd -2 10 Iterative: gcd (-2, 10) = 2 Recursive: gcd (-2, 10) = 2 $ ./gcd 0 0 gcd (0, 0) = undefined $ ./gcd 1 1 1 Usage: ./gcd <> Raw Blame Note that your program must behave EXACTLY the same as the sample executions above. This means that the strings, ordering, and spacing must be the same. Requirements/Tips • The only libraries you can include are stdio.h and stdlib.h. Any other libraries you include will be removed before we test your code. • gcd.c may not include any source files directly. (i.e. you cannot do #include "recursive.c") You may use the abs library function to find the absolute value of an int. Use man 3 abs for more information. You will need to use atoi to convert from char * to int. Use man 3 atoi for more information. • Use the EXIT_SUCCESS and EXIT_FAILURE macros for returning from the main function of gcd.c. • You are required to add header guards (conditional compilation directives) to each header file. (recursive.h and iterative.h).
Part 1 You will implement the following three methods to find the GCD of two numbers. You may not change any of these method signatures. int gcd_iterative(int m, int n); int gcd_recursive(int m, int n); int main(int argc, char **argv); In both gcd_iterative and gcd_recursive, you will implement Euclid's method (feel free to search for the algorithm on Google). The iterative version will use a while loop, while the recursive will, of course, use recursion. The function header for gcd_iterative can be found in iterative.h and the function header for gcd_recursive can be found in recursive.h. The function definitions must be in iterative.c and recursive.c respectively (which you will create). The main method in gcd.c will parse the command line arguments and call both gcd_iterative and gcd_recursive. Don't forget that gcd (m, n) is equivalent to gcd (m, n) so you should never return a negative value. If more than or less than two command line arguments are provided, you should print the following error message to stderr then exit in failure. Usage: ./gcd If two command line arguments are provided, you may assume that they will be valid integers. If the two integers are 0, you should print the following message to stdout then exit in success. gcd(0, 0) = undefined Otherwise, your output should match the sample executions below. Then, you should exit in success. Your Makefile should generate an executable called gcd. Sample Executions mes (41 sloc) 2.6 KB Kecursive: gca(24, 12) = 12 $ ./gcd -2 10 Iterative: gcd (-2, 10) = 2 Recursive: gcd (-2, 10) = 2 $ ./gcd 0 0 gcd (0, 0) = undefined $ ./gcd 1 1 1 Usage: ./gcd <> Raw Blame Note that your program must behave EXACTLY the same as the sample executions above. This means that the strings, ordering, and spacing must be the same. Requirements/Tips • The only libraries you can include are stdio.h and stdlib.h. Any other libraries you include will be removed before we test your code. • gcd.c may not include any source files directly. (i.e. you cannot do #include "recursive.c") You may use the abs library function to find the absolute value of an int. Use man 3 abs for more information. You will need to use atoi to convert from char * to int. Use man 3 atoi for more information. • Use the EXIT_SUCCESS and EXIT_FAILURE macros for returning from the main function of gcd.c. • You are required to add header guards (conditional compilation directives) to each header file. (recursive.h and iterative.h).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need Help with this C
![Part 1
You will implement the following three methods to find the GCD of two numbers. You may not change any of these method
signatures.
int gcd_iterative(int m, int n);
int gcd_recursive(int m, int n);
int main(int argc, char **argv);
In both gcd_iterative and gcd_recursive, you will implement Euclid's method (feel free to search for the algorithm on Google). The
iterative version will use a while loop, while the recursive will, of course, use recursion.
The function header for gcd_iterative can be found in iterative.h and the function header for gcd_recursive can be found in
recursive.h. The function definitions must be in iterative.c and recursive.c respectively (which you will create).
The main method in gcd.c will parse the command line arguments and call both gcd_iterative and gcd_recursive. Don't forget
that gcd(m, n) is equivalent to gcd ([m], [n]) so you should never return a negative value.
If more than or less than two command line arguments are provided, you should print the following error message to stderr then exit
in failure.
Usage: ./gcd <integer m> <integer n>
If two command line arguments are provided, you may assume that they will be valid integers. If the two integers are 0, you should
print the following message to stdout then exit in success.
gcd (0, 0) = undefined
Otherwise, your output should match the sample executions below. Then, you should exit in success.
Your Makefile should generate an executable called gcd .
Sample Executions
nes (41 sloc)
Recursive: gca(24, 12) = 12
$ ./gcd -2 10
2.6 KB
Iterative: gcd (-2, 10) = 2
Recursive: gcd (-2, 10) = 2
$ ./gcd 0 0
gcd(0, 0) = undefined
$ ./gcd 1 1 1
Usage: ./gcd <integer m> <integer n>
<>
U
Raw
• gcd.c may not include any source files directly. (i.e. you cannot do #include "recursive.c")
• You may use the abs library function to find the absolute value of an int. Use man 3 abs for more information.
• You will need to use atoi to convert from char * to int. Use man 3 atoi for more information.
Blame
Note that your program must behave EXACTLY the same as the sample executions above. This means that the strings, ordering, and
spacing must be the same.
Requirements/Tips
The only libraries you can include are stdio.h and stdlib.h. Any other libraries you include will be removed before we test your
code.
• Use the EXIT_SUCCESS and EXIT_FAILURE macros for returning from the main function of gcd.c.
• You are required to add header guards (conditional compilation directives) to each header file. (recursive.h and iterative.h).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F925db09b-487b-4703-a279-315e508d629e%2Fdeec588c-85d5-4b7a-932d-97fecdc8589c%2Fxo6ehkg_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part 1
You will implement the following three methods to find the GCD of two numbers. You may not change any of these method
signatures.
int gcd_iterative(int m, int n);
int gcd_recursive(int m, int n);
int main(int argc, char **argv);
In both gcd_iterative and gcd_recursive, you will implement Euclid's method (feel free to search for the algorithm on Google). The
iterative version will use a while loop, while the recursive will, of course, use recursion.
The function header for gcd_iterative can be found in iterative.h and the function header for gcd_recursive can be found in
recursive.h. The function definitions must be in iterative.c and recursive.c respectively (which you will create).
The main method in gcd.c will parse the command line arguments and call both gcd_iterative and gcd_recursive. Don't forget
that gcd(m, n) is equivalent to gcd ([m], [n]) so you should never return a negative value.
If more than or less than two command line arguments are provided, you should print the following error message to stderr then exit
in failure.
Usage: ./gcd <integer m> <integer n>
If two command line arguments are provided, you may assume that they will be valid integers. If the two integers are 0, you should
print the following message to stdout then exit in success.
gcd (0, 0) = undefined
Otherwise, your output should match the sample executions below. Then, you should exit in success.
Your Makefile should generate an executable called gcd .
Sample Executions
nes (41 sloc)
Recursive: gca(24, 12) = 12
$ ./gcd -2 10
2.6 KB
Iterative: gcd (-2, 10) = 2
Recursive: gcd (-2, 10) = 2
$ ./gcd 0 0
gcd(0, 0) = undefined
$ ./gcd 1 1 1
Usage: ./gcd <integer m> <integer n>
<>
U
Raw
• gcd.c may not include any source files directly. (i.e. you cannot do #include "recursive.c")
• You may use the abs library function to find the absolute value of an int. Use man 3 abs for more information.
• You will need to use atoi to convert from char * to int. Use man 3 atoi for more information.
Blame
Note that your program must behave EXACTLY the same as the sample executions above. This means that the strings, ordering, and
spacing must be the same.
Requirements/Tips
The only libraries you can include are stdio.h and stdlib.h. Any other libraries you include will be removed before we test your
code.
• Use the EXIT_SUCCESS and EXIT_FAILURE macros for returning from the main function of gcd.c.
• You are required to add header guards (conditional compilation directives) to each header file. (recursive.h and iterative.h).
Expert Solution

Step 1
Introduction
Euclid GCD:
Euclid's GCD (Greatest Common Divisor) is an algorithm used to determine the greatest common divisor of two numbers. It is based on the property that the GCD of two numbers is the same as the GCD of the smaller number and the remainder of the larger number divided by the smaller number. The algorithm repeatedly applies this property until the smaller number becomes 0, at which point the GCD of the two numbers is the last non-zero remainder. This algorithm was first described by the ancient Greek mathematician Euclid and is one of the oldest algorithms still in use.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
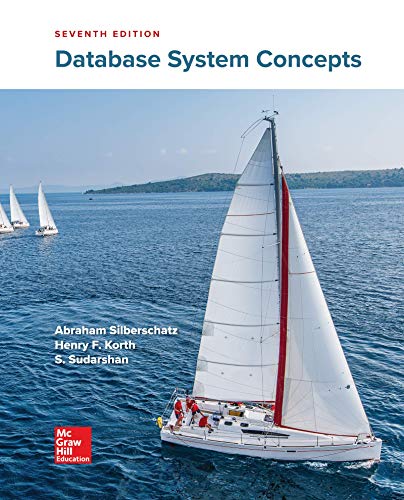
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
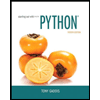
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
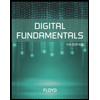
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
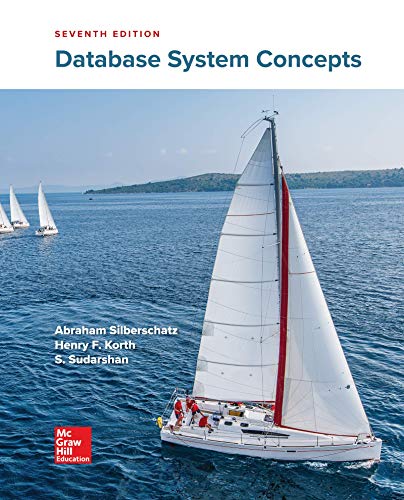
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
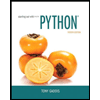
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
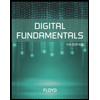
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
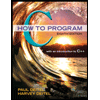
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
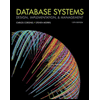
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
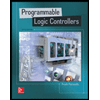
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education