JAVA Question 2: For two integers m and n, their GCD (Greatest Common Divisor) can be computed by a recursive method. Write a recursive method gcd(m,n) to find their Greatest Common Divisor. Method body: If m is 0, the method returns n. If n is 0, the method returns m. If neither is 0, the method can recursively calculate the Greatest Common Divisor with two smaller parameters: One is n, the second one is m mod n (or m % n). The recursive method cannot have loops. Note: although there are other approaches to calculate Greatest Common Divisor, please follow the instructions in this question, otherwise you will not get the credit. main method: Prompt and read in two numbers to find the greatest common divisor. Call the gcd method with the two numbers as its argument. Print the result to the monitor. Example program run: Enter m: 12 Enter n: 28 GCD(12,28) = 4 And here is what I have so far, package CSCI1302; import java.util.*; public class RecursionDemo { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Please enter a start number."); int start = scan.nextInt(); System.out.println("Please enter an end number."); int end = scan.nextInt(); System.out.println(addStartEnd(start, end)); // Question 2 System.out.println("Please enter two new numbers to find the gcd."); gcd(0,0); } public static int addStartEnd(int start, int end){ if(start == end) return start; else { return start + addStartEnd(start + 1, end); } }// Question 2 public static int gcd(int m, int n) { if(m == 0); return n; else { System.out.println(n); } } } What am I doing wrong here and how do I fix it? Java please
JAVA
Question 2:
For two integers m and n, their GCD (Greatest Common Divisor) can be computed by a recursive method. Write a recursive method gcd(m,n) to find their Greatest Common Divisor.
Method body:
If m is 0, the method returns n. If n is 0, the method returns m. If neither is 0, the method can recursively calculate the Greatest Common Divisor with two smaller parameters: One is n, the second one is m mod n (or m % n). The recursive method cannot have loops.
Note: although there are other approaches to calculate Greatest Common Divisor, please follow the instructions in this question, otherwise you will not get the credit.
main method:
Prompt and read in two numbers to find the greatest common divisor.
Call the gcd method with the two numbers as its argument. Print the result to the monitor.
Example program run:
Enter m:
12
Enter n:
28
GCD(12,28) = 4
And here is what I have so far,
package CSCI1302;
import java.util.*;
public class RecursionDemo {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.println("Please enter a start number.");
int start = scan.nextInt();
System.out.println("Please enter an end number.");
int end = scan.nextInt();
System.out.println(addStartEnd(start, end));
// Question 2
System.out.println("Please enter two new numbers to find the gcd.");
gcd(0,0);
}
public static int addStartEnd(int start, int end){
if(start == end)
return start;
else {
return start + addStartEnd(start + 1, end);
}
}// Question 2
public static int gcd(int m, int n) {
if(m == 0);
return n;
else {
System.out.println(n);
}
}
}
What am I doing wrong here and how do I fix it? Java please

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

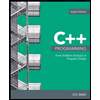
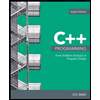