PART 1. This exercise will have the user enter some information about a movie. 1. Create an enumerated type (enum) that represents movie ratings: G, PG, PG_13, R, NC_17 (Remember enum names must following C++ variable name rules, so no dashes (-) allowed) 2. Create a STRUCTURE with the following information: move name movie rating DEFINE this as the TYPE of the enum created in step 1. year produced main star of the movie, i.e., person value indicating whether the user saw the movie. Can be a char, int, etc. storing a Y or N type value value indicating whether the user liked the movie. Can be a char, int, etc. storing a Y or N type value 3. Request the above information from the user and store in the structure. The rating may be requested as follows: 1 - G 2 - PG 3 - PG-13 4 - R 5 - NC-17 NOTE: The following may come in handy: structureVariable.move_ratingVariable = enumType(rating value entered) Where structureVariable is the variable name of the enum type move_ratingVariable is the variable name in the structure for move rating enumType is the name of the defined enum rating value entered is the variable containing the rating value the user entered Try the above before asking!!! 4. Output the information the user entered from the data stored in the STRUCTURE. The move name, the year, the main star Output the rating using the enum as follows: if G - This movie is rated G: General Audiences if PG - This movie is rated PG : Parental Guidance Suggested if PG_13 - This movie is rated PG-13: Parent's Strongly Cautioned if R - This movie is rated R: Under 17 requires accompanying adult or adult guardian if NC_17 - This movie is rated NC-17: No one under 17 and under admitted If the user saw the movie output You saw the movie; else output You did not see the movie. If the user liked the movie, output You Liked the Move; else output You did not like the movie. PART 2 Read the following carefully to get full value from the practice. This exercise will have you extend the lab performed in part I. Using the code in part I of this lab: 1. Create a function to INPUT the information for the movie. Pass the empty structure in as a parameter. 2. Create a function to OUTPUT the information in the structure. Pass the filled structure in as a parameter. 3. Place the ENUM definition, STRUCTURE definition, and FUNCTION prototypes in a HEADER FILE (*.h). Remove those definitions from the main program and include your header file in the main program file. DECLARE the STRUCTURE VARIABLE as a LOCAL (not global) variable in the ma
PART 1.
This exercise will have the user enter some information about a movie.
1. Create an enumerated type (enum) that represents movie ratings:
G, PG, PG_13, R, NC_17
(Remember enum names must following C++ variable name rules, so no dashes (-) allowed)
2. Create a STRUCTURE with the following information:
move name
movie rating DEFINE this as the TYPE of the enum created in step 1.
year produced
main star of the movie, i.e., person
value indicating whether the user saw the movie. Can be a char, int, etc.
storing a Y or N type value
value indicating whether the user liked the movie. Can be a char, int, etc.
storing a Y or N type value
3. Request the above information from the user and store in the structure. The
rating may be requested as follows:
1 - G
2 - PG
3 - PG-13
4 - R
5 - NC-17
NOTE: The following may come in handy: structureVariable.move_ratingVariable = enumType(rating value entered)
Where structureVariable is the variable name of the enum type
move_ratingVariable is the variable name in the structure for move rating
enumType is the name of the defined enum
rating value entered is the variable containing the rating value the user entered
Try the above before asking!!!
4. Output the information the user entered from the data stored in the STRUCTURE.
The move name, the year, the main star
Output the rating using the enum as follows:
if G - This movie is rated G: General Audiences
if PG - This movie is rated PG : Parental Guidance Suggested
if PG_13 - This movie is rated PG-13: Parent's Strongly Cautioned
if R - This movie is rated R: Under 17 requires accompanying adult or adult guardian
if NC_17 - This movie is rated NC-17: No one under 17 and under admitted
If the user saw the movie output You saw the movie; else output You did not see the movie.
If the user liked the movie, output You Liked the Move; else output You did not like the movie.
PART 2
Read the following carefully to get full value from the practice.
This exercise will have you extend the lab performed in part I. Using the code in part I of
this lab:
1. Create a function to INPUT the information for the movie. Pass the empty structure in
as a parameter.
2. Create a function to OUTPUT the information in the structure. Pass the filled structure
in as a parameter.
3. Place the ENUM definition, STRUCTURE definition, and FUNCTION prototypes in a HEADER FILE (*.h).
Remove those definitions from the main program and include your header file in the main program
file. DECLARE the STRUCTURE VARIABLE as a LOCAL (not global) variable in the main program.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

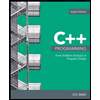
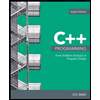