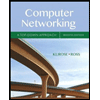
struct namerec{
char last[15]; char first[15]; char middle[15];
};
struct payrecord{
int id; struct namerec name; float hours, rate; float regular, overtime;
float gross, tax_withheld, net;
};
Given the above declaration, let payroll data record be stored in a structure called payrecord. Also define a type called payrecord for the structure data type that houses a payroll data record:
typedef struct payrecord payrecord;
This program reads data, computes payroll and prints it. Each data record is a structure, and the payroll is an array of structures. Overtime hours are 150% of the rate. (Note: Maximum regular hours for the week is 40.) Tax is withheld 15% if weekly pay is below 500, 28% if pay is below 1000, and 33% otherwise. A summary report prints the total gross pay and tax withheld.
The following are the function prototypes:
void readName(payrecord payroll[], int i); - reads a single name.
void printName(payrecord payroll[], int i); - prints a single name.
void printSummary(double gross, double tax);
- prints total tax gross pay and total tax withheld.
void readRecords(payrecord payroll[], int n);
- reads payroll input data records until n records have been read.
void printRecords(payrecord payroll[], int n); - prints n payroll records.
double calcRecords(payrecord payroll[], int n, double *taxptr);
- computes regular and overtime pay, and the tax to be withheld.
- also cumulatively sums total gross pay and total tax withheld.
- passes the address of tax initialized to 0 and returns the gross.
![struct namerec{
char last[15];
char first[15]; char
middle[15];
};
struct payrecord{
int id; struct
namerec name;
float
hours, rate;
float regular,
overtime;
float gross, tax_withheld, net;
};
Given the above declaration, let payroll data record be stored in a structure called payrecord.
Also define a type called payrecord for the structure data type that houses a payroll data record:
typedef struct payrecord payrecord;
This program reads data, computes payroll and prints it. Each data record is a structure, and the
payroll is an array of structures. Overtime hours are 150% of the rate. (Note: Maximum regular
hours for the week is 40.) Tax is withheld 15% if weekly pay is below 500, 28% if pay is below 1000,
and 33% otherwise. A summary report prints the total gross pay and tax withheld.
The following are the function prototypes:
void readName(payrecord payroll[], int i);
- reads a single name.
void printName(payrecord payroll[], int i);
- prints a single name.
void printSummary(double gross, double tax);
prints total tax gross pay and total tax withheld.
void readRecords(payrecord payroll[], int n);
- reads payroll input data records until n records have been read.
void printRecords(payrecord payroll[], int n);
- prints n payroll records.
double calcRecords(payrecord payroll), int n, double *taxptr);
- computes regular and overtime pay, and the tax to be withheld.
also cumulatively sums total gross pay and total tax withheld.
passes the address of tax initialized to 0 and returns the gross.](https://content.bartleby.com/qna-images/question/c4a34d12-0d6c-4be0-9193-6f0de1d04b68/f92cee0f-0de7-4470-a65e-7fd26a726bf7/o0hjh2_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- #include <iostream>using namespace std; struct Person{ int weight ; int height;}; int main(){ Person fred; Person* ptr = &fred; fred.weight=190; fred.height=70; return 0;} What is a correct way to subtract 5 from fred's weight using the pointer variable 'ptr'?arrow_forwardThe following struct types have been defined: typedef struct { int legs; char *sound; char *name; } pet_t; typedef struct { char *name; int age; pet_t *pet; } owner_t; A variable kid has been defined by: owner_t *kid; Functions get_owner and get_pet have the prototypes: owner_t *get_owner(char *,int,pet_t *); pet_t *get_pet (char *name, char *sound, int legs); Using kid, print a line like: Julie's pet parrot has 2 legs and says, "squawk".arrow_forwardC# helparrow_forward
- Define a struct type that represents an exercise plan. The struct should store the following data: plan name (a character array of size 50) and goal calories to burn (an integer). The type should be renamed from struct exercise_plan to Exercise_plan.arrow_forwardIn C++ struct applianceType { string supplier; string modelNo; double cost; }; applianceType myNewAppliance; applianceType applianceList[25]; Write the C++ statements that prints out all the appliance info from applianceList whose modelNo is B1234.arrow_forward// NOTE: Two separate versions of the sequence (one for a sequence of real numbers and another for a sequence of characters are specified, in two separate namespaces in this header file. For both versions, the same documentation applies.// TYPEDEFS and MEMBER functions for the sequence class:// typedef ____ value_type// sequence::value_type is the data type of the items in the sequence.// It may be any of the C++ built-in types (int, char, etc.), or a// class with a default constructor, an assignment operator, and a// copy constructor.// typedef ____ size_type// sequence::size_type is the data type of any variable that keeps// track of how many items are in a sequence.// static const size_type CAPACITY = _____// sequence::CAPACITY is the maximum number of items that a// sequence can hold.//// CONSTRUCTOR for the sequence class:// sequence()// Pre: (none)// Post: The sequence has been initialized as an empty sequence.//// MODIFICATION…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
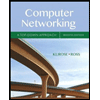
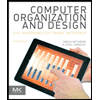
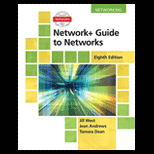
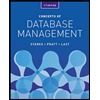
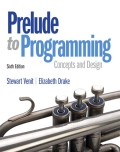
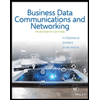