Part 6: Boolean Logic Expressions Boolean expressions are types of logical operations that we can perform on true and false values. Note that the word Boolean is always capitalized because it was named after its inventor, George Boole. Boolean algebra is a very important topic in computer science, and if you haven't learned it before you definitely will in the future. However, for our purposes we are only interested in how we can use them to manipulate binary numbers. The way we use them on binary numbers is by treating 1 as true and 0 as false. From this point on I will be using 0 and 1 instead of false and true. There are many types of Boolean expressions, but the three most important ones are AND, OR, and NOT. AND takes 2 operands and will output 1 if they are both 1, or 0 otherwise OR takes 2 operands and will output 1 if either one is 1, or 0 if neither is 1 NOT takes 1 operand and reverses it: 1 becomes 0, and 0 becomes 1 We can represent this behavior using something called a truth table to show all possible outcomes: AND First operand Second operand Result 0 0 0 0 1 0 1 0 0 1 1 1 OR First operand Second operand Result 0 0 0 0 1 1 1 0 1 1 1 1 NOT Operand Result 0 1 1 0 Exercise 12: What is 1 AND 0? We can also use these operations on larger binary numbers. The way we do so is the same as with other arithmetic: one column at a time. Unlike addition there is no chance of carried values, so we can perform Boolean operations from right-to-left or left-to-right and get the same result. For example, here is how we get the result of 1100 AND 1010: 1 1 0 0 AND 1 0 1 0 1 0 0 0 Leftmost column: 1 AND 1 = 1 Second column: 1 AND 0 = 0 Third column: 0 AND 1 = 0 Last column: 0 AND 0 = 0 The process is the same for OR: 1 1 0 0 OR 1 0 1 0 1 1 1 0 Leftmost column: 1 OR 1 = 1 Second column: 1 OR 0 = 1 Third column: 0 OR 1 = 1 Last column: 0 OR 0 = 0 For NOT, you just need to flip each bit: NOT 1 1 0 0 0 0 1 1 Exercise 13: What is 11101000 AND 01000001? Exercise 14: What is 11110000 OR 00001111? Exercise 15: Convert the number 128 to binary, then perform the NOT operation on it. What is the result?
Part 6: Boolean Logic Expressions
Boolean expressions are types of logical operations that we can perform on true and false values. Note that the word Boolean is always capitalized because it was named after its inventor, George Boole. Boolean algebra is a very important topic in computer science, and if you haven't learned it before you definitely will in the future. However, for our purposes we are only interested in how we can use them to manipulate binary numbers. The way we use them on binary numbers is by treating 1 as true and 0 as false. From this point on I will be using 0 and 1 instead of false and true.
There are many types of Boolean expressions, but the three most important ones are AND, OR, and NOT.
- AND takes 2 operands and will output 1 if they are both 1, or 0 otherwise
- OR takes 2 operands and will output 1 if either one is 1, or 0 if neither is 1
- NOT takes 1 operand and reverses it: 1 becomes 0, and 0 becomes 1
We can represent this behavior using something called a truth table to show all possible outcomes:
AND | ||
First operand | Second operand | Result |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
OR | ||
First operand | Second operand | Result |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
NOT | |
Operand | Result |
0 | 1 |
1 | 0 |
Exercise 12: What is 1 AND 0?
We can also use these operations on larger binary numbers. The way we do so is the same as with other arithmetic: one column at a time. Unlike addition there is no chance of carried values, so we can perform Boolean operations from right-to-left or left-to-right and get the same result.
For example, here is how we get the result of 1100 AND 1010:
1 1 0 0
AND 1 0 1 0
1 0 0 0
Leftmost column: 1 AND 1 = 1
Second column: 1 AND 0 = 0
Third column: 0 AND 1 = 0
Last column: 0 AND 0 = 0
The process is the same for OR:
1 1 0 0
OR 1 0 1 0
1 1 1 0
Leftmost column: 1 OR 1 = 1
Second column: 1 OR 0 = 1
Third column: 0 OR 1 = 1
Last column: 0 OR 0 = 0
For NOT, you just need to flip each bit:
NOT 1 1 0 0
0 0 1 1
Exercise 13: What is 11101000 AND 01000001?
Exercise 14: What is 11110000 OR 00001111?
Exercise 15: Convert the number 128 to binary, then perform the NOT operation on it. What is the result?

Step by step
Solved in 3 steps

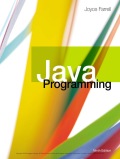
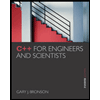
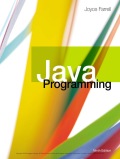
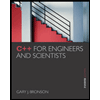