Part B: Strategy Pattern We've already seen a design pattern, the iterator pattern, that allowed us to iterate over any collection of items without knowing how the collection is actually implemented. In this part of the lab, we'll be looking at another design pattern called the Strategy Pattern. It involves applying a collection of methods (with an interface in common) to a list of objects. Create an interface like this: public interface OpClass { abstract Object op (Object arg); Next, create three classes Square, Cube, and SquareRoot that implement this interface. The Square should convert the arg to a Number, square it, then return the Number as an object. The Cube will do the same thing, expect it will cube the number. The same applies to the SquareRoot which returns the square root of the number. Create a method called apply in another public class called Calculator and implement your main method here. The apply method should accept an ArrayList of Numbers and an OpClass to apply to the list. It should return a new ArrayList with the new values in it. Make use of the ArrayList iterator here as well. Test this out by building a list of integers, and applying the square, cube, then square root objects on the list. Print out the list after each application.
Part B: Strategy Pattern We've already seen a design pattern, the iterator pattern, that allowed us to iterate over any collection of items without knowing how the collection is actually implemented. In this part of the lab, we'll be looking at another design pattern called the Strategy Pattern. It involves applying a collection of methods (with an interface in common) to a list of objects. Create an interface like this: public interface OpClass { abstract Object op (Object arg); Next, create three classes Square, Cube, and SquareRoot that implement this interface. The Square should convert the arg to a Number, square it, then return the Number as an object. The Cube will do the same thing, expect it will cube the number. The same applies to the SquareRoot which returns the square root of the number. Create a method called apply in another public class called Calculator and implement your main method here. The apply method should accept an ArrayList of Numbers and an OpClass to apply to the list. It should return a new ArrayList with the new values in it. Make use of the ArrayList iterator here as well. Test this out by building a list of integers, and applying the square, cube, then square root objects on the list. Print out the list after each application.
Chapter4: More Object Concepts
Section: Chapter Questions
Problem 10RQ
Related questions
Question
in Java
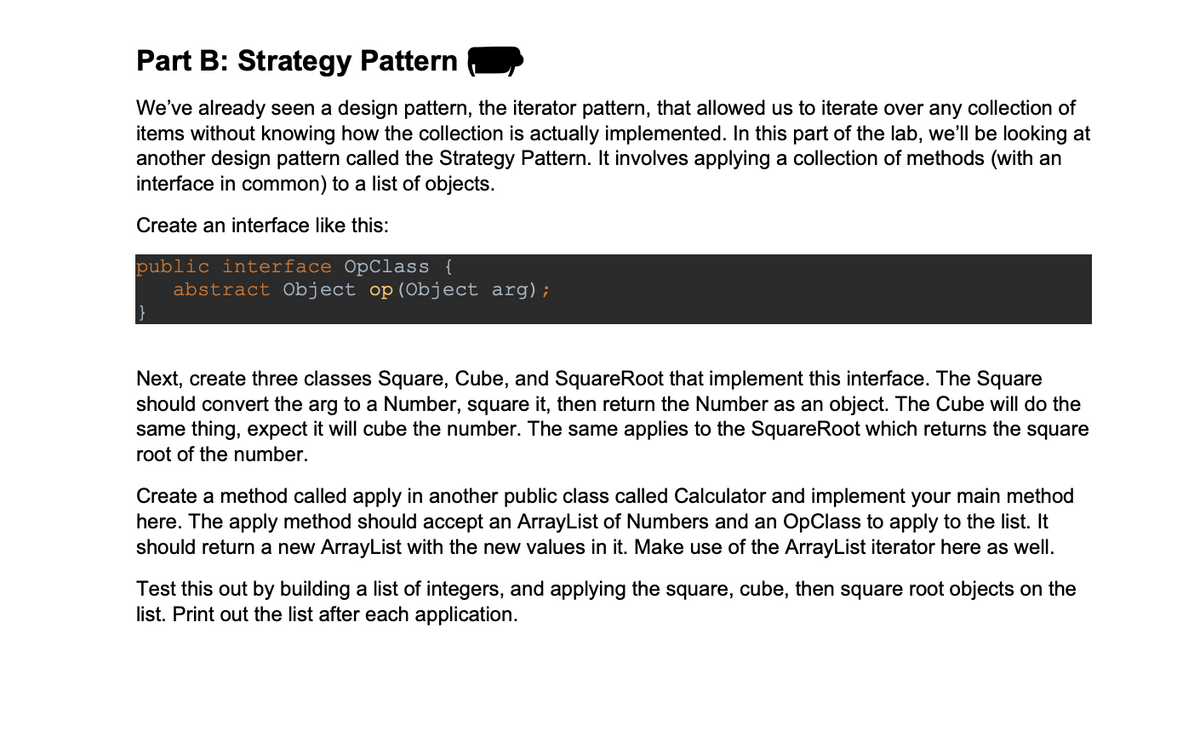
Transcribed Image Text:Part B: Strategy Pattern
We've already seen a design pattern, the iterator pattern, that allowed us to iterate over any collection of
items without knowing how the collection is actually implemented. In this part of the lab, we'll be looking at
another design pattern called the Strategy Pattern. It involves applying a collection of methods (with an
interface in common) to a list of objects.
Create an interface like this:
public interface OpClass {
abstract Object op (Object arg);
Next, create three classes Square, Cube, and SquareRoot that implement this interface. The Square
should convert the arg to a Number, square it, then return the Number as an object. The Cube will do the
same thing, expect it will cube the number. The same applies to the SquareRoot which returns the square
root of the number.
Create a method called apply in another public class called Calculator and implement your main method
here. The apply method should accept an ArrayList of Numbers and an OpClass to apply to the list. It
should return a new ArrayList with the new values in it. Make use of the ArrayList iterator here as well.
Test this out by building a list of integers, and applying the square, cube, then square root objects on the
list. Print out the list after each application.
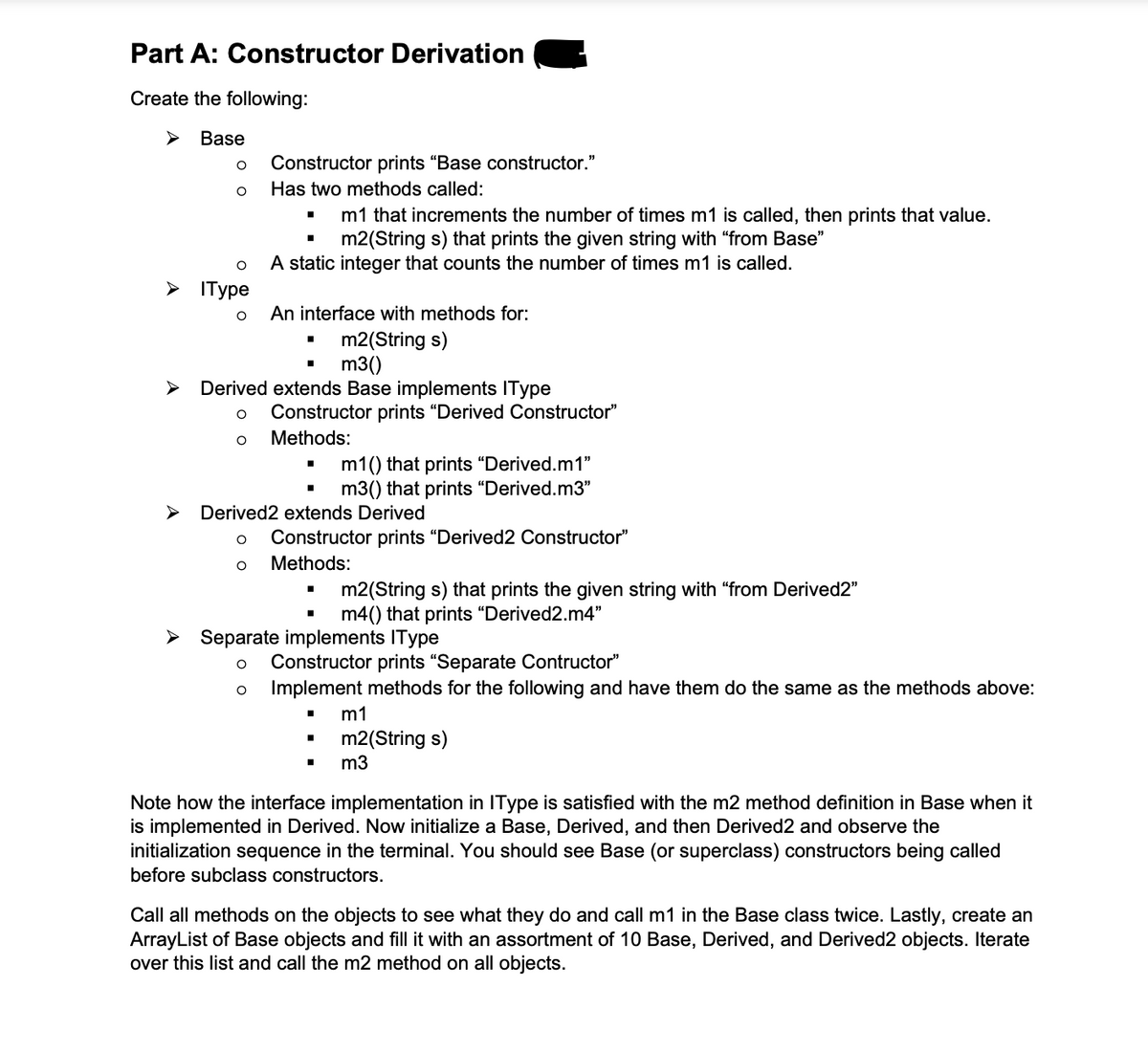
Transcribed Image Text:Part A: Constructor Derivation
Create the following:
<
Base
о
о
Constructor prints "Base constructor."
Has two methods called:
<
"
m1 that increments the number of times m1 is called, then prints that value.
m2(String s) that prints the given string with "from Base"
о
IType
о
"
A static integer that counts the number of times m1 is called.
An interface with methods for:
"
m2(String s)
m3()
Derived extends Base implements IType
о Constructor prints "Derived Constructor"
о
Methods:
m1() that prints "Derived.m1"
"
m3() that prints "Derived.m3"
Derived2 extends Derived
о
о
Constructor prints "Derived2 Constructor"
Methods:
■
m2(String s) that prints the given string with "from Derived2"
m4() that prints "Derived2.m4"
➤ Separate implements IType
о Constructor prints "Separate Contructor"
о Implement methods for the following and have them do the same as the methods above:
m1
m2(String s)
m3
Note how the interface implementation in IType is satisfied with the m2 method definition in Base when it
is implemented in Derived. Now initialize a Base, Derived, and then Derived2 and observe the
initialization sequence in the terminal. You should see Base (or superclass) constructors being called
before subclass constructors.
Call all methods on the objects to see what they do and call m1 in the Base class twice. Lastly, create an
ArrayList of Base objects and fill it with an assortment of 10 Base, Derived, and Derived2 objects. Iterate
over this list and call the m2 method on all objects.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
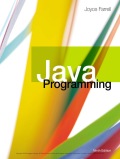
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
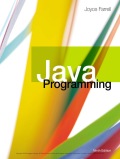
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT