# Pets ## Breed Class Create a `Breed` class with the following: ### Member Variables Create the following private member variables, all of type `std::string`: 1. `species_` 2. `breed_name_` 3. `color_` ### Constructors 1. Create a default constructor for `Breed` that sets its `species_` to `"Dog"`, `breed_name_` to `"Chihuahua"`, and `color_` to `"Fawn"`. 2. Create a non-default constructor that receives a `std::string` for `species_`, `breed_name_`, and `color_`; in that order. The values from the constructor should appropriately assign the member variables. ### Accessors and Mutators Create accessors and mutators for all member variables, following the naming conventions covered in class. e.g. for species_, name the accessor `Species`, and the mutator `SetSpecies`. ## Pet Class Create a `Pet` class with the following: ### Member Variables Create the following private member variables: 1. `std::string name_` 2. `Breed breed_` 3. `double weight_` ### Constructors 1. Create a default constructor for `Pet` that sets its name to `"Cookie"` and weight to `15.6`. The `Breed` object will automatically be created using its default constructor. 2. Create a non-default constructor that receives a `std::string` for `name_`, `Breed` for `breed_`, and a `double` for `weight_` in that order. The values from the constructor should appropriately assign the member variables. 3. Create another non-default constructor that receives a `std::string` for Pet's name stored in `name_`, `std::string` for the `Breed` species, `std::string` for the `Breed` name, and `std::string` for the `Breed` color, and `double` for `weight_`. The values accepted for the `Breed` species, `Breed` name, and `Breed` color should all be passed into the `Breed` constructor to create an object to assign to the `breed_` member variable (hint: you can initialize an object member variable within a member initializer list by invoking its constructor, see [this slide](https://docs.google.com/presentation/d/1zIAC4kj9FZ2GVZN2aMCJYm8qK_GFIKwfg55bW6b_s2M/edit#slide=id.g1636e2659d3_0_117)). The other values from the constructor should appropriately assign the member variables. ### Accessors and Mutators Create accessors and mutators for `name_`, `breed_`, and `weight_`. Please name the accessor for `breed_` as `GetBreed`, to avoid conflicting with the constructor for the `Breed` class. ### SetBreed overload Create a function overload for `SetBreed` that accepts a `species`, `breed_name`, and `color`, all of type `std::string`, that will internally create a `Breed` object using the values provided and then assign it to the `breed_` member variable. ### Print Create a member function called `Print` that returns `void` and does not take in any parameters. Using the member variables, this function should print out the name and weight of the `Pet`. It should also utilize accessors of the `Breed` class to get the species, breed name, and color. ## Other instructions Complete the `main` function as described. Place the `Pet` class in `pet.h`, and the `Breed` class in `breed.h`. Member functions that take more than ten lines or use complex constructs should have their function prototype in the respective `.h` header file and implementation in the respective `.cc` implementation file. ## Sample Output: ``` Please enter the pet's name (q to quit): Bitzy Please enter the pet's type: Dog Please enter the pet's breed: Chihuahua Please enter the pet's color: Tan Please enter the pet's weight (lbs): 11.5 Please enter the pet's name (q to quit): Cookie Please enter the pet's type: Dog Please enter the pet's breed: Long Chihuahua Please enter the pet's color: Brown & White Please enter the pet's weight (lbs): 6.2 Please enter the pet's name (q to quit): q Printing Pets: Pet 1 Name: Bitzy Species: Dog Breed: Chihuahua Color: Tan Weight: 11.5 lbs Pet 2 Name: Cookie Species: Dog Breed: Long Chihuahua Color: Brown & White Weight: 6.2 lbs I have solved the breed.h but got wrong on others breed.h: #include #ifndef BREED_H #define BREED_Hclass Breed { public: Breed(): species_ ("Dog"), breed_name_ ("Chihuahua"), color_ ("Fawn") {} Breed(std::string species, std::string breed_name, std::string color) { species_ = species; breed_name_ = breed_name; color_ = color; } std::string Species() const { return species_; } std::string BreedName() const { return breed_name_; } std::string Color() const { return color_; } void SetSpecies(std::string species) { species_ = species; } void SetBreedName(std::string breed_name) { breed_name_ = breed_name; } void SetColor(std::string color) { color_ = color; } private: // Declaring variables std::string species_; std::string breed_name_; std::string color_; }; #endif pet.h: #include #ifndef PET_H #define PET_H #include "breed.h" using namespace std; class Pet { public: private: string name_; Breed breed_; double weight_; }; #endif
# Pets
## Breed Class
Create a `Breed` class with the following:
### Member Variables
Create the following private member variables, all of type `std::string`:
1. `species_`
2. `breed_name_`
3. `color_`
### Constructors
1. Create a default constructor for `Breed` that sets its `species_` to `"Dog"`, `breed_name_` to `"Chihuahua"`, and `color_` to `"Fawn"`.
2. Create a non-default constructor that receives a `std::string` for `species_`, `breed_name_`, and `color_`; in that order. The values from the constructor should appropriately assign the member variables.
### Accessors and Mutators
Create accessors and mutators for all member variables, following the naming conventions covered in class. e.g. for species_, name the accessor `Species`, and the mutator `SetSpecies`.
## Pet Class
Create a `Pet` class with the following:
### Member Variables
Create the following private member variables:
1. `std::string name_`
2. `Breed breed_`
3. `double weight_`
### Constructors
1. Create a default constructor for `Pet` that sets its name to `"Cookie"` and weight to `15.6`. The `Breed` object will automatically be created using its default constructor.
2. Create a non-default constructor that receives a `std::string` for `name_`, `Breed` for `breed_`, and a `double` for `weight_` in that order. The values from the constructor should appropriately assign the member variables.
3. Create another non-default constructor that receives a `std::string` for Pet's name stored in `name_`, `std::string` for the `Breed` species, `std::string` for the `Breed` name, and `std::string` for the `Breed` color, and `double` for `weight_`. The values accepted for the `Breed` species, `Breed` name, and `Breed` color should all be passed into the `Breed` constructor to create an object to assign to the `breed_` member variable (hint: you can initialize an object member variable within a member initializer list by invoking its constructor, see [this slide](https://docs.google.com/presentation/d/1zIAC4kj9FZ2GVZN2aMCJYm8qK_GFIKwfg55bW6b_s2M/edit#slide=id.g1636e2659d3_0_117)). The other values from the constructor should appropriately assign the member variables.
### Accessors and Mutators
Create accessors and mutators for `name_`, `breed_`, and `weight_`. Please name the accessor for `breed_` as `GetBreed`, to avoid conflicting with the constructor for the `Breed` class.
### SetBreed overload
Create a function overload for `SetBreed` that accepts a `species`, `breed_name`, and `color`, all of type `std::string`, that will internally create a `Breed` object using the values provided and then assign it to the `breed_` member variable.
### Print
Create a member function called `Print` that returns `void` and does not take in any parameters. Using the member variables, this function should print out the name and weight of the `Pet`. It should also utilize accessors of the `Breed` class to get the species, breed name, and color.
## Other instructions
Complete the `main` function as described. Place the `Pet` class in `pet.h`, and the `Breed` class in `breed.h`. Member functions that take more than ten lines or use complex constructs should have their function prototype in the respective `.h` header file and implementation in the respective `.cc` implementation file.
## Sample Output:
```
Please enter the pet's name (q to quit): Bitzy
Please enter the pet's type: Dog
Please enter the pet's breed: Chihuahua
Please enter the pet's color: Tan
Please enter the pet's weight (lbs): 11.5
Please enter the pet's name (q to quit): Cookie
Please enter the pet's type: Dog
Please enter the pet's breed: Long Chihuahua
Please enter the pet's color: Brown & White
Please enter the pet's weight (lbs): 6.2
Please enter the pet's name (q to quit): q
Printing Pets:
Pet 1
Name: Bitzy
Species: Dog
Breed: Chihuahua
Color: Tan
Weight: 11.5 lbs
Pet 2
Name: Cookie
Species: Dog
Breed: Long Chihuahua
Color: Brown & White
Weight: 6.2 lbs
I have solved the breed.h but got wrong on others
breed.h:
#include <string>
#ifndef BREED_H
#define BREED_Hclass Breed
{
public:
Breed(): species_ ("Dog"), breed_name_ ("Chihuahua"), color_ ("Fawn") {}
Breed(std::string species, std::string breed_name, std::string color) {
species_ = species;
breed_name_ = breed_name;
color_ = color;
}
std::string Species() const {
return species_;
}
std::string BreedName() const {
return breed_name_;
}
std::string Color() const {
return color_;
}
void SetSpecies(std::string species) {
species_ = species;
}
void SetBreedName(std::string breed_name) {
breed_name_ = breed_name;
}
void SetColor(std::string color) {
color_ = color;
}
private:
// Declaring variables
std::string species_;
std::string breed_name_;
std::string color_;
};
#endif
pet.h:
#include <string>
#ifndef PET_H
#define PET_H
#include "breed.h"
using namespace std;
class Pet
{
public:
private:
string name_;
Breed breed_;
double weight_;
};
#endif
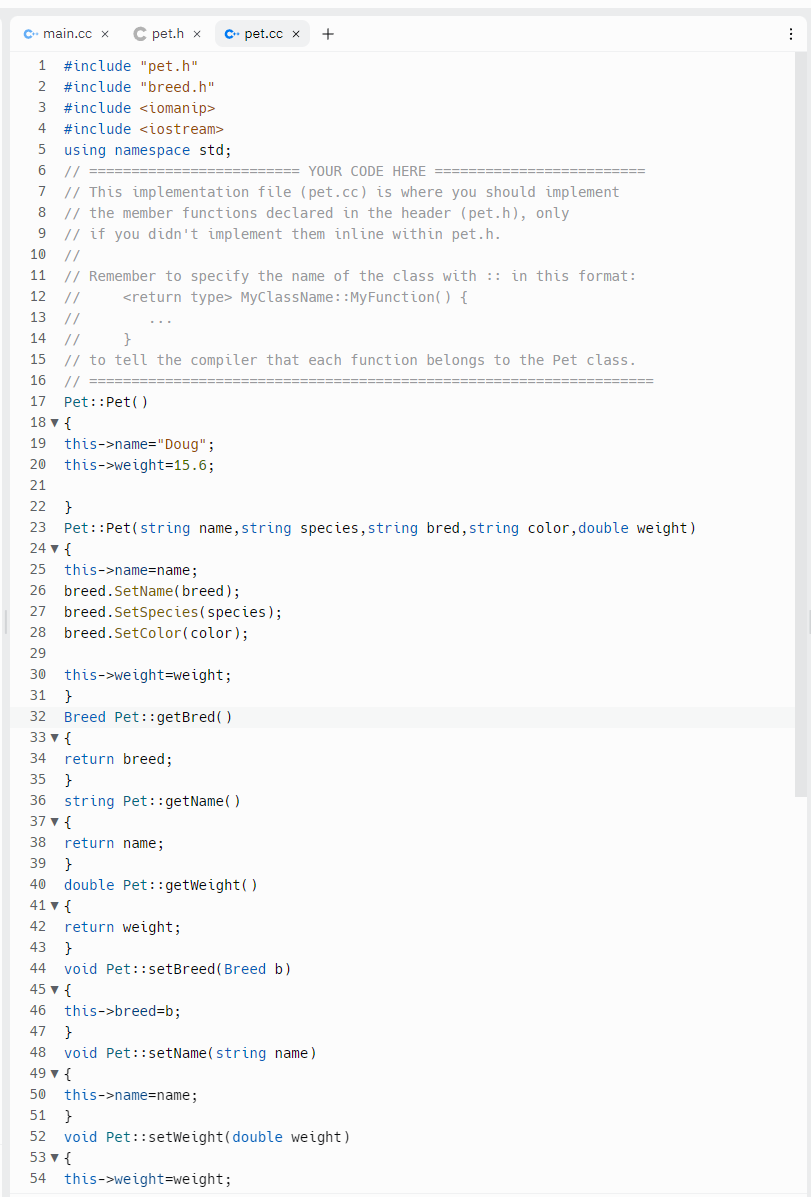
![⠀
⠀
C pet.hx
1 #include <iostream>
2 #include "pet.h"
3 #include <vector>
4 using namespace std;
5
6
7
8
C+ main.ccx
int main() {
// Create an array of 100 pet objects called 'pet_arr`
9 Pet* pet_arr=new Pet [100];
10
int num_pet = 0;
11 std::string name;
std::string breed;
12
13
std::string species;
14 std::string color;
15 double weight;
16 ▼ do {
17
std::cout << "Please enter the pet's name (q to quit): ";
18 std::getline(std::cin, name);
19
if (name != "q") {
20
std::cout << "Please enter the pet's species: ";
21 std::getline(std::cin, species);
22 std::cout << "Please enter the pet's breed: ";
23 std::getline(std::cin, breed);
24 std::cout << "Please enter the pet's color: ";
25 std::getline(std::cin, color);
26 std::cout << "Please enter the pet's weight (lbs): ";
27 std::cin>> weight;
28 std::cin.ignore();
29 // Create a pet object using the input from the user
30 Pet p(name, species, breed, color, weight);
31 // Store the newly-created pet object into the array. Use `num_pet to
32 pet_arr[num_pet]=p;
33 num_pet++;
34 // control the index where the pet object is placed and update it
35 // accordingly
36 }
37 } while (name != "q");
38 std::cout << "Printing Pets:\n";
39 for (int i = 0; i < num_
i++) {
40 std::cout << "Pet " << i + 1 << "\n";
1 2 3 4 45
41 // Print information about the `i`th pet object
42 pet_arr[i].print();
C- pet.cc x +
43
}
44 return 0;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2216a831-152e-4ce3-9ac2-b23e19574410%2F772f2211-9fcd-40b1-b7a8-37095ff70de4%2F8kbrn6m_processed.png&w=3840&q=75)

Step by step
Solved in 5 steps with 1 images

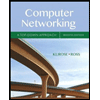
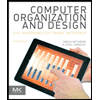
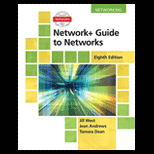
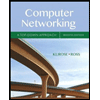
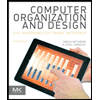
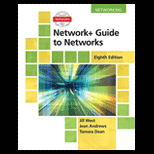
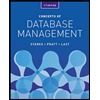
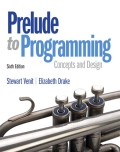
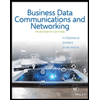