__init__(self, id: int, fist_name: str, last_name: str, town:str): """ This creates a student object with the specified ID first and last name and home town. This constructor should also create data structure for holding the students grades for all of there assignments. Additionally it should create a variable that holds the student's energy level which will be a number between 0 and 1. :param id: The student's identifiaction number :param fist_name: The student's first name :param last_name: The student's last name :param town: The student's home town """ get_id(self)->int: """ Returns the ID of the student as specified in the constructor. :return: The student's ID """ get_first_name(self) -> str: """ Returns the first name of the student. :return: The student's first name """ set_first_name(self, name:str): """ Changes the student first name to the specified value of the name parameter. :param name: The value that the first name of the student will equal. """ get_last_name(self) -> str: """ Returns the last name of the student. :return: The student's last name """ set_last_name(self, name: str): """ Changes the student last name to the specified value of the name parameter. :param name: The value that the last name of the student will equal. """ get_town(self) -> str: """ Returns the hometown of the student. :return: The student's town name """ set_town(self, town: str): """ Changes the student's hometown to the specified value of the town parameter. :param name: The value that the hometown of the student will equal. """ __str__(self) ->str: """ Returns a string containing the student's first and last name separated by a space. :return: Returns a string of the full name of the student """ get_grade(self)->float: """ Calculates a an average grade based off of the student's past assignment's grades. The lowest grade is not included in the grade calculation if the student has been assigned to two or more assignments in the past. See assign() for more detains. If the student has not been assigned any assignments in the pass this should return 0. :return: A number between 0-1 indicating the student's grade """ assign(self, assignment:Assignment) -> AssignmentResult: """ This function is to simulate the process of the student receiving an assignment, then working on the assignment, then submitting the assignment and finally receiving grade for the assignment. This function will receive an assignment then a grade should be calculated using the following formula: grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0) After the grade is calculated the student's energy should be decreased by percentage difficulty. Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0) Finally the grade calculated should be stored internally with in this class so it can be retrieved later. Then an Assignment Result object should be created with the student's ID, the assignment received as a parameter, and the grade calculated. This newly created Assignment Result object should be returned. :return: The an AssignmentResult outlining this process """ sleep(self, hours:float): """ This function restore the student's energy as a rate of 10% per hour. So if they sleep for 8 hours there energy will be restored by 80%. If they have 50% (.5) energy and sleep for 8 hours the will wake up with 90% energy = (.5 * (1+.8)). The max energy a s student may have is 100% (1) :param hours: The number of hours a student will sleep for. Example: .2 is equal to 12 minutes or 20% of an hour. :return: None """ get_energy(self): """ Returns the current energy of the student. A number between 0 and 1 :return: The energy of the student. """
Class Student
__init__(self, id: int, fist_name: str, last_name: str, town:str):
""" This creates a student object with the specified ID first and last name and home town. This constructor should also create data structure for holding the students grades for all of there assignments. Additionally it should create a variable that holds the student's energy level which will be a number between 0 and 1. :param id: The student's identifiaction number :param fist_name: The student's first name :param last_name: The student's last name :param town: The student's home town """
get_id(self)->int:
""" Returns the ID of the student as specified in the constructor. :return: The student's ID """
get_first_name(self) -> str:
""" Returns the first name of the student. :return: The student's first name """
set_first_name(self, name:str):
""" Changes the student first name to the specified value of the name parameter. :param name: The value that the first name of the student will equal. """
get_last_name(self) -> str:
""" Returns the last name of the student. :return: The student's last name """
set_last_name(self, name: str):
""" Changes the student last name to the specified value of the name parameter. :param name: The value that the last name of the student will equal. """
get_town(self) -> str:
""" Returns the hometown of the student. :return: The student's town name """
set_town(self, town: str):
""" Changes the student's hometown to the specified value of the town parameter. :param name: The value that the hometown of the student will equal. """
__str__(self) ->str:
""" Returns a string containing the student's first and last name separated by a space. :return: Returns a string of the full name of the student """
get_grade(self)->float:
""" Calculates a an average grade based off of the student's past assignment's grades. The lowest grade is not included in the grade calculation if the student has been assigned to two or more assignments in the past. See assign() for more detains. If the student has not been assigned any assignments in the pass this should return 0. :return: A number between 0-1 indicating the student's grade """
assign(self, assignment:Assignment) -> AssignmentResult:
""" This function is to simulate the process of the student receiving an assignment, then working on the assignment, then submitting the assignment and finally receiving grade for the assignment. This function will receive an assignment then a grade should be calculated using the following formula: grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0)
After the grade is calculated the student's energy should be decreased by percentage difficulty. Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0) Finally the grade calculated should be stored internally with in this class so it can be retrieved later. Then an Assignment Result object should be created with the student's ID, the assignment received as a parameter, and the grade calculated. This newly created Assignment Result object should be returned.
:return: The an AssignmentResult outlining this process """
sleep(self, hours:float):
""" This function restore the student's energy as a rate of 10% per hour. So if they sleep for 8 hours there energy will be restored by 80%. If they have 50% (.5) energy and sleep for 8 hours the will wake up with 90% energy = (.5 * (1+.8)). The max energy a s student may have is 100% (1)
:param hours: The number of hours a student will sleep for. Example: .2 is equal to 12 minutes or 20% of an hour. :return: None """
get_energy(self):
""" Returns the current energy of the student. A number between 0 and 1 :return: The energy of the student. """

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

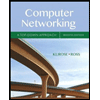
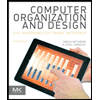
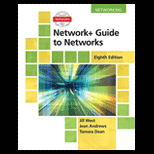
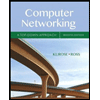
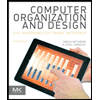
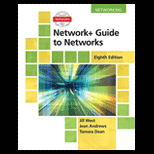
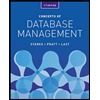
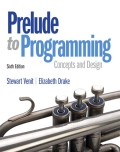
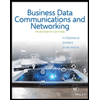