Phyton: Program 6: Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it. You should have the following functions: · add_from_file() – prompts the user for a file and add the data from it. · add_by_hand() – prompts the user to enter data until user type a negative number. · print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions. Handling errors such as whether the input file exist is extra credit. To print a 10-column table, you can use the code below: for i in range(len(data)): print(f"{data[i]:3}", end="") if (i+1)%10==0: print() print() Sample run: Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 2 Type a positive number (-1 to end):1 Type a positive number (-1 to end):2 Type a positive number (-1 to end):3 Type a positive number (-1 to end):-1 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 1 Enter file to read: a.txt Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 1 Enter file to read: b.txt Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 9 8 5 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 2 Type a positive number (-1 to end):10 20 Type a positive number (-1 to end):20 Type a positive number (-1 to end):30 Type a positive number (-1 to end):-1 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 9 8 5 10 20 30 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 9 8 5 10 20 30 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 3 min = 1 max = 30 mean= 7.769230769230769 sum = 101 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 5 Goodbye
Phyton:
Program 6:
Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it.
You should have the following functions:
- · add_from_file() – prompts the user for a file and add the data from it.
- · add_by_hand() – prompts the user to enter data until user type a negative number.
- · print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions.
Handling errors such as whether the input file exist is extra credit.
To print a 10-column table, you can use the code below:
for i in range(len(data)): print(f"{data[i]:3}", end="") if (i+1)%10==0: print() print() |
Sample run:
Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 2 Type a positive number (-1 to end):1 Type a positive number (-1 to end):2 Type a positive number (-1 to end):3 Type a positive number (-1 to end):-1 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 1 Enter file to read: a.txt Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 1 Enter file to read: b.txt Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 9 8 5
Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 2 Type a positive number (-1 to end):10 20 Type a positive number (-1 to end):20 Type a positive number (-1 to end):30 Type a positive number (-1 to end):-1 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 9 8 5 10 20 30 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 4 1 2 3 3 1 4 5 9 8 5 10 20 30 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 3 min = 1 max = 30 mean= 7.769230769230769 sum = 101 Choose an action 1) add data from a file 2) add data by hand 3) print stats 4) view data 5) exit Choice: 5 Goodbye |

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

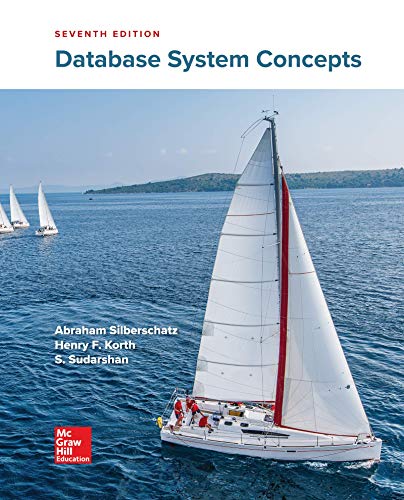
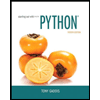
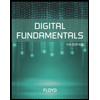
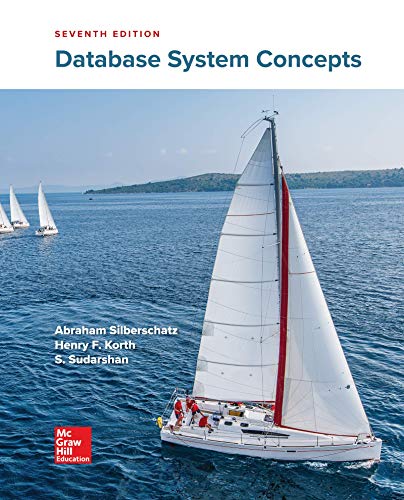
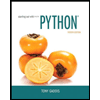
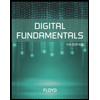
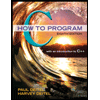
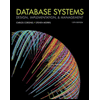
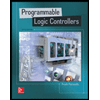