please code in python A mining company conducts a survey of an n-by-n square grid of land. Each row of land is numbered from 0 to n-1 where 0 is the top and n-1 is the bottom, and each column is also numbered from 0 to n-1 where 0 is the left and n-1 is the right. The company wishes to record which squares of this grid contain mineral deposits. The company decides to use a list of tuples to store the location of each deposit. The first item in each tuple is the row of the deposit. The second item is the column. The third item is a non-negative number representing the size of the deposit, in tons. For example, the following code defines a sample representation of a set of deposits in an 8-by-8 grid. deposits = [(0, 4, .3), (6, 2, 3), (3, 7, 2.2), (5, 5, .5), (3, 5, .8), (7, 7, .3)] Given a list of deposits like the one above, write a function to create a string representation for a rectangular sub-region of the land. Your function should take a list of deposits, then a set of parameters denoting the top, bottom, left, and right edges of the sub-grid. It should return (do not print in the function) a multi-line string in which grid squares without deposits are represented by "-" and grid squares with a deposit are represented by "X". def display(deposits, top, bottom, left, right): """display a subgrid of the land, with rows starting at top and up to but not including bottom, and columns starting at left and up to but not including right.""" ans = "" # delete this line and enter your own code return ans complete the following function to compute the total number of tons (as a float) in a rectangular sub-region of the grid. def tons_inside(deposits, top, bottom, left, right): """Returns the total number of tons of deposits for which the row is at least top, but strictly less than bottom, and the column is at least left, but strictly less than right.""" # Do not alter the function header. # Just fill in the code so it returns the correct number of tons. pass # delete this statement before entering your code!
please code in python
A mining company conducts a survey of an n-by-n square grid of land. Each row of land is numbered from 0 to n-1 where 0 is the top and n-1 is the bottom, and each column is also numbered from 0 to n-1 where 0 is the left and n-1 is the right. The company wishes to record which squares of this grid contain mineral deposits.
The company decides to use a list of tuples to store the location of each deposit. The first item in each tuple is the row of the deposit. The second item is the column. The third item is a non-negative number representing the size of the deposit, in tons. For example, the following code defines a sample representation of a set of deposits in an 8-by-8 grid.
deposits = [(0, 4, .3), (6, 2, 3), (3, 7, 2.2), (5, 5, .5), (3, 5, .8), (7, 7, .3)]
Given a list of deposits like the one above, write a function to create a string representation for a rectangular sub-region of the land. Your function should take a list of deposits, then a set of parameters denoting the top, bottom, left, and right edges of the sub-grid. It should return (do not print in the function) a multi-line string in which grid squares without deposits are represented by "-" and grid squares with a deposit are represented by "X".
def display(deposits, top, bottom, left, right):
"""display a subgrid of the land, with rows starting at top and up to
but not including bottom, and columns starting at left and up to but
not including right."""
ans = "" # delete this line and enter your own code
return ans
complete the following function to compute the total number of tons (as a float) in a rectangular sub-region of the grid.
def tons_inside(deposits, top, bottom, left, right):
"""Returns the total number of tons of deposits for which the row is at least top,
but strictly less than bottom, and the column is at least left, but strictly
less than right."""
# Do not alter the function header.
# Just fill in the code so it returns the correct number of tons.
pass # delete this statement before entering your code!
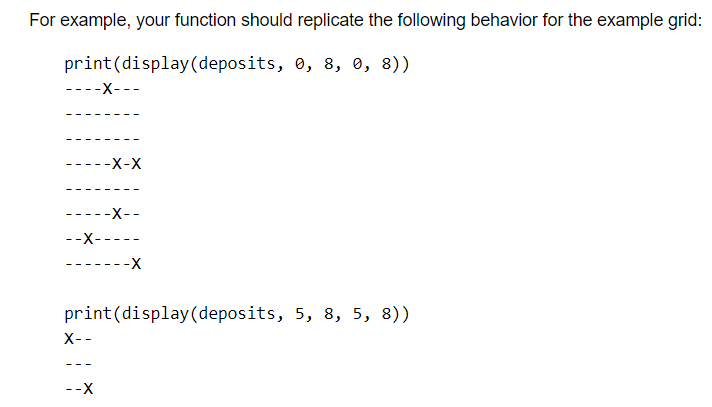

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

please code in python
this is the continuation of the above question
How would we solve ti
def tons_inside(deposits, top, bottom, left, right):
"""Returns the total number of tons of deposits for which the row is at least top,
but strictly less than bottom, and the column is at least left, but strictly
less than right."""
# Do not alter the function header.
# Just fill in the code so it returns the correct number of tons.
pass # delete this statement before entering your code!
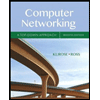
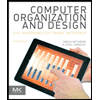
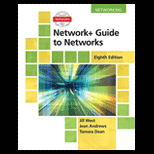
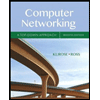
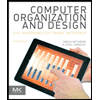
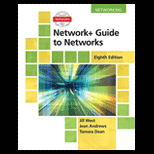
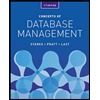
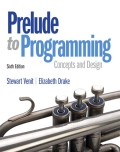
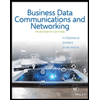