int compares (Card cl, Card c2) This method takes two card objects and returns -1, 0, or 1 if c1 is smaller, equal to, or greater than c2, respectively. You need to compare their suits first and if they have the same suit, then compare their ranks. void quickSort (Card [] cardArray, int first, int last) This method takes a card array as well as two indices. It sorts the cards in the range specified by first and last with quick sort. It first calls the split method to partition the elements in the range into two subarrays, and sorts both subarrays recursively. It repeats this process until the entire array is sorted. int split (Card [] cardArray, int first, int last) This is a helper method for quick sort which takes a card array and two indices. It begins by selecting the first element in the card array as the pivot, and then moves elements so that everything in the left subarray is smaller than the pivot and everything on the right is greater than the pivot. The method returns the final index of the pivot. You will need to call the compares method to make comparisons between cards.
I need help implementing those functions.
public class DeckCards
{
// card comparison
public int compares(Card c1, Card c2)
{
return - 1; // replace this statement with your own return
}
// This is the wrapper method for quick sort
// Do not make any changes to this method!
public void quickSort(Card[] cardArray)
{
quickSortRec(cardArray, 0, cardArray.length - 1);
}
// This is the recursive helper method for quickSort
public void quickSortRec(Card[] cardArray, int first, int last)
{
// TODO: implement this method
}
// This method splits a Card array into two sections, with all the elements less
// than the pivot in the left section and all the elements greater than the pivot
// in the right section
public int split(Card[] cardArray, int first, int last)
{
// TODO: implement this method
return -1; // replace this statement with your own return
}
// This method takes an int array which represents a complete binary tree as
// the parameter. It checks if the tree stored in the array is a heap.
public boolean isHeap(int[] A)
{
// TODO: implement this method
return false; // replace this statement with your own return
}
}
![int compares (Card c1, Card c2)
This method takes two card objects and returns -1, 0, or 1 if c1 is smaller, equal to, or greater
than c2, respectively. You need to compare their suits first and if they have the same suit, then
compare their ranks.
void quickSort (Card [] cardArray, int first, int last)
This method takes a card array as well as two indices. It sorts the cards in the range specified
by first and last with quick sort. It first calls the split method to partition the elements in
the range into two subarrays, and sorts both subarrays recursively. It repeats this process until
the entire array is sorted.
int split (Card [] cardArray, int first, int last)
This is a helper method for quick sort which takes a card array and two indices. It begins by
selecting the first element in the card array as the pivot, and then moves elements so that
everything in the left subarray is smaller than the pivot and everything on the right is greater
than the pivot. The method returns the final index of the pivot. You will need to call the
compares method to make comparisons between cards.
boolean isHeap (int[] A)
This method takes an int array as the parameter and verifies if the elements saved in this
array form a heap. You can assume that the array represents a complete binary tree so the
shape property is already satisfied. You will need to verify that the elements meet the order
property requirement for heaps. Return true if this is a heap, false otherwise. This method is
separate from the above methods and has nothing to do with the card or Deck classes used by
other methods.
Note that you are only supposed to touch the above methods. You are NOT allowed to
create any other methods, instance variables, or make any changes to methods other
than these methods or files other than "Homework6.java". Points will be taken off if you
fail to follow this rule.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffdd44bf7-1ef3-43ad-9026-09878676258e%2F5a48a9ca-a9d2-4f0e-90a1-01ba6031ff71%2Fqi8nrom_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Unfortunately, the code itself executes but recursively in infinite loop. Is there a reason why it is doing that?
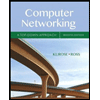
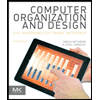
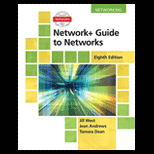
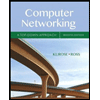
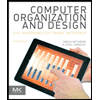
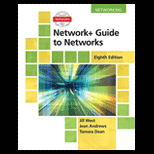
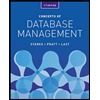
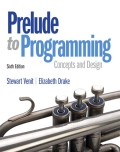
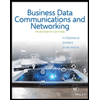