Please come up with some scenario where mocking is required, write the Python class, and the Python unit test that included the patch that enables the mocking. You may patch a single value using return_value, or multiple values with side_effect. from territory import Territory from army import Army from territory_test import TestDoBattle t = Territory(10, Army()) t.do_battle(Army()) print(t.curr_territory) unittest.main() ----- class Army: def get_curr_strength(self): return 0 # pretend there is code here that returns an actual value ------ from army import Army class Territory: def __init__(self, initial_territory, this_army): self.curr_territory = initial_territory self.this_army = this_army def do_battle(self, enemy_army): self.curr_territory += self.this_army.get_curr_strength() - \ enemy_army.get_curr_strength() ------ something similar to this code: from territory import Territory from army import Army import unittest from unittest.mock import patch class TestThisArmyTenEnemyFiveIncreasesTerritoryByFive(unittest.TestCase): @patch('army.Army.get_curr_strength', side_effect = (10,5)) def runTest(self, _): t = Territory(10, Army()) t.do_battle(Army()) self.assertEqual(15, t.curr_territory) unittest.main()
Please come up with some scenario where mocking is required, write the Python class, and the Python unit test that included the patch that enables the mocking. You may patch a single value using return_value, or multiple values with side_effect.
from territory import Territory
from army import Army
from territory_test import TestDoBattle
t = Territory(10, Army())
t.do_battle(Army())
print(t.curr_territory)
unittest.main()
-----
class Army:
def get_curr_strength(self):
return 0 # pretend there is code here that returns an actual value
------
from army import Army
class Territory:
def __init__(self, initial_territory, this_army):
self.curr_territory = initial_territory
self.this_army = this_army
def do_battle(self, enemy_army):
self.curr_territory += self.this_army.get_curr_strength() - \
enemy_army.get_curr_strength()
------
something similar to this code:
from territory import Territory
from army import Army
import unittest
from unittest.mock import patch
class TestThisArmyTenEnemyFiveIncreasesTerritoryByFive(unittest.TestCase):
@patch('army.Army.get_curr_strength', side_effect = (10,5))
def runTest(self, _):
t = Territory(10, Army())
t.do_battle(Army())
self.assertEqual(15, t.curr_territory)
unittest.main()

Step by step
Solved in 2 steps with 2 images

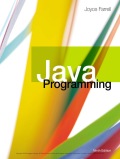
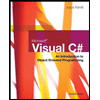
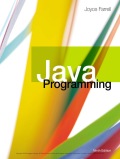
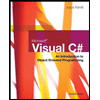
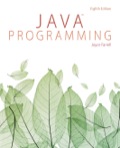
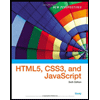