Answer the given question with a proper explanation and step-by-step solution. USING JAVA: ---Sorting the Invoices via dates--- Another error that will still be showing is that there is not Comparable/compareTo() method setup on the Invoice class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the Invoice class. The compareTo() method will take a little work here. We are going to compare via the date of the invoice. The dates of the Invoice class are by default saved in a MM-DD-YYYY format. So they have a dash '-' between each part of the date. So you will need to split the date of the current invoice AND split the date of the object sent to the method. We have three things to compare against here. First we need to check the year. If the years are the same then you should go another step forward and compare the months. If the months are the same then you will lastly have to compare the day. Use whatever method you want. Remember that these are in String format, so you could try the compareTo() on String but what happens if the date is 09 for month, does it compare that correctly. Otherwise think about parsing the data to ints.
Answer the given question with a proper explanation and step-by-step solution.
USING JAVA:
---Sorting the Invoices via dates---
Another error that will still be showing is that there is not Comparable/compareTo() method setup on the Invoice class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the Invoice class.
The compareTo() method will take a little work here. We are going to compare via the date of the invoice. The dates of the Invoice class are by default saved in a MM-DD-YYYY format. So they have a dash '-' between each part of the date. So you will need to split the date of the current invoice AND split the date of the object sent to the method.
We have three things to compare against here. First we need to check the year. If the years are the same then you should go another step forward and compare the months. If the months are the same then you will lastly have to compare the day.
Use whatever method you want. Remember that these are in String format, so you could try the compareTo() on String but what happens if the date is 09 for month, does it compare that correctly. Otherwise think about parsing the data to ints.
-----Invoice.java-----
import java.text.NumberFormat;
public class Invoice implements Comparable {
private String id;
private String date;
private String lastName;
private String firstName;
private String address;
private String paint;
private PaintFinish finish;
private String color;
private double cost;
private static final NumberFormat currency = NumberFormat.getCurrencyInstance();
public Invoice(String id, String date, String lastName, String firstName, String address, String paint,
PaintFinish finish, String color, double cost) {
this.id = id;
this.date = date;
this.lastName = lastName;
this.firstName = firstName;
this.address = address;
this.paint = paint;
this.finish = finish;
this.color = color;
this.cost = cost;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPaint() {
return paint;
}
public void setPaint(String paint) {
this.paint = paint;
}
public PaintFinish getPaintFinish() {
return finish;
}
public void setPaintFinish(PaintFinish paintFinish) {
this.finish = paintFinish;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public double getCost() {
return cost;
}
public void setCost(double cost) {
this.cost = cost;
}
@Override
public String toString() {
return String.format("Invoice ID: %s\nInvoice Date: %s\nCustomer Name: %s %s\nAddress: %s" +
"\nPaint: %s %s\nColor: %s\nTotal Cost: %s",
id, date, firstName, lastName, address, finish.toString(), paint, color, currency.format(cost));
}
public String fileWriteOut() {
return String.format("%s\t%s\t%s\t%s\t%s\t%s\t%s\t%s\t%.2f",
id, date, lastName, firstName, address, paint, finish.toString(), color, cost);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Invoice) {
Invoice i = (Invoice) obj;
return i.getId().equalsIgnoreCase(id);
}
return false;
}
@Override
public int compareTo(Object obj) {
Invoice i = (Invoice) obj;
String[] dateSplit = date.split("-");
int curMonth = Integer.parseInt(dateSplit[0]);
int curDay = Integer.parseInt(dateSplit[1]);
int curYear = Integer.parseInt(dateSplit[2]);
String[] iDateSplit = i.getDate().split("-");
int iMonth = Integer.parseInt(iDateSplit[0]);
int iDay = Integer.parseInt(iDateSplit[1]);
int iYear = Integer.parseInt(iDateSplit[2]);
if (curYear < iYear) return -1;
else if (curYear > iYear) return 1;
else {
if (curMonth < iMonth) return -1;
else if(curMonth > iMonth) return 1;
else {
if (curDay < iDay) return -1;
else if (curDay > iDay) return 1;
else return 0;
}
}
}
}
-----PaintjobInvoices.txt-----
id date lastname firstname address paint paintfinish color cost
1589 01-25-2023 Banner Bruce 890 5th Ave, Manhattan, New York 10451 Dutch Boy Flat Calming Gray 1026.75
8536 12-22-2022 Odinson Thor Asgard Behr Gloss Cape Red 836.59
2859 01-05-2023 Barnes Bucky 166 Montague St, Brooklyn, NY 11201 Dutch Boy Flat Off-Black 796.18
3695 01-12-2023 Odinson Loki Asgard Dutch Boy Gloss Emerald 586.25
7698 02-23-2023 Wilson Sam 1425 4th St A616, Washington, DC 20024 Behr Satin See-through Red 674.25
1234 02-15-2023 Stark Tony 10880 Malibu Point, Malibu, CA 90265 Sherwin Williams Semi-Gloss Hot Rod Red 2631.58
9563 11-14-2022 Barton Clint Iowa Sherwin Williams Satin Bullseye Yellow 156.48
3589 02-25-2023 Maximoff Wanda 2800 Sherwood Dr, Westview, New Jersey 08801 Dutch Boy Flat Tan 965.23
4569 02-24-2023 Panther Clan Shuri Wakanda Palace Sherwin Williams Semi-Gloss Vibranium Silver 8269.45

Step by step
Solved in 3 steps

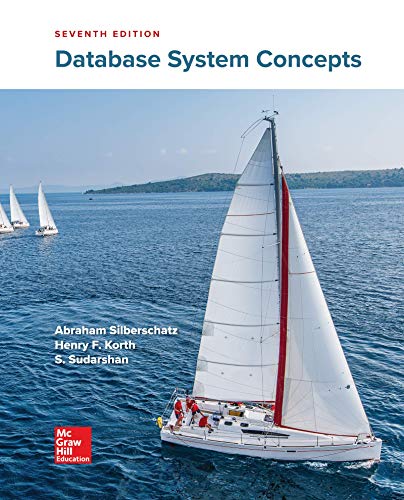
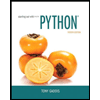
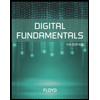
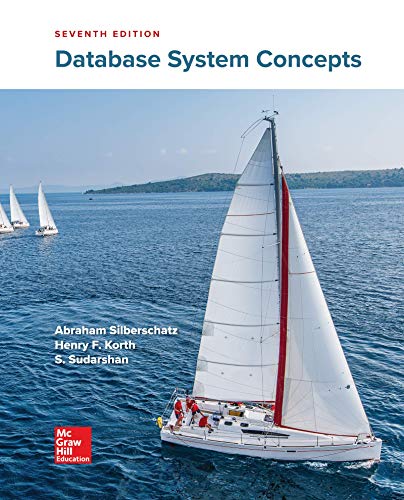
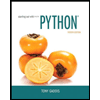
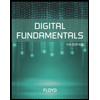
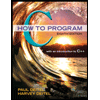
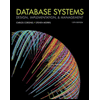
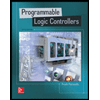