Please convert to C language //linear probing #include using namespace std; void add_using_linear_probing(int hash[], int a) { //hash function h(x)=x%10 int k = a % 10; //linear probing while (true) { if (hash[k] == -1) { hash[k] = a; break; } k = (k + 1) % 10; //linear increment of probe } } int main() { //set of input numbers vector arr{ 123, 124, 333, 4679, 983 }; //initialize the hash table //each entry of the hash table is a single entry int hash[10]; //size of hashtable is 10 memset(hash, -1, sizeof(hash)); //initialize with empty initially for (int a : arr) { //hashing add_using_linear_probing(hash, a); } cout << "---------using linear probing---------\n"; cout << "Hash table is:\n"; for (int i = 0; i < 10; i++) { if (hash[i] == -1) cout << i << "->" << "Empty" << endl; else cout << i << "->" << hash[i] << endl; } return 0; }
Please convert to C language
//linear probing
#include <bits/stdc++.h>
using namespace std;
void add_using_linear_probing(int hash[], int a)
{
//hash function h(x)=x%10
int k = a % 10;
//linear probing
while (true) {
if (hash[k] == -1) {
hash[k] = a;
break;
}
k = (k + 1) % 10; //linear increment of probe
}
}
int main()
{
//set of input numbers
//initialize the hash table
//each entry of the hash table is a single entry
int hash[10]; //size of hashtable is 10
memset(hash, -1, sizeof(hash)); //initialize with empty initially
for (int a : arr) {
//hashing
add_using_linear_probing(hash, a);
}
cout << "---------using linear probing---------\n";
cout << "Hash table is:\n";
for (int i = 0; i < 10; i++) {
if (hash[i] == -1)
cout << i << "->"
<< "Empty" << endl;
else
cout << i << "->" << hash[i] << endl;
}
return 0;
}
Output:
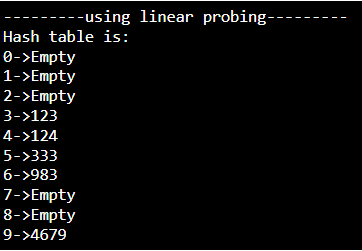

Step by step
Solved in 2 steps with 1 images

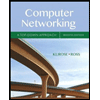
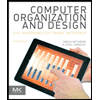
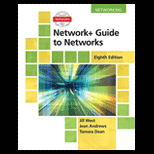
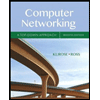
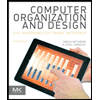
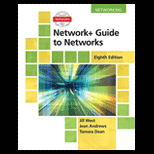
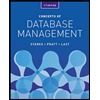
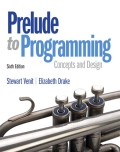
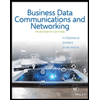