#include #include #include #define capacity 101 // Size of Hash Table int hash_function(char* str) { int i = 0; for (int j=0; str[j]; j++) i += str[j]; return i % capacity; } struct Ht_item { char* name; int numberCourses; }; // Hash Table Definition struct HashTable { struct Ht_item** items; int size; int count; }; struct Ht_item* create_item(char* key, int value) { struct Ht_item* item = (struct Ht_item*) malloc (sizeof(struct Ht_item)); item->name = (char*) malloc (strlen(key) + 1); item->numberCourses = value; strcpy(item->name, key); item->numberCourses = value; return item; } struct HashTable* create_table(int size) { // Creates a New Hash Table struct HashTable* table = (struct HashTable*) malloc (sizeof(struct HashTable)); table->size = size; table->count = 0; table->items = (struct Ht_item**) calloc (table->size, sizeof(struct Ht_item*)); for (int i=0; isize; i++) table->items[i] = 0; return table; } void put(struct HashTable* table, char* key, int value) { struct Ht_item* item = create_item(key, value); int index = hash_function(key); struct Ht_item* current_item = table->items[index]; if (current_item == 0) { // Key Not Available if (table->count == table->size) { // Hash Table Full printf("Hash Table Full...\n"); return; } // Direct Insertion table->items[index] = item; table->count++; } else { if (strcmp(current_item->name, key) == 0) { table->items[index]->numberCourses = value; return; } else { // Write the collision case using loop? } } } int contains(struct HashTable* table, char* key) { // Search in Hash Table // Return 0 if Not Available int index = hash_function(key); struct Ht_item* item = table->items[index]; if (item != 0) { if (strcmp(item->name, key) == 0) return item->numberCourses; } return 0; }
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define capacity 101 // Size of Hash Table
int hash_function(char* str) {
int i = 0;
for (int j=0; str[j]; j++)
i += str[j];
return i % capacity;
}
struct Ht_item {
char* name;
int numberCourses;
};
// Hash Table Definition
struct HashTable {
struct Ht_item** items;
int size;
int count;
};
struct Ht_item* create_item(char* key, int value) {
struct Ht_item* item = (struct Ht_item*) malloc (sizeof(struct Ht_item));
item->name = (char*) malloc (strlen(key) + 1);
item->numberCourses = value;
strcpy(item->name, key);
item->numberCourses = value;
return item;
}
struct HashTable* create_table(int size) {
// Creates a New Hash Table
struct HashTable* table = (struct HashTable*) malloc (sizeof(struct HashTable));
table->size = size;
table->count = 0;
table->items = (struct Ht_item**) calloc (table->size, sizeof(struct Ht_item*));
for (int i=0; i<table->size; i++)
table->items[i] = 0;
return table;
}
void put(struct HashTable* table, char* key, int value) {
struct Ht_item* item = create_item(key, value);
int index = hash_function(key);
struct Ht_item* current_item = table->items[index];
if (current_item == 0) {
// Key Not Available
if (table->count == table->size) {
// Hash Table Full
printf("Hash Table Full...\n");
return;
}
// Direct Insertion
table->items[index] = item;
table->count++;
}
else {
if (strcmp(current_item->name, key) == 0) {
table->items[index]->numberCourses = value;
return;
}
else {
// Write the collision case using loop?
}
}
}
int contains(struct HashTable* table, char* key) {
// Search in Hash Table
// Return 0 if Not Available
int index = hash_function(key);
struct Ht_item* item = table->items[index];
if (item != 0) {
if (strcmp(item->name, key) == 0)
return item->numberCourses;
}
return 0;
}
int get(struct HashTable* table, char* key){
}
void display(struct HashTable* table, char* key) {
int val;
if ((val = contains(table, key)) == 0) {
printf("Key: %s Not Available...\n", key);
return;
}
else {
printf("Key: %s, Value: %d\n", key, val);
}
}
int main() {
struct HashTable* ht = create_table(capacity);
put(ht, "Alex", 4);
put(ht, "Amy", 3);
put(ht, "Tiny", 7);
put(ht, "Amy", 2);
put(ht, "Cary", 3);
display(ht, "Mark");
display(ht, "Amy");
display(ht, "Tiny");
display(ht, "Cary");
display(ht, "Alex");
return 0;
}
The code for the problem is given above.
// Write the collision case using loop? (handle the collision case in the comment section and it will search linearly.)
The get function itself gives the number of lessons learned for the most sent key. send it. If the key is not present in the map, it returns -1. (Write the get function in accordance with the definition.)
Complete the code by adding the specified problems to the code.

Step by step
Solved in 2 steps

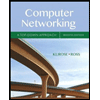
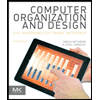
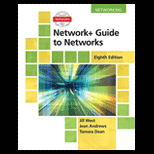
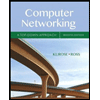
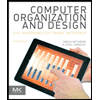
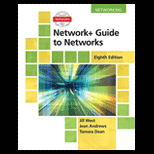
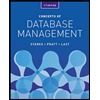
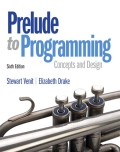
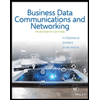