Please develop a Python application that meets the requirements described below: Project Description: Scenario: Write a program for a retail store that will allow them to calculate discounts for their employees when they buy items. Discounts are based on the number of years worked (2% for each year, maximum 10%) as well as if the employee is a manager (10% more discount on top of the worked year discount) or hourly employee (2% discount). They are also allowed no discount once they have received $200 discount. The program starts asking for the employee discount number to start purchasing. The employee can purchase 1 item at a time. Do this until the user answers “NO” for “Another purchase?”. Once all employees have been processed, display the All-Employee Summary and give the users option to go back to Menu or Exit the program. A workflow diagram is provided for each function to help visualize the flow through the program. Actions: Menu Pages: Create the menus below using a square created in the console by printing dashes (-) and pipes (|) where appropriate. It should look something like this but large enough to cover almost ½ of your computer screen when printed on the console (no need to calculate for the monitor size- just approximate is fine): ———— | | ———— e.g. ——————————————— | 1- Create Employee | | 2- Create Item | | 3- Make Purchase | | 4-All Employee Summary | | 5-Exit | ——————————————— 2. Specific Menu Pages to Create: 2.1. Create Employee Page Please define a function that asks the user to get the employees’ information and add that to the list. Whenever the user enters “NO” it will finish getting the employees’ information. 2. Use a 2-dimensional list to create and save the employee information where each item in the list consists of the information of 1 employee: [Employee ID, Employee Name, Employee Type, Years Worked, Total Purchased, Total Discounts, Employee Discount Number] E.g. employee_list = [ [1001, John Alber, hourly, 8, 0, 0, 22737], [1002, Sarah Rose, manager, 12, 0, 0, 22344], [1003, Alex Folen, manager, 5, 0, 0, 22957], [1004, Pola Sahari, hourly, 17, 0, 0, 22488] ] 3.Inputs must be validated to satisfy the following requirements: Employee ID is unique within the list Employee Discount is unique within the list Null/empty value is not allowed for any of the fields Input must be number for Employee ID, Years Worked, and Employee Discount Number Input must be “hourly” or “manager” for Employee Type Input is not required for Total Purchased and Total Discounts when the employee is first created in the system, these fields should be assigned with a default value of Below is the workflow diagram for the Create Employee Process, the check for null values is not included to simplify the diagram. Please make sure to implement all the checks as per requirements. 2.1. Create Item Page Please define a function that asks the user to get the items’ information and add that to the Whenever the user enters “NO” it will finish getting the items’ information. Use a 2-dimensional list to create and save the item information where each item in the list consists of similar information as follows: [Item Number, Item Name, Item Cost] E.g. item_list = [ [11526, Nike shoes, 120], [11849, Trampoline,180], [11966, Mercury Bicycle, 150], [11334, Necklace Set, 80] ] Inputs must be validated to satisfy the following requirements: Item Number is unique within the list Null/empty value is not allowed for any of the fields Input must be number for Item Number and Item Cost Below is the workflow diagram for the Create Item Process, the check for null values is not included to simplify the diagram. Please make sure to implement all the checks as per requirements. 2.3 Make a Purchase Page This page will list all the items available for sales. For each item, display Item Number, Item Name, Item Cost. Please choose one of the following formats to display the Item list. The page then prompts for user inputs such as item number and employee discount number to make the purchase. Do this until the user answers “NO” for “Another purchase?”. Once all employees have been processed, display the All-Employee Summary Page and give the users option to go back to Menu or Exit the program. Following the flowchart diagram below for the purchasing process. Discounts are based on the number of years worked (2% for each year, maximum 10%) as well as if the employee is a manager (10% more discount on top of the worked year discount) or hourly employee (2% discount). They are also allowed no discount once they have received $200 discount. 2.4. All Employee Summary Page This page will list all the employees of the company. For each employee, display Employee ID, Employee Name, Employee Type, Years Worked, Total Purchased, Employee Discount Number. Please choose one of the following formats to display the Employee list.
Please develop a Python application that meets the requirements described below:
Project Description:
Scenario:
Write a program for a retail store that will allow them to calculate discounts for their employees when they buy items.
Discounts are based on the number of years worked (2% for each year, maximum 10%) as well as if the employee is a manager (10% more discount on top of the worked year discount) or hourly employee (2% discount). They are also allowed no discount once they have received $200 discount.
The program starts asking for the employee discount number to start purchasing. The employee can purchase 1 item at a time. Do this until the user answers “NO” for “Another purchase?”.
Once all employees have been processed, display the All-Employee Summary and give the users option to go back to Menu or Exit the program.
A workflow diagram is provided for each function to help visualize the flow through the program.
Actions:
- Menu Pages:
Create the menus below using a square created in the console by printing dashes (-) and pipes (|) where appropriate. It should look something like this but large enough to cover almost ½ of your computer screen when printed on the console (no need to calculate for the monitor size- just approximate is fine):
————
| |
————
e.g.
———————————————
| |
1- Create Employee |
| |
| |
2- Create Item |
| |
| |
3- Make Purchase |
| |
| |
4-All Employee Summary |
| |
| |
5-Exit |
| |
———————————————
2. Specific Menu Pages to Create:
2.1. Create Employee Page
- Please define a function that asks the user to get the employees’ information and add that to
the list. Whenever the user enters “NO” it will finish getting the employees’ information.
2. Use a 2-dimensional list to create and save the employee information where each item in the list consists of the information of 1 employee:
[Employee ID, Employee Name, Employee Type, Years Worked, Total Purchased, Total Discounts, Employee Discount Number]
E.g.
employee_list = [
[1001, John Alber, hourly, 8, 0, 0, 22737],
[1002, Sarah Rose, manager, 12, 0, 0, 22344],
[1003, Alex Folen, manager, 5, 0, 0, 22957],
[1004, Pola Sahari, hourly, 17, 0, 0, 22488]
]
3.Inputs must be validated to satisfy the following requirements:
-
- Employee ID is unique within the list
- Employee Discount is unique within the list
- Null/empty value is not allowed for any of the fields
- Input must be number for Employee ID, Years Worked, and Employee Discount Number
- Input must be “hourly” or “manager” for Employee Type
- Input is not required for Total Purchased and Total Discounts when the employee is first created in the system, these fields should be assigned with a default value of
Below is the workflow diagram for the Create Employee Process, the check for null values is not included to simplify the diagram. Please make sure to implement all the checks as per requirements.
2.1. Create Item Page
- Please define a function that asks the user to get the items’ information and add that to the Whenever the user enters “NO” it will finish getting the items’ information.
- Use a 2-dimensional list to create and save the item information where each item in the list consists of similar information as follows:
[Item Number, Item Name, Item Cost] E.g.
item_list = [
[11526, Nike shoes, 120],
[11849, Trampoline,180],
[11966, Mercury Bicycle, 150],
[11334, Necklace Set, 80]
]
- Inputs must be validated to satisfy the following requirements:
- Item Number is unique within the list
- Null/empty value is not allowed for any of the fields
- Input must be number for Item Number and Item Cost
Below is the workflow diagram for the Create Item Process, the check for null values is not included to simplify the diagram. Please make sure to implement all the checks as per requirements.
2.3 Make a Purchase Page
This page will list all the items available for sales. For each item, display Item Number, Item Name, Item Cost. Please choose one of the following formats to display the Item list.
The page then prompts for user inputs such as item number and employee discount number to make the purchase. Do this until the user answers “NO” for “Another purchase?”. Once all employees have been processed, display the All-Employee Summary Page and give the users option to go back to Menu or Exit the program. Following the flowchart diagram below for the purchasing process.
Discounts are based on the number of years worked (2% for each year, maximum 10%) as well as if the employee is a manager (10% more discount on top of the worked year discount) or hourly employee (2% discount). They are also allowed no discount once they have received $200 discount.
2.4. All Employee Summary Page
This page will list all the employees of the company. For each employee, display Employee ID, Employee Name, Employee Type, Years Worked, Total Purchased, Employee Discount Number. Please choose one of the following formats to display the Employee list.

Step by step
Solved in 4 steps with 4 images

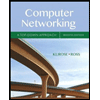
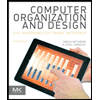
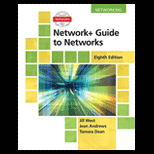
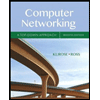
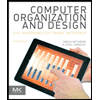
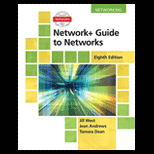
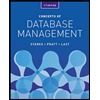
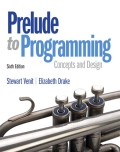
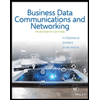