Please explain this C++ program, it doesn't have to be long as long as you explain the important parts of the code do. You can explain it line by line and I will give you a good rating. Thank you Activity name: Trinode Restructuring
Please explain this C++ program, it doesn't have to be long as long as you explain the important parts of the code do. You can explain it line by line and I will give you a good rating. Thank you
Activity name: Trinode Restructuring
#include "node.h"
#include <iostream>
using namespace std;
class BSTree {
node* root;
int size;
node* create_node(int num, node* parent) {
node* n = (node*) malloc( sizeof(node) );
n->element = num;
n->parent = parent;
n->right = NULL;
n->left = NULL;
return n;
}
bool search(node* curr, int num) {
if (curr == NULL) {
return false;
}
if (num == curr->element) {
return true;
}
if (num < curr->element) {
return search(curr->left, num);
}
return search(curr->right, num);
}
node* search_node(node* curr, int num) {
if (num == curr->element) {
return curr;
}
if (num < curr->element) {
if (curr->left != NULL) {
return search_node(curr->left, num);
}
return curr;
}
if (curr->right != NULL) {
return search_node(curr->right, num);
}
return curr;
}
public:
BSTree() {
root = NULL;
size = 0;
}
bool search(int num) {
return search(root, num);
}
bool insert(int num) {
if (root == NULL) {
root = create_node(num, NULL);
size++;
return true;
} else {
node* parent = search_node(root, num);
if (parent->element != num) {
node* newest = create_node(num, parent);
if (parent->element < num) {
parent->right = newest;
} else {
parent->left = newest;
}
size++;
return true;
}
}
return false;
}
// TODO implementation of rotate operation of x where
// |
// y
// \
// x <- curr
void zigleft(node* curr) {
node* x = curr;
node* y = curr->parent;
node* z= y->parent;
y->right = x->left;
if(x->left!=NULL){
x->left->parent=y;
}
if(y!=root){
x->parent = y->parent;
z = y->parent;
if(y==z->right){
z->right=x;
}else{
z->left = x;
}
} else {
x->parent = NULL;
root = x;
}
y->parent = x;
x->left = y;
}
// TODO implementation of rotate operation of x where
// |
// y
// /
// x <- curr
void zigright(node* curr) {
node* x = curr;
node* y= curr->parent;
y->left = x->right;
if(x->right!=NULL){
x->right->parent = y;
}
if(y!=root){
curr->parent = y->parent;
node* z = y->parent;
if(y==z->right){
z->right=curr;
}else{
z->left=curr;
}
}else{
curr->parent=NULL;
root=curr;
}
y->parent = x;
x->right = y;
}
bool restructure(int child) {
node* curr = search_node(root, child);
node* par = curr->parent;
if (!par) {
return false;
}
bool ptoc_right = false;
if (par->right == curr) {
ptoc_right = true;
}
node* gp = par->parent;
if (!gp) {
// y <- root
// \
// x
if (ptoc_right) {
// TODO call to either zigleft or zigright
zigleft(curr);
}
// y <- root
// /
// x
else {
// TODO call to either zigleft or zigright
zigright(curr);
}
return true;
}
bool gtop_right = false;
if (gp->right == par) {
gtop_right = true;
}
// z
// \
// y
// \
// x
if (gtop_right && ptoc_right) {
// TODO call to either zigleft or zigright or both
zigleft(par);
}
// z
// \
// y
// /
// x
else if (gtop_right && !ptoc_right) {
// TODO call to either zigleft or zigright or both
zigright(curr);
zigleft(curr);
}
// z
// /
// y
// /
// x
else if (!gtop_right && !ptoc_right) {
// TODO call to either zigleft or zigright or both
zigright(par);
}
// z
// /
// y
// \
// x
else {
// TODO call to either zigleft or zigright or both
zigleft(curr);
zigright(curr);
}
return true;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

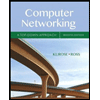
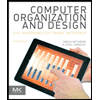
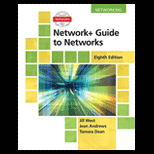
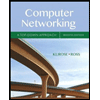
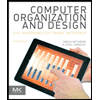
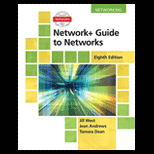
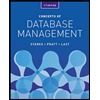
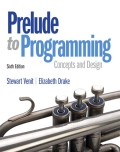
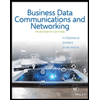