Please Explain this code Step by Step (How Code Works). #include #include #include char password[10] = "1234"; struct library { int id; char title[40]; char author[20]; float price; }; //dynamic books struct library *b[100]; int num = 0; void Add() { int count = 0; printf("How many books' info do you want to enter? "); scanf(" %d", &count); for (int i = 0; i < count; i++) { b[num] = (struct library *)malloc(sizeof(struct library)); //local variables int id; char title[40]; char author[20]; float price; printf("Enter the following information about the book:\n"); printf("Enter ID: "); scanf("%d%*c", &id); fflush(stdin); fflush(stdin); printf("Enter title: "); scanf("%[^\n]%*c", title); printf("Enter author's name: "); scanf("%[^\n]%*c", author); printf("Enter price(in Tk): "); scanf("%f", &price); //check if information exists already for (int i = 0; i < num; i++) { if (b[i]->id == id) { printf("Book with id %d already present in the library", id); return; } } b[num]->id = id; strcpy(b[num]->title, title); strcpy(b[num]->author, author); b[num]->price = price; num++; } } void Disp() { printf("\tID\tName\tAuthor\tPrice(Tk)\n"); for (int i = 0; i < num; i++) { printf("\t%d\t%s\t%s\t%f\n", b[i]->id, b[i]->title, b[i]->author, b[i]->price); } }
Please Explain this code Step by Step (How Code Works).
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
char password[10] = "1234";
struct library
{
int id;
char title[40];
char author[20];
float price;
};
//dynamic books
struct library *b[100];
int num = 0;
void Add()
{
int count = 0;
printf("How many books' info do you want to enter? ");
scanf(" %d", &count);
for (int i = 0; i < count; i++)
{
b[num] = (struct library *)malloc(sizeof(struct library));
//local variables
int id;
char title[40];
char author[20];
float price;
printf("Enter the following information about the book:\n");
printf("Enter ID: ");
scanf("%d%*c", &id);
fflush(stdin);
fflush(stdin);
printf("Enter title: ");
scanf("%[^\n]%*c", title);
printf("Enter author's name: ");
scanf("%[^\n]%*c", author);
printf("Enter price(in Tk): ");
scanf("%f", &price);
//check if information exists already
for (int i = 0; i < num; i++)
{
if (b[i]->id == id)
{
printf("Book with id %d already present in the library", id);
return;
}
}
b[num]->id = id;
strcpy(b[num]->title, title);
strcpy(b[num]->author, author);
b[num]->price = price;
num++;
}
}
void Disp()
{
printf("\tID\tName\tAuthor\tPrice(Tk)\n");
for (int i = 0; i < num; i++)
{
printf("\t%d\t%s\t%s\t%f\n", b[i]->id, b[i]->title, b[i]->author, b[i]->price);
}
}
void Count()
{
printf("\nNo of books avalable in the library = %d\n", num);
}
void List()
{
char str[20];
printf("Enter the author's name: ");
scanf("%s", str);
for (int i = 0; i < num; i++)
{
if (strcmp(str, b[i]->author) == 0)
printf("\n\t%d\t%s\t%s\t%f\n", b[i]->id, b[i]->title, b[i]->author, b[i]->price);
}
}
int validateLibrarion()
{
char passwd[10];
printf("Enter password: ");
scanf("%s", passwd);
if (strcmp(passwd, password) == 0)
{
printf("\nPassword Authentication successful..\n");
return 1;
}
else
{
printf("Incorrect password....\n");
return 0;
}
}
int main()
{
int option = 0;
do
{
printf("\n\nWelcome to the library\nPlease Select an Option: \n");
printf("-----------------------------------------------------------\n");
printf("1.Add book details\n2.Display book details\n3.List all books of a given author\n4.Show total no. of books in the library.\n5.Exit\n");
printf("-----------------------------------------------------------\n");
scanf("%d", &option);
switch (option)
{
case 1:
if (validateLibrarion())
Add();
break;
case 2:
Disp();
break;
case 3:
List();
break;
case 4:
Count();
break;
}
} while (option != 5);
return 0;
}

Step by step
Solved in 10 steps with 2 images

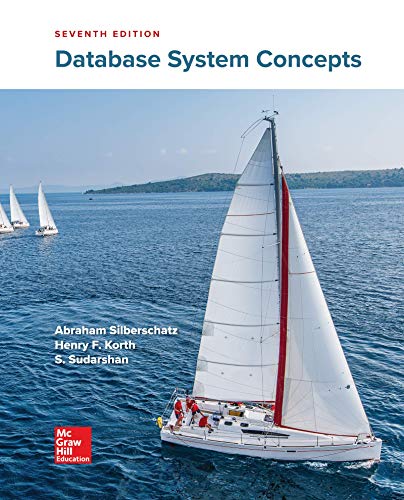
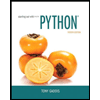
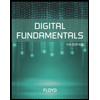
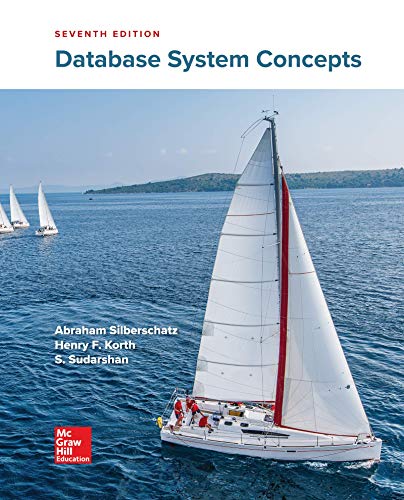
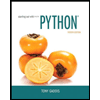
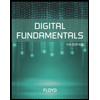
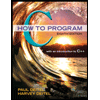
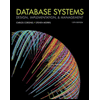
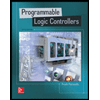