Please fill in the blanks for C. /* Part 1 will count how many times a character appears in a sentence. Part 2 will remove the character of interest from the sentence. Note: I also use different typs of loop to show it doesnt have to be the same type of loop everytime. */ __1__<__2__> //add the library to use printf and scanf functions __3__<__4__> //add the library to use boolean #__5__ LEN 1000 //create a constant max LEN // Add function headers __6__ search_char(__7__ c1, __8__ c2); __9__ get_occurrences(__10__ str[], __11__ search); __12__ scan_string(__13__ str[]); __14__ remove_char_from_str(__15__ s1[], __16__ s2[], __17__ search); //PART 1 + 2 /*This function returns an integer 1 is the two character are the same and 0 otherwise. Normally, we returns -1 if they are not the same, but in this case, this number will be added to the counts from get_occrrences function to return the number of times a character appears for Part 1. */ __18__ search_char(__19__ c1, __20__ c2) { //Using ternary to check and return if the 2 characters are the same __21__ (c1 __22__ c2) __23__ __24__ __25__ __26__; } //PART 1 /*This function goes through the string and add 1 to the total count if currect character is the same as the search character, and add 0 otherwise. Function returns a count.*/ __27__ get_occurrences(__28__ str[], __29__ search) { int num = 0; //keep track of # of occurrences //Go through the string with for loop for(int i = __30__ ; __31__ __32__ __33__; i++) { __34__ += search_char(__35__[__36__], search); //update the count } __37__ __38__; //return the count } //Part 1 /*This function scans the users input. No return needed.*/ __39__ scan_string(__40__ str[]) { printf("Enter you string here: "); int strIdx = 0; //index for str char curChar; //Go through the string with do-while loop do{ curChar = __41__; //get a character input from user __42__[__43__] = __44__; //add user input to array strIdx++; //update index }while(__45__ __46__ __47__); //condition to run //End the string __48__[__49__] = __50__; } //Part 2 /*This function gets 2 strings and 1 character. Go through the string, if currect character is not equal to search character, add that to the new string. This is essentially the same as ignorning the search character (remove it from original string).*/ __51__ remove_char_from_str(__52__ s1[], __53__ s2[], __54__ search) { //i1 and i2 are the indices for s1 and s2, repectively int i1 = 0, i2 = 0; //Loop through the original string with while loop while(__55__[__56__] != __57__) //condition to run { int isFound = search_char(__58__[__59__], __60__); //compare character from original string with search character if(__61__) //condition to add a character to result string. //No space (cannot put .. == ..., just 1 opeartor and a variable) { __62__[__63__] = __64__[__65__]; //copy the character over to the new string. Check main to know which one is new and which is original __66__ ++; //update index } __67__ ++; //update index } __68__[__69__] = __70__; //end the substring } int main() { char myStr[LEN], search; int counts = 0; // Get string printf("Hello. Give me a string and a character and I'll count how many times the character appears.\n"); scan_string(myStr); //Get character printf("Got your string. Enter the character: "); scanf(" %c", &search); //call function get_occurrences to get back the number of times a character appears in the string counts = get_occurrences(myStr,search); printf("You inputted string : \"%s\", and character \'%c\' appears %i times.\n", myStr, search, counts); //create a new string to save the result of string after removing the character char subStr[LEN] = ""; printf("\n\nRemoving character \'%c\' from your string.\n", search); /*remove_char_from_str() function gets an original string, an null string to save the result, and the character to remove. Go through each character, compare current character with search character. If they aren't the same, save the character to the result substring.*/ remove_char_from_str(myStr,subStr,search); printf("Your new string is: \"%s\"\n", subStr); return 0; }
Please fill in the blanks for C.
/*
Part 1 will count how many times a character appears in a sentence.
Part 2 will remove the character of interest from the sentence.
Note: I also use different typs of loop to show it doesnt have to be the same type of loop everytime.
*/
__1__<__2__> //add the library to use printf and scanf functions
__3__<__4__> //add the library to use boolean
#__5__ LEN 1000 //create a constant max LEN
// Add function headers
__6__ search_char(__7__ c1, __8__ c2);
__9__ get_occurrences(__10__ str[], __11__ search);
__12__ scan_string(__13__ str[]);
__14__ remove_char_from_str(__15__ s1[], __16__ s2[], __17__ search);
//PART 1 + 2
/*This function returns an integer 1 is the two character are the same
and 0 otherwise.
Normally, we returns -1 if they are not the same, but in this case,
this number will be added to the counts from get_occrrences function
to return the number of times a character appears for Part 1. */
__18__ search_char(__19__ c1, __20__ c2)
{
//Using ternary to check and return if the 2 characters are the same
__21__ (c1 __22__ c2) __23__ __24__ __25__ __26__;
}
//PART 1
/*This function goes through the string and add 1 to the total count
if currect character is the same as the search character, and add 0 otherwise.
Function returns a count.*/
__27__ get_occurrences(__28__ str[], __29__ search)
{
int num = 0; //keep track of # of occurrences
//Go through the string with for loop
for(int i = __30__ ; __31__ __32__ __33__; i++)
{
__34__ += search_char(__35__[__36__], search); //update the count
}
__37__ __38__; //return the count
}
//Part 1
/*This function scans the users input. No return needed.*/
__39__ scan_string(__40__ str[])
{
printf("Enter you string here: ");
int strIdx = 0; //index for str
char curChar;
//Go through the string with do-while loop
do{
curChar = __41__; //get a character input from user
__42__[__43__] = __44__; //add user input to array
strIdx++; //update index
}while(__45__ __46__ __47__); //condition to run
//End the string
__48__[__49__] = __50__;
}
//Part 2
/*This function gets 2 strings and 1 character.
Go through the string, if currect character is not equal to search character, add that to the new string.
This is essentially the same as ignorning the search character (remove it from original string).*/
__51__ remove_char_from_str(__52__ s1[], __53__ s2[], __54__ search)
{
//i1 and i2 are the indices for s1 and s2, repectively
int i1 = 0, i2 = 0;
//Loop through the original string with while loop
while(__55__[__56__] != __57__) //condition to run
{
int isFound = search_char(__58__[__59__], __60__); //compare character from original string with search character
if(__61__) //condition to add a character to result string.
//No space (cannot put .. == ..., just 1 opeartor and a variable)
{
__62__[__63__] = __64__[__65__]; //copy the character over to the new string. Check main to know which one is new and which is original
__66__ ++; //update index
}
__67__ ++; //update index
}
__68__[__69__] = __70__; //end the substring
}
int main()
{
char myStr[LEN], search;
int counts = 0;
// Get string
printf("Hello. Give me a string and a character and I'll count how many times the character appears.\n");
scan_string(myStr);
//Get character
printf("Got your string. Enter the character: ");
scanf(" %c", &search);
//call function get_occurrences to get back the number of times a character appears in the string
counts = get_occurrences(myStr,search);
printf("You inputted string : \"%s\", and character \'%c\' appears %i times.\n", myStr, search, counts);
//create a new string to save the result of string after removing the character
char subStr[LEN] = "";
printf("\n\nRemoving character \'%c\' from your string.\n", search);
/*remove_char_from_str() function gets an original string, an null string to save the result,
and the character to remove.
Go through each character, compare current character with search character.
If they aren't the same, save the character to the result substring.*/
remove_char_from_str(myStr,subStr,search);
printf("Your new string is: \"%s\"\n", subStr);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

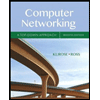
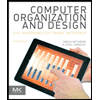
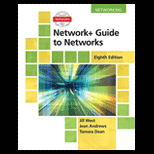
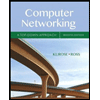
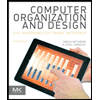
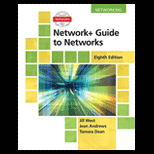
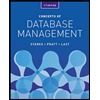
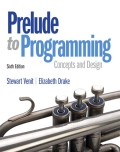
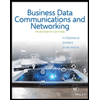