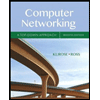
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Problem 2 (Cipher module)
• Create a Python file named cipher_module.py with functions to encode and decode
string inputs using the basic Caesar cipher (see here for more information
https://en.wikipedia.org/wiki/Caesar_cipher). This cipher is a basic encryption
technique that supports encoding and decoding operations on strings.
• The encoding operation replaces each character in a string with another character
some fixed number (shift) of positions down the ASCII table. The string can be
encoded using any characters on the ASCII table and no need to use the circular list
(where ‘Z’ is followed by ‘A’) image shown below. Your module must have a function
named encode() that takes a string and an integer shift (can be positive or negative)
as parameters and returns the encoded string using the shift value. This module must
• Create a Python file named cipher_module.py with functions to encode and decode
string inputs using the basic Caesar cipher (see here for more information
https://en.wikipedia.org/wiki/Caesar_cipher). This cipher is a basic encryption
technique that supports encoding and decoding operations on strings.
• The encoding operation replaces each character in a string with another character
some fixed number (shift) of positions down the ASCII table. The string can be
encoded using any characters on the ASCII table and no need to use the circular list
(where ‘Z’ is followed by ‘A’) image shown below. Your module must have a function
named encode() that takes a string and an integer shift (can be positive or negative)
as parameters and returns the encoded string using the shift value. This module must
Page 3 of 4
also have a second function name decode() that takes an encoded string and an
integer shift as parameters and returns the decoded string. The decoding operation
is the reverse of encoding.
• Import your cipher_module module in another Python program named p2.py and
use the functions provided by the module to test the encoding/decoding operations
on some sample strings using different shift values.
• Submit both cipher_module and p2.py.
• Hint: use built-in ord() function to convert a character to ascii integer and chr()
function to convert the ascii to a character.
Sample run:
cipher_module.encode(abde, 2) returns cdfg
cipher_module.decode(cdfg, 2) returns abde
cipher_module.encode(eebcd, 3) returns hhefg
cipher_module.decode(hhefg, 3) returns eebcd
cipher_module.encode(hhefg, -2) returns ffcde
cipher_module.decode(ffcde, -2) returns hhefg
also have a second function name decode() that takes an encoded string and an
integer shift as parameters and returns the decoded string. The decoding operation
is the reverse of encoding.
• Import your cipher_module module in another Python program named p2.py and
use the functions provided by the module to test the encoding/decoding operations
on some sample strings using different shift values.
• Submit both cipher_module and p2.py.
• Hint: use built-in ord() function to convert a character to ascii integer and chr()
function to convert the ascii to a character.
Sample run:
cipher_module.encode(abde, 2) returns cdfg
cipher_module.decode(cdfg, 2) returns abde
cipher_module.encode(eebcd, 3) returns hhefg
cipher_module.decode(hhefg, 3) returns eebcd
cipher_module.encode(hhefg, -2) returns ffcde
cipher_module.decode(ffcde, -2) returns hhefg
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
how do i get the same output because i followed the instrution and the code but i am not able to get the same result as the question require the creation of both the cipher_module and p2.py?
Solution
by Bartleby Expert
Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
how do i get the same output because i followed the instrution and the code but i am not able to get the same result as the question require the creation of both the cipher_module and p2.py?
Solution
by Bartleby Expert
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- C programming - (image attached ) Write a programme called pgmTile which takes 3 arguments, an input file (either ASCII or binary), an integer factor n, and an output file name template. It should divide the input file into nxn smaller images corresponding to parts of the image. For example, suppose that the invocation is: pgmTile sampleFile.pgm 3 sampleFile_<row>_<column>.pgm then the programme should read in sampleFile.pgm and output 9 smaller images, named: sampleFile_0_0.pgm sampleFile_0_1.pgm sampleFile_0_2.pgm sampleFile_1_0.pgm sampleFile_1_1.pgm sampleFile_1_2.pgm sampleFile_2_0.pgm sampleFile_2_1.pgm sampleFile_2_2.pgm i.e. substituting the relative position of the subimage in the larger image into the <row> and <column> tags in the template namearrow_forwardpls help NOTE! it must be in ARM assembly languagearrow_forwardCan you do 1 and 2 please? (C++ language) 1- Please implement the Word Counting program that is shown in the pictures attached. 2- Also in the same program count the number of words longer than 3 letters.arrow_forward
- Create the following in C++arrow_forwardPython, input file has the following structure (number, then animal each on a line): 4Cows5Camels1Peacock3Whales4Lions2Scorpions Two output files are required:first output_file: sort by (dictionary) key but those sharing the same key should be listed on the same line with a ";" separated 1: Peacock2: Scorpions3: Whales4: Cows; lions ------------------------------> separated by ';' because they share the same key 45: Camels second output_file:Only values are sorted as follows and written to file: CamelsCowsLions PeacockScorpionsWhales Thank youarrow_forwardIn standard C programming pleasearrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
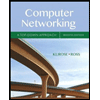
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
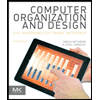
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
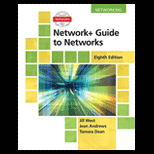
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
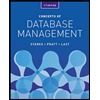
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
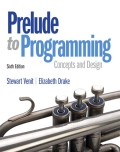
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
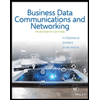
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY