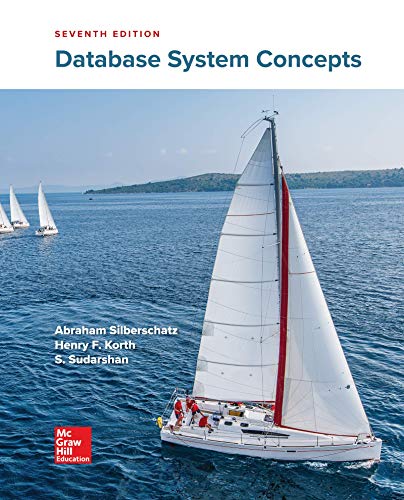
Concept explainers
Please help me
Create mocha testing unit using the code
const url = "http://localhost:3000/document";
let undoStack = [];
let redoStack = [];
function sendDataToServer(data) {
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ content: data })
})
.then(response => response.json())
.then(data => console.log('Success:', data))
.catch((error) => console.error('Error:', error));
}
function formatText(command) {
document.execCommand(command, false, null);
}
function changeColor() {
const color = prompt("Enter text color code:");
document.execCommand("foreColor", false, color);
}
function changeFont() {
const font = prompt("Enter font:");
document.execCommand("fontName", false, font);
}
function changeFontSize() {
const size = prompt("Enter font size:");
document.execCommand("fontSize", false, size);
}
function clearText() {
document.getElementById("editor").innerHTML = "";
}
function toUpperCase() {
const selectedText = window.getSelection().toString();
document.execCommand("insertText", false, selectedText.toUpperCase());
}
function toLowerCase() {
const selectedText = window.getSelection().toString();
document.execCommand("insertText", false, selectedText.toLowerCase());
}
function highlightText() {
const color = prompt("Enter highlight color code:");
document.execCommand("hiliteColor", false, color);
}
function createTable() {
const rows = prompt("Enter the number of rows:");
const cols = prompt("Enter the number of columns:");
let tableHTML = "<table border='1'>";
for (let i = 0; i < rows; i++) {
tableHTML += "<tr>";
for (let j = 0; j < cols; j++) {
tableHTML += "<td>Cell</td>";
}
tableHTML += "</tr>";
}
tableHTML += "</table>";
document.execCommand("insertHTML", false, tableHTML);
}
function increaseImageSize() {
const selectedImage = document.querySelector('img.resizable');
if (selectedImage) {
const currentWidth = selectedImage.width;
selectedImage.style.width = (currentWidth * 1.2) + 'px';
}
}
function decreaseImageSize() {
const selectedImage = document.querySelector('img.resizable');
if (selectedImage) {
const currentWidth = selectedImage.width;
selectedImage.style.width = (currentWidth * 0.8) + 'px';
}
}
function insertImage() {
const imageUrl = prompt("Enter the image URL:");
if (imageUrl) {
const imgHTML = "<img src='" + imageUrl + "' alt='User Image' class='resizable'>";
document.execCommand("insertHTML", false, imgHTML);
}
}
function undo() {
if (undoStack.length > 0) {
redoStack.push(document.getElementById("editor").innerHTML);
document.getElementById("editor").innerHTML = undoStack.pop();
}
}
function redo() {
if (redoStack.length > 0) {
undoStack.push(document.getElementById("editor").innerHTML);
document.getElementById("editor").innerHTML = redoStack.pop();
}
}
function autoSave() {
const currentContent = document.getElementById("editor").innerHTML;
undoStack.push(currentContent);
if (undoStack.length > 10) {
undoStack.shift();
}
// Implement autosave functionality here (e.g., send currentContent to server for saving)
}
function updateTextInfo() {
const editorContent = document.getElementById("editor").innerText;
const wordCount = editorContent.trim().split(/\s+/).filter(word => word.length > 0).length;
const characterCount = editorContent.replace(/[\n\s]+/g, '').length;
const sentenceCount = editorContent.split(/[.!?]+/).filter(sentence => sentence.length > 0).length;
const paragraphCount = editorContent.split('\n\n').filter(para => para.trim() !== '').length;
document.getElementById("wordCount").innerText = "Word Count: " + wordCount;
document.getElementById("charCount").innerText = "Character Count: " + characterCount;
document.getElementById("sentenceCount").innerText = "Sentence Count: " + sentenceCount;
document.getElementById("paraCount").innerText = "Paragraph Count: " + paragraphCount;
});

Step by stepSolved in 2 steps

- Explain the functionality of each line of code in the provided C# scripts- using System.Collections;using System.Collections.Generic;using UnityEngine;using UnityEngine.UI; public class Healthbar : MonoBehaviour{ [SerializeField] private Health playerHealth; [SerializeField] private Image totalHealthbar; [SerializeField] private Image currentHealthbar; private void Start() { totalHealthbar.fillAmount = playerHealth.currentHealth / 10; } private void Update() { currentHealthbar.fillAmount = playerHealth.currentHealth / 10; }} using System.Collections;using System.Collections.Generic;using UnityEngine;using UnityEngine.SceneManagement; public class Health : MonoBehaviour{ [SerializeField] private float startingHealth; public float currentHealth { get; private set; } private Animator anim; private void Awake() { currentHealth = startingHealth; anim = GetComponent<Animator>(); } public void…arrow_forwarddef get_nearest_station(my_latitude: float, my_longitude: float, stations: List['Station']) -> int: "''Return the id of the station from stations that is nearest to the location given by my_latidute and my_longitude. In the case of a tie, return the ID of the last station in stations with that distance. Preconditions: len(stations) > 1 » get_nearest_station(43.671134, -79.325164, SAMPLE_STATIONS) 7571 » get_nearest_station(43.674312, -79.299221, SAMPLE_STATIONS) 7486arrow_forwarddef upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0arrow_forward
- Create class Node in a file named Node.java. This class has the following properties: Public fields previous and next, pointing to the previous and next nodes in the list, respectively. Private field data, defined as a reference to an object of any type. Public constructor that takes the node’s data as an argument. Public function GetData() that returns the node’s data.arrow_forwardWrite the lines of code to insert the key (book's ISBN) and value ("book") pair into "my_hash_table".arrow_forwardWhat are the Javadoc comments for each class? I am strugglingarrow_forward
- help me java web (Can you guide the solution?) 1)file db DbContext public class DBContext { protected Connection connection; public DBContext() { try { String user = "sa"; String pass = "123456789"; String url = "jdbc:sqlserver://MSI:1433;databaseName=MyOrder"; Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); connection = DriverManager.getConnection(url, user, pass); } catch (ClassNotFoundException | SQLException ex) { Logger.getLogger(DBContext.class.getName()).log(Level.SEVERE, null, ex); } } } 2)public class DBContext1 { public Connection getConnection()throws Exception { String url = "jdbc:sqlserver://"+serverName+":"+portNumber +";databaseName="+dbName; Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); return DriverManager.getConnection(url, userID, password); } private final String serverName =…arrow_forwardWhen the cache cannot handle a request, the CPU sends the data back to main memory through the write buffer. We need your advice on how to proceed.arrow_forwarddef get_nearest_station(my_latitude: float, my_longitude: float, stations: List['Station']) -> int: """Return the id of the station from stations that is nearest to the location given by my_latidute and my_longitude. In the case of a tie, return the ID of the last station in stations with that distance. Preconditions: len(stations) > 1 >>> get_nearest_station(43.671134, -79.325164, SAMPLE_STATIONS) 7571 >>> get_nearest_station(43.674312, -79.299221, SAMPLE_STATIONS) 7486 """arrow_forward
- Design a class that acquires the JSON string from question #1 and converts it to a class data member dictionary. Your class produces data sorted by key or value but not both. Provide searching by key capabilities to your class. Provide string functionality to convert the dictionary back into a JSON string. question #1: import requestsfrom bs4 import BeautifulSoupimport json class WebScraping: def __init__(self,url): self.url = url self.response = requests.get(self.url) self.soup = BeautifulSoup(self.response.text, 'html.parser') def extract_data(self): data = [] lines = self.response.text.splitlines()[57:] # Skip the first 57 lines for line in lines: if line.startswith('#'): # Skip comment lines continue values = line.split() row = { 'year': int(values[0]), 'month': int(values[1]), 'decimal_date': float(values[2]),…arrow_forwardin this android app package com.example.myapplication;import androidx.appcompat.app.AppCompatActivity;import android.os.Bundle;import android.widget.ListView;public class PlayerActivity2 extends AppCompatActivity {ListView simpleList;String SerialNo[] = {"1", "2", "3", "4", "5", "6","7","8","9","10"};int flags[] = {R.drawable.image1, R.drawable.image2, R.drawable.image3, R.drawable.image4, R.drawable.image5, R.drawable.image6, R.drawable.image7, R.drawable.image8, R.drawable.image9, R.drawable.image10};String Names[] = {"mmm", "nnn", "aaa.", "bbb", "ccc", "ddd","eee jk"," ijk","Virgil jk","gil jklk"};String Score[] = {"1", "2","3", "5", "4", "3","5","5","5","5"};@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity2);simpleList = (ListView)findViewById(R.id.simpleListView);//ArrayAdapter<String> arrayAdapter = new ArrayAdapter<String>(this, R.layout.activity_listview, R.id.textView,…arrow_forwardWhat do the urlretrieve and urlopen functions do?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
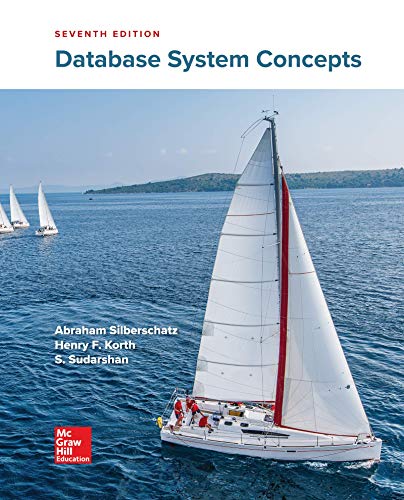
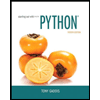
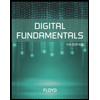
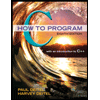
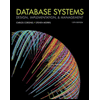
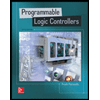