Please help me! The program is able to accept 3 input scores from the user. The average of the 3 scores can also be obtained. How do I show thw highest score and the lowest score from the 3 input scores. strictly use this program below import csv # Define global variables student_fields = [' Student No.', ' Name', ' Age', ' Email address'] math = ['Math'] physics = ['Physics'] computer = ['Computer'] average = ['Average'] student_database = 'students.csv' def display_menu(): print(" Welcome to Student Management System ") print("1. Add New Student") print("2. View Students") print("3. Search Student") print("4. Update Student") print("5. Delete Student") print("6. Quit") def add_student(): print("Add Student Information") global student_fields global math global physics global computer
Please help me! The program is able to accept 3 input scores from the user. The average of the 3 scores can also be obtained. How do I show thw highest score and the lowest score from the 3 input scores.
strictly use this program below
import csv
# Define global variables
student_fields = [' Student No.', ' Name', ' Age', ' Email address']
math = ['Math']
physics = ['Physics']
computer = ['Computer']
average = ['Average']
student_database = 'students.csv'
def display_menu():
print(" Welcome to Student Management System ")
print("1. Add New Student")
print("2. View Students")
print("3. Search Student")
print("4. Update Student")
print("5. Delete Student")
print("6. Quit")
def add_student():
print("Add Student Information")
global student_fields
global math
global physics
global computer
global average
global student_database
student_data = []
for field in student_fields:
value = input("Enter" + field + ": ")
student_data.append(value)
for m in math:
m = int(input("Enter your math grade: "))
student_data.append(m)
for p in physics:
p = int(input("Enter your physics grade: "))
student_data.append(p)
for c in computer:
c = int(input("Enter your computer grade: "))
student_data.append(c)
for ave in average:
ave = float((p + c + m)/3)
student_data.append(ave)
with open(student_database, "a", encoding="utf-8") as f:
writer = csv.writer(f)
writer.writerows([student_data])
print("Data saved successfully")
input("Press any key to continue: ")
return
def grades():
global student_fields
global math
global physics
global computer
global average
global student_database
with open(student_database, "r", encoding="utf-8") as f:
reader = csv.reader(f)
for line in reader:
print(line)
def view_students():
global student_fields
global math
global physics
global computer
global average
global student_database
print("Student Records")
with open(student_database, "r", encoding="utf-8") as f:
reader = csv.reader(f)
for x in student_fields:
print(x, end='\t |')
for m in math:
print(m, end='\t |')
for p in physics:
print(p, end='\t |')
for c in computer:
print(c, end='\t |')
for a in average:
print(a, "{.2f}" , end='\t |')
for row in reader:
for item in row:
print(item, end="\t |")
print("\n")
input("Press any key to continue: ")
def search_student():
global student_fields
global math
global physics
global computer
global average
global student_database
print("Search Student")
roll = input("Enter roll no. to search: ")
with open(student_database, "r", encoding="utf-8") as f:
reader = csv.reader(f)
for row in reader:
if len(row) > 0:
if roll == row[0]:
print("Student Found")
print("Student No.: ", row[0])
print("Name: ", row[1])
print("Age: ", row[2])
print("Email Address: ", row[3])
print("Math: ", row[4])
print("Physics: ", row[5])
print("Computer: ", row[6])
print("Average: ", row[7])
break
else:
print("Student No. not found in our
input("Press any key to continue: ")
def update_student():
global student_fields
global math
global physics
global computer
global average
global student_database
print("Update Student")
roll = input("Enter Student No. to update: ")
index_student = None
updated_data = []
with open(student_database, "r", encoding="utf-8") as f:
reader = csv.reader(f)
counter = 0
for row in reader:
if len(row) > 0:
if roll == row[0]:
index_student = counter
print("Student Found.: at index ",index_student)
student_data = []
for field in student_fields:
value = input("Enter " + field + ": ")
student_data.append(value)
for m in math:
m = int(input("Enter your math grade: "))
student_data.append(m)
for p in physics:
p = int(input("Enter your physics grade: "))
student_data.append(p)
for c in computer:
c = int(input("Enter your computer grade: "))
student_data.append(c)
for ave in average:
ave = float((p + c + m)/3)
student_data.append(ave)
updated_data.append(student_data)
else:
updated_data.append(row)
counter += 1
# Check if the record is found or not
if index_student is not None:
with open(student_database, "w", encoding="utf-8") as f:
writer = csv.writer(f)
writer.writerows(updated_data)
else:
print("Student No. not found in our database")
print("Data has been updated.")
input("Press any key to continue: ")
def delete_student():
global student_fields
global math
global physics
global computer
global average
global student_database
print("Delete Student")
roll = input("Enter Student No. to delete: ")
student_found = False
updated_data = []
with open(student_database, "r", encoding="utf-8") as f:
reader = csv.reader(f)
counter = 0
for row in reader:
if len(row) > 0:
if roll != row[0]:
updated_data.append(row)
counter += 1
else:
student_found = True
if student_found is True:
with open(student_database, "w", encoding="utf-8") as f:
writer = csv.writer(f)
writer.writerows(updated_data)
print("Student No. ", roll, "deleted successfully")
else:
print("Student No. not found in our database")
while True:
display_menu()
choice = input("Enter your choice: ")
if choice == '1' :
add_student()
elif choice == '2' :
view_students()
elif choice == '3' :
search_student()
elif choice == '4' :
update_student()
elif choice == '5' :
delete_student()
else:
break
print(" Thank you for using our system")

Step by step
Solved in 2 steps

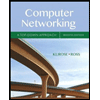
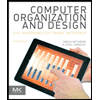
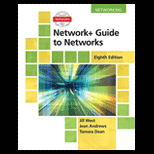
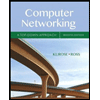
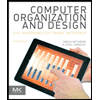
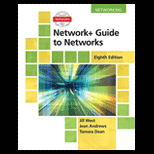
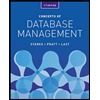
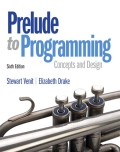
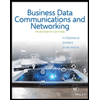