Please help me with this one! bagIntersection: The intersection of two bags is a new bag containing the entries that occur in both of the original bags. Design and specify a method intersection for the ArrayBag that returns as a new bag the intersection of the bag receiving the call to the method and the bag that is the method's parameter. The method signature is: ArrayBag bagIntersection(const ArrayBag &otherBag) const; Note that the intersection of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the intersection of these bags contains x two times. The intersection does not affect the contents of the original bags.
Please help me with this one!
bagIntersection: The intersection of two bags is a new bag containing the entries that occur in both of the original bags. Design and specify a method intersection for the ArrayBag that returns as a new bag the intersection of the bag receiving the call to the method and the bag that is the method's parameter. The method signature is:
ArrayBag<ItemType> bagIntersection(const ArrayBag<ItemType> &otherBag) const;
Note that the intersection of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the intersection of these bags contains x two times. The intersection does not affect the contents of the original bags.
Here is the all of the file:
ArrayBag.cpp:
#include <string>
#include "ArrayBag.h"
template <class T>
ArrayBag<T>::ArrayBag() : itemCount(0) {
// itemCount = 0;
}
template <class T>
ArrayBag<T>::ArrayBag(const ArrayBag& orig) {
itemCount = orig.itemCount;
for (int i = 0; i < orig.itemCount; i++) {
items[i] = orig.items[i];
}
}
template <class T>
ArrayBag<T>::~ArrayBag() {
}
//interface methods
template <class T>
int ArrayBag<T>::getCurrentSize() const {
return itemCount;
}
template <class T>
bool ArrayBag<T>::isEmpty() const {
return (itemCount == 0);
}
template <class T>
bool ArrayBag<T>::add(const T& anItem) {
bool hasRoom = (itemCount < SIZE);
if (hasRoom) {
items[itemCount] = anItem;
itemCount++;
}
return hasRoom;
}
template <class T>
bool ArrayBag<T>::remove(const T& anItem) {
int location = find(anItem);
bool canRemove = (!isEmpty() && location > -1);
if (canRemove) {
//remove
itemCount--;
items[location] = items[itemCount];
}
return canRemove;
}
template <class T>
void ArrayBag<T>::clear() {
itemCount = 0;
}
template <class T>
int ArrayBag<T>::getFreqOf(const T& anItem) const {
int freq = 0;
for (int i = 0; i < itemCount; i++) {
if (items[i] == anItem) {
freq++;
}
}
return freq;
}
template <class T>
bool ArrayBag<T>::contains(const T& anItem) const {
bool found = false;
// for (int i = 0; i < itemCount; i++) {
// if (items[i] == anItem)
// found = true;
// }
int i = 0;
while (i < itemCount && !found) {
if (items[i] == anItem)
found = true;
i++;
}
return found;
// return (getFreqOf(anItem) > 0);
}
template <class T>
void ArrayBag<T>::prtItems() const {
for (int i = 0; i < itemCount; i++) {
std::cout << items[i] << "\t";
}
std::cout << std::endl;
}
template <class T>
int ArrayBag<T>::find(const T& anItem) {
int index = -1;
int i = 0;
while (i < itemCount && index < 0) {
if (items[i] == anItem)
index = i;
i++;
}
return index;
}
//homework methods
template <class T>
ArrayBag<T> ArrayBag<T>::bagUnion(const ArrayBag<T>& otherBag) const {
}
ArrayBag.h
#ifndef ARRAYBAG_H
#define ARRAYBAG_H
#include <string>
#include "BagADT.h"
template <class T>
class ArrayBag : BagADT<T> {
public:
ArrayBag();
ArrayBag(const ArrayBag& orig);
virtual ~ArrayBag();
//interface methods
int getCurrentSize() const;
bool isEmpty() const;
bool add (const T& anItem);
bool remove (const T& anItem);
void clear();
int getFreqOf(const T& anItem) const;
bool contains(const T& anItem) const;
//other methods
void prtItems() const;
//homework methods
ArrayBag<T> bagUnion(const ArrayBag<T> &otherBag) const;
private:
static const int SIZE = 20;
T items[SIZE];
int itemCount;
/**
* Searches the array for anItem and returns its location
* @param anItem item to search for
* @return index of the item in the array; returns -1 if not found
*/
int find(const T& anItem);
};
#include "ArrayBag.cpp"
#endif /* ARRAYBAG_H */
BagADT
#ifndef BAGADT_H
#define BAGADT_H
#include <string>
template <class T>
class BagADT {
public:
/**
* Returns the number of items in the bag
* @return the number of items in the bag
*/
virtual int getCurrentSize() const = 0;
/**
* Tests to see if the bag is empty
* @return true if the bag is empty, false if it contains items
*/
virtual bool isEmpty() const = 0;
/**
* Adds an item to the bag in no particular order
* @param anItem item to be added
* @return true if successfully added
*/
virtual bool add (const T& anItem) = 0;
/**
* Removes an item from the bag
* @param anItem the item to be removed
* @return true if successfully removed
*/
virtual bool remove (const T& anItem) = 0;
/**
* Removes all items from the bag
*/
virtual void clear() = 0;
/**
* Counts the number of times an item is in the bag
* @param anItem items to be counted
* @return the number of times an item is in the bag
*/
virtual int getFreqOf(const T& anItem) const = 0;
/**
* Tests to see if an item is in the bag
* @param anItem item to test for
* @return true if the bag contains the item
*/
virtual bool contains(const T& anItem) const = 0;
};
#endif /* BAGADT_H */

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

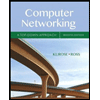
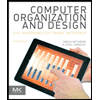
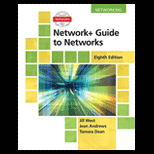
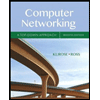
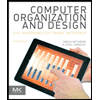
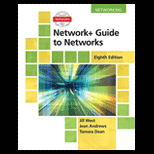
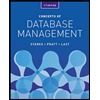
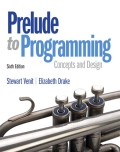
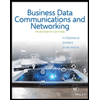