Counts the number of occurrences of the given element in the given array. * Oparam array to search * eparam element to search for * Breturn number of times element is in array publie int howMany (int () array, int element) // TODO Implement method return 0: In addition, you will write unit tests to test your method implementations. Each unit test method has been defined for you, including some test cases. First make sure you pass all of the provided tests, then write additional and distinct test cases for each unit test method. For example, we have defined a "testHowMany" method for you (see below) which tests the "howMany" method. Pass the tests provided then write additional tests where it says "/ TODO". You'll do this for each unit test method in the program.
Counts the number of occurrences of the given element in the given array. * Oparam array to search * eparam element to search for * Breturn number of times element is in array publie int howMany (int () array, int element) // TODO Implement method return 0: In addition, you will write unit tests to test your method implementations. Each unit test method has been defined for you, including some test cases. First make sure you pass all of the provided tests, then write additional and distinct test cases for each unit test method. For example, we have defined a "testHowMany" method for you (see below) which tests the "howMany" method. Pass the tests provided then write additional tests where it says "/ TODO". You'll do this for each unit test method in the program.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please use java only and public int method.
Show the steps with the pictures also.
In this assignment, you will implement a class calledArrayAndArrayList. This class includes some interesting methods for working with Arrays and ArrayLists.
For example, the ArrayAndArrayList class has a “findMax” method which finds and returns the max number in a given array. For a defined array: int[] array = {1, 3, 5, 7, 9}, calling findMax(array) will return 9.
There are 4 methods that need to be implemented in the A rrayAndArrayList class:
● howMany(int[] array, int element) - Counts the number of occurrences of the given element in the given array.
● findMax(int[] array) - Finds the max number in the given array.
● maxArray(int[] array) - Keeps track of every occurrence of the max number in the given
array.
● swapZero(int[] array) - Puts all of the zeros in the given array, at the end of the given
array.
Each method has been defined for you, but without the code. See the javadoc for each method for instructions on what the method is supposed to do and how to write the code. It should be clear enough. In some cases, we have provided hints and example method calls to help you get started.
For example, we have defined a “howMany” method for you (see below) which counts and returns the number of occurrences of a given element in a given array. For now, the method returns a 0 value as a placeholder. Read the javadoc, which explains what the method is
supposed to do. Then write your code where it says “// TODO” to implement the method. You’ll do this for each method in the program.
/**
* Counts the number of occurrences of the given element in the given
array.
* @param array to search
* @param element to search for
* @return number of times element is in array */
public int howMany(int[] array, int element) { // TODO Implement method
return 0; }
In addition, you will write unit tests to test your method implementations. Each unit test method has been defined for you, including some test cases. First make sure you pass all of the provided tests, then write a dditional and distinct test cases for each unit test method.
For example, we have defined a “testHowMany” method for you (see below) which tests the “howMany” method. Pass the tests provided then write additional tests where it says “// TODO”. You’ll do this for each unit test method in the program.
/**
* Test howMany method in ArrayAndArrayList. */
@Test
void testHowMany() {
// element in the array
int[] array = {1, 3, 5, 7, 9, 1, 2, 3, 4, 5}; assertEquals(2, this.myArrayAndArrayList.howMany(array, 1));
// TODO write at least 3 additional test cases }
Tips for this Assignment
In this assignment, some tips are given as follows:
● Unit testing a method that doesn't return anything in Java:
○ Although a method doesn't return a value, it has some side effects and can be
tested. There may be a way to verify that the side effects actually occurred as expected.
For example: Consider a method that modifies a given array. The elements in the array will be changed after the method is called. Thus, an array can be created, and then the method can be called. After that, the same array can be tested for correctness.
Here’s an example testing the “swapZero” method, which puts all of the zeros in a given array, at the end of the array. The method updates the array itself, so we need to call the method, then test the array directly.
//create array
int[] array = {0, 1, 0, 2};
//call swapZero method with array this.myArrayAndArrayList.swapZero(array);
//test updated array by comparing to another test array int[] testArray = {1, 2, 0, 0}; assertArrayEquals(testArray, array);
● Creating an empty array in Java: ○ For example:
int[] array = new int[0];
![The Assignment
In this assignment, you will implement a class called ArrayAndArrayList. This class includes
some interesting methods for working with Arrays and ArrayLists.
For example, the ArrayAndArrayList class has a "findMax" method which finds and returns the
max number in a given array. For a defined array: int) array = (1. 3, 5, 7. 9), calling
findMax(array) will return 9.
There are 4 methods that need to be implemented in the ArrayAndArrayList class:
• howMany(int) array, int element) - Counts the number of occurrences of the given
element in the given array.
• findMax(int] array) - Finds the max number in the given array.
• maxArray(int] array) - Keeps track of every occurrence of the max number in the given
array.
• swapZero(int] array) - Puts all of the zeros in the given array, at the end of the given
array.
Each method has been defined for you, but without the code. See the javadoc for each method
for instructions on what the method is supposed to do and how to write the code. It should be
clear enough. In some cases, we have provided hints and example method calls to help you
get started.
For example, we have defined a "howMany" method for you (see below) which counts and
returns the number of occurrences of a given element in a given array. For now, the method
returns a 0 value as a placeholder. Read the javadoc, which explains what the method is
Penn
Engineering
TONLINE LEARNNG
Introduction to Java & Object Oriented Programming
supposed to do. Then write your code where it says "// TODO" to implement the method.
You'll do this for each method in the program.
/**
* Counts the number of occurrences of the given element in the given
array.
* @param array to search
* @param element to search for
* @return number of times element is in array
*/
public int howMany (int [] array, int element) {
// TODO Implement method
return 0:
In addition, you will write unit tests to test your method implementations. Each unit test
method has been defined for you, including some test cases. First make sure you pass all of
the provided tests, then write additional and distinct test cases for each unit test method.
For example, we have defined a "testHowMany" method for you (see below) which tests the
"howMany" method. Pass the tests provided then write additional tests where it says "/
TODO". You'll do this for each unit test method in the program.
/**
* Test howMany method in ArrayAndArrayList.
*/
eTest
void testHowMany ()
// element in the array
int () array - (1, 3, 5, 7, 9, 1, 2, 3, 4, 5};
assertEquals (2, this.myArrayAndArrayList.howMany (array, 1)) :
// TODO write at least 3 additional test cases
Tips for this ASsignment
In this assignment, some tips are given as follows:
• Unit testing a method that doesn't return anything in Java:
o Although a method doesn't return a value, it has some side effects and can be
tested. There may be a way to verify that the side effects actually occurred as
еxpected.
APenn
Engineering](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa4946c40-85e7-4bdb-afce-1b8e9a9b2811%2F7e944929-0503-4674-a9b9-1d5f59214329%2Fozafabi_processed.jpeg&w=3840&q=75)
Transcribed Image Text:The Assignment
In this assignment, you will implement a class called ArrayAndArrayList. This class includes
some interesting methods for working with Arrays and ArrayLists.
For example, the ArrayAndArrayList class has a "findMax" method which finds and returns the
max number in a given array. For a defined array: int) array = (1. 3, 5, 7. 9), calling
findMax(array) will return 9.
There are 4 methods that need to be implemented in the ArrayAndArrayList class:
• howMany(int) array, int element) - Counts the number of occurrences of the given
element in the given array.
• findMax(int] array) - Finds the max number in the given array.
• maxArray(int] array) - Keeps track of every occurrence of the max number in the given
array.
• swapZero(int] array) - Puts all of the zeros in the given array, at the end of the given
array.
Each method has been defined for you, but without the code. See the javadoc for each method
for instructions on what the method is supposed to do and how to write the code. It should be
clear enough. In some cases, we have provided hints and example method calls to help you
get started.
For example, we have defined a "howMany" method for you (see below) which counts and
returns the number of occurrences of a given element in a given array. For now, the method
returns a 0 value as a placeholder. Read the javadoc, which explains what the method is
Penn
Engineering
TONLINE LEARNNG
Introduction to Java & Object Oriented Programming
supposed to do. Then write your code where it says "// TODO" to implement the method.
You'll do this for each method in the program.
/**
* Counts the number of occurrences of the given element in the given
array.
* @param array to search
* @param element to search for
* @return number of times element is in array
*/
public int howMany (int [] array, int element) {
// TODO Implement method
return 0:
In addition, you will write unit tests to test your method implementations. Each unit test
method has been defined for you, including some test cases. First make sure you pass all of
the provided tests, then write additional and distinct test cases for each unit test method.
For example, we have defined a "testHowMany" method for you (see below) which tests the
"howMany" method. Pass the tests provided then write additional tests where it says "/
TODO". You'll do this for each unit test method in the program.
/**
* Test howMany method in ArrayAndArrayList.
*/
eTest
void testHowMany ()
// element in the array
int () array - (1, 3, 5, 7, 9, 1, 2, 3, 4, 5};
assertEquals (2, this.myArrayAndArrayList.howMany (array, 1)) :
// TODO write at least 3 additional test cases
Tips for this ASsignment
In this assignment, some tips are given as follows:
• Unit testing a method that doesn't return anything in Java:
o Although a method doesn't return a value, it has some side effects and can be
tested. There may be a way to verify that the side effects actually occurred as
еxpected.
APenn
Engineering
![In addition, you will write unit tests to test your method implementations. Each unit test
method has been defined for you, including some test cases. First make sure you pass all of
the provided tests, then write additional and distinct test cases for each unit test method.
For example, we have defined a "testHowMany" method for you (see below) which tests the
"howMany" method. Pass the tests provided then write additional tests where it says "//
TODO". You'll do this for each unit test method in the program.
/**
Test howMany method in ArrayAndArrayList.
* /
@Test
void testHowMany ()
// element in the array
int [] array = (1, 3, 5, 7, 9, 1, 2, 3, 4, 5};
assertEquals (2, this.myArrayAndArrayList.howMany (array, 1));
{
// TODO write at least 3 additional test cases
Tips for this Assignment
In this assignment, some tips are given as follows:
return anything
o Although a method doesn't return a value, it has some side effects and can be
Unit testing a method that does
tested. There may be a way to verify that the side effects actually occurred as
expected.
Penn
Engineering
ONLINE LEARNING
Introduction to Java & Object Oriented Programming
For example: Consider a method that modifies a given array. The elements in
the array will be changed after the method is called. Thus, an array can be
created, and then the method can be called. After that, the same array can be
tested for correctness.
Here's an example testing the "swapZero" method, which puts all of the zeros in
a given array, at the end of the array. The method updates the array itself, so
we need to call the method, then test the array directly.
//create array
int [] array = {0, 1, 0, 2};
//call swapZero method with array
this.myArrayAndArrayList.swapZero (array);
//test updated array by comparing to another test array
int[] testArray = {1, 2, 0, 0};
assertArrayEquals (testArray, array);
• Creating an empty array in Java:
o For example:
int [] array = new int [0];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa4946c40-85e7-4bdb-afce-1b8e9a9b2811%2F7e944929-0503-4674-a9b9-1d5f59214329%2F3pq5897_processed.jpeg&w=3840&q=75)
Transcribed Image Text:In addition, you will write unit tests to test your method implementations. Each unit test
method has been defined for you, including some test cases. First make sure you pass all of
the provided tests, then write additional and distinct test cases for each unit test method.
For example, we have defined a "testHowMany" method for you (see below) which tests the
"howMany" method. Pass the tests provided then write additional tests where it says "//
TODO". You'll do this for each unit test method in the program.
/**
Test howMany method in ArrayAndArrayList.
* /
@Test
void testHowMany ()
// element in the array
int [] array = (1, 3, 5, 7, 9, 1, 2, 3, 4, 5};
assertEquals (2, this.myArrayAndArrayList.howMany (array, 1));
{
// TODO write at least 3 additional test cases
Tips for this Assignment
In this assignment, some tips are given as follows:
return anything
o Although a method doesn't return a value, it has some side effects and can be
Unit testing a method that does
tested. There may be a way to verify that the side effects actually occurred as
expected.
Penn
Engineering
ONLINE LEARNING
Introduction to Java & Object Oriented Programming
For example: Consider a method that modifies a given array. The elements in
the array will be changed after the method is called. Thus, an array can be
created, and then the method can be called. After that, the same array can be
tested for correctness.
Here's an example testing the "swapZero" method, which puts all of the zeros in
a given array, at the end of the array. The method updates the array itself, so
we need to call the method, then test the array directly.
//create array
int [] array = {0, 1, 0, 2};
//call swapZero method with array
this.myArrayAndArrayList.swapZero (array);
//test updated array by comparing to another test array
int[] testArray = {1, 2, 0, 0};
assertArrayEquals (testArray, array);
• Creating an empty array in Java:
o For example:
int [] array = new int [0];
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
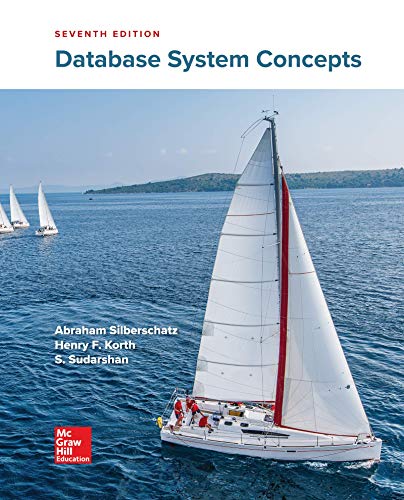
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
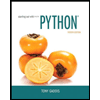
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
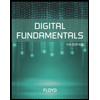
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
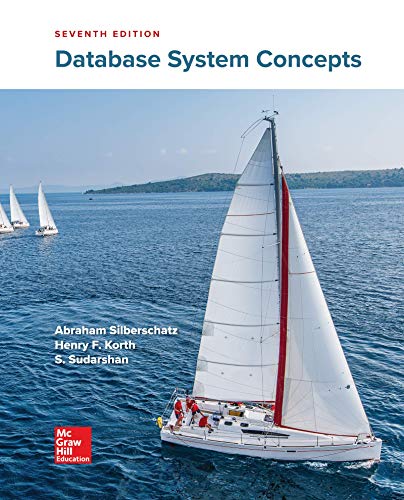
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
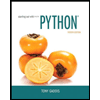
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
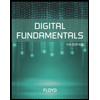
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
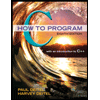
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
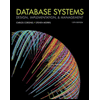
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
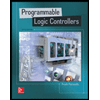
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education