PLEASE! I need your help to write down the project description of this prgrame coding below for example, if you can write a short paragraph as description to explains this project. Please! I will appriciate your help
PLEASE! I need your help to write down the project description of this prgrame coding below for example, if you can write a short paragraph as description to explains this project. Please! I will appriciate your help
# Program make a simple calculator
from itertools import permutations,combinations
import statistics
# This function adds two numbers
def add(x, y):
return x + y
# This function subtracts two numbers
def subtract(x, y):
return x - y
# This function multiplies two numbers
def multiply(x, y):
return x * y
# This function divides two numbers
def divide(x, y):
return x / y
def permutationfun(arr,length):
perm = permutations(arr, length)
return perm
def combinationfun(arr,length):
comb=combinations(arr,length)
return comb
def stats(marks):
lengths = [x for x in marks.values()]
n = len(lengths)
get_sum = sum(lengths)
mean = get_sum / n
lengths.sort()
if n % 2 == 0:
median1 = lengths[n//2]
median2 = lengths[n//2 - 1]
median = (median1 + median2)/2
else:
median = lengths[n//2]
data = Counter(lengths)
get_mode = dict(data)
mode = [k for k, v in get_mode.items() if v == max(list(data.values()))]
if len(mode) == n:
get_mode = "No mode found"
else:
get_mode = "Mode is / are: " + ', '.join(map(str, mode))
print("Mean of the marks are",mean,end=" ")
print("Median of the marks",median,end=" ")
print("Mode of the marks",get_mode)
print("Select operation.")
print("1.Add")
print("2.Subtract")
print("3.Multiply")
print("4.Divide")
print("5.Permutation")
print("6.Combination")
print("7.Equation")
print("8. Mean, Median and Mode")
print("9.Power")
print("10. Power with modulus ")
while True:
choice = input("Enter choice(1/2/3/4/5/6/7/8/9/10): ")
# Check if choice is one of the four options
if choice in ('1', '2', '3', '4'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(num1, "+", num2, "=", add(num1, num2))
elif choice == '2':
print(num1, "-", num2, "=", subtract(num1, num2))
elif choice == '3':
print(num1, "*", num2, "=", multiply(num1, num2))
elif choice == '4':
print(num1, "/", num2, "=", divide(num1, num2))
break
elif choice in ('5','6'):
num1 = list((input("Enter array : ")))
num2 = float(input("Enter length: "))
if choice=="5":
perm=permutationfun(num1,num2)
for i in list(perm):
print (i)
if choice=="6":
comb=combinationfun(num1,num2)
for i in list(comb):
print (i)
elif choice=="7":
eq=(input("Enter the equation"))
print(eval(eq))
elif choice=="8":
marks = {}
n=int(input("Enter the number of students"))
for i in range(n):
student_name = input("Enter student's name: ")
student_mark = int(input("Enter student's mark: "))
marks[student_name] = student_mark
stats(marks)
elif choice in ('9','10'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice=="9":
print(pow(num1, num2))
if choice=="10":
num3=float(input("Enter the modulus number:"))
print(pow(num1,num2,num3))
else:
print("Invalid Input")

Step by step
Solved in 2 steps with 1 images

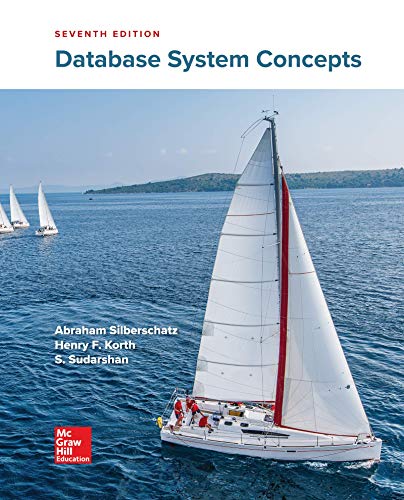
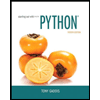
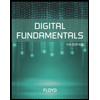
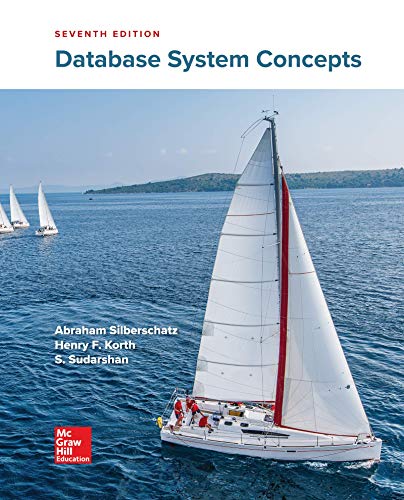
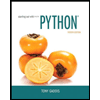
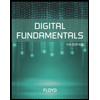
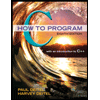
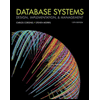
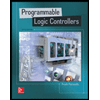