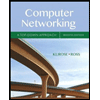
please create a simple python script for this exercise with an explanation of how it works. I have attached my starter code and desired outcome in the images.
my starter
# Program to simulate a speed dialer
# Complete the four functions listed below that work with
# two global lists that manage a dialer with 5 fixed slots.
# Initialize the dialer with empty data.
dialerNames = ["Empty", "Empty", "Empty", "Empty", "Empty"]
dialerNumbers = ["Empty", "Empty", "Empty","Empty", "Empty"]
#function to show the current dialer data
def printList():
for i in range(len(dialerNames)):
print((i + 1),dialerNames[i],dialerNumbers[i], sep="\t")
#Function to handle dial by slot number command which
# prompts the user for the slot number
# validates the slot number
# if not within valid range (1 through 5), prints "Invalid slot number"
# if user enters non-integer input, prints "Please enter integer" using
# try/except code block.
# else checks if the slot at given slot number is empty, if so prints "Slot empty"
# else prints "Calling name ........xxx-xxx-xxxx"
# E.g. If user enters 3 and slot number 3 (values at index 2 in above lists) had
# name = "Susan" and number 4255551212, this function will print
# "Dialing Susan ........425-555-1212"
def dialBySlot():
#Implement this function
pass
#Function to handle update by slot number command which
# prompts the user for the slot number to be updated
# if not within valid range, prints "Invalid slot number"
# if user enters non-integer input, prints "Please enter integer"
# using try/except code block.
# else
# prompts the user for the new name and new number
# and updates entries for the slot number.
# prints "Updated slot number X" where X is the slot number user entered.
def updateSlot():
#Implement this function
pass
#Function to handle dial by name command which
# prompts the user for the name
# checks if the name is present in dialerNames list (make the comparison
# case-insensitive)
# if not present prints "Name not found."
# else prints "Calling name ....xxx-xxx-xxxx"
# E.g. If user enters "Susan" and slot number 3 (values at index 2
# in above lists) had name = "Susan" and number 4255551212,
# dialByName() will print
# "Dialing Susan ........425-555-1212"
def dialByName():
#Implement this function
pass
def main():
print('''Welcome to the Speed Dialer.
Commands:
p for print \tn dial by name
u for update\ts dial by slot
e for exit''')
command = input('''Please enter command(p/n/s/u/e): ''').lower()
while (not command.startswith('e')):
if (command.startswith('p')):
printList()
elif (command.startswith('n')):
dialByName()
elif (command.startswith('s')):
dialBySlot()
elif (command.startswith('u')):
updateSlot()
command = input("Please enter command(p/n/s/u/e): ").lower()
print("Goodbye!")
if __name__ == "__main__":
main()
You will complete a program that keeps track of a phone speed dialer directory which has 5 fixed slots. Your program will allow the user to see all the entries of the directory, and allow to dial and update an entry at a slot number. The 3 functions listed below and make sure the program runs as shown in the sample runs. Note that the functions are expected to work on the global lists, no need to pass them as arguments.
dialBySlot: Function to handle dial by slot number command which prompts the user for the slot number and validates the slot number. If not within valid range (1 through 5), prints "Invalid slot number". If user enters non-integer input, prints "Please enter integer" using try/except code block. Otherwise checks if the slot at the given slot number is empty, if so prints "Slot empty" else prints "Calling name ........xxx-xxx-xxxx"
E.g. If user enters 3 and slot number 3 (values at index 2 in above lists) had name = "Susan" and number 4255551212, this function will print "Dialing Susan .......425-555-1212".
updateBySlot: Function to handle update by slot number command which prompts the user for the slot number to be updated. It next validates the slot number, if not within valid range, prints "Invalid slot number". If user enters non-integer input, prints "Please enter integer" using try/except code block. If valid, prompts the user for the new name and new number and updates entries in the two global lists for the slot number. It then prints "Updated slot number X" where X is the slot number user entered.
dialByName: Function to handle dial by name command which prompts the user for the name. It then checks if the name is present in dialerNames list. (Make the name comparison case-insensitive). If not present, prints "Name not found." If present, prints "Calling name ....xxx-xxx-xxxx"
E.g. If user enters "Susan" and slot number 3 (values at index 2 in above lists) had name = "Susan" and number 4255551212, dialByName() will print
"Dialing Susan ........425-555-1212"
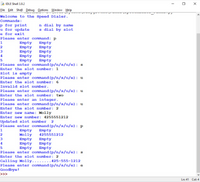

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Need help with this Java review If you can also send a screenshot will be helpful to understan Objective: The purpose of this lab exercise is to create a Singly Linked List data structure . Please note that the underlying implementation should follow with the descriptions listed below. Instructions : Create the following Linked List Data Structure in your single package and use "for loops" for your repetitive tasks. Task Check List ONLY "for" loops should be used within the data structure class. Names of identifiers MUST match the names listed in the description below. Deductions otherwise. Description The internal structure of this Linked List is a singly linked Node data structure and should have at a minimum the following specifications: data fields: The data fields to declare are private and you will keep track of the size of the list with the variable size and the start of the list with the reference variable data. first is a reference variable for the first Node in…arrow_forwardplease use your own code for better explanationarrow_forwardComplete the function that computes the length of a path that starts with the given Point. The Point structure = point on the plane that is a part of a path. The structure includes a pointer to the next point on the path, or nullptr if it's the last point. I'm not sure how to fill in the '?'; especially the while loop I'm not sure how to implement the condition for that.arrow_forward
- Create a module called chemistry that contains the following three functions: elementName(symbol) This function accepts one string parameter. The symbol represents the name of a specific element on the periodic table. The function returns the name of the element (as string) of the specific element. For example, elementName(Na) returns Sodium. elementName(Li) return Lithium. Note: You are NOT allowed to use lists or any data types not yet covered in class. ionicCompound(e1, c1, e2, c2) This function accepts the chemical symbol and charge for two elements. The function should return the ionic compound created with these two elements. The subscripts should be reduced. ionicName(e1, e2) This function accepts the chemical symbols for two elements. Based on these two symbols the function should return an appropriate chemical name. ionicName(Mg, O) returns Magnesium Oxide. Create a Python program that uses the functions defined in your chemistry module to allow a user to create chemical…arrow_forwardWritten in python with docstrings if applicable. Thanksarrow_forwardThe code fragment above contains a list of integers named my_list and a pair of counter-controlled loops. Once this code has been executed the variables qux and egg will have been assigned values. Let x denote the average those two values. If you were to create a new list that contains every integer in my_list that is greater than than x but also less than than x + 7 then what would be the length of that list? This question is internally recognized as variant 235. Q13) 1 2 3 4 5 6 7 8 none of the abovearrow_forward
- Use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. This program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in the queue…arrow_forwardHow do I make a program that keeps track of a phone speed dialer directory which has 5 fixed slots, without changing the starter code. # Program to simulate a speed dialer# Complete the functions listed below that work with# two lists that manage a dialer with 5 fixed slots.def printList(names,numbers):'''function to show the current dialer data as shown in sample runs'''#Implement this functionpassdef updateSlot(names,numbers):'''Function to handle update by slot number command whichprompts the user for the slot number to be updatedUses try-except to handle error cases:if slot number not within valid rangeif user enters non-integer inputIf slot number is valid, thenprompts the user for the new name and new numberand updates entries for the slot number.prints "Updated slot number X" where X is the slot number user entered.'''#Implement this functionpassdef dialByName(names,numbers):'''Function to handle dial by name command whichprompts the user for the namechecks if the name is…arrow_forwardYou are going to write a program (In Python) called BankApp to simulate a banking application.The information needed for this project are stored in a text file. Those are:usernames, passwords, and balances.Your program should read username, passwords, and balances for each customer, andstore them into three lists.userName (string), passWord(string), balances(float)The txt file with information is provided as UserInformtion.txtExample: This will demonstrate if file only contains information of 3 customers. Youcould add more users into the file.userName passWord Balance========================Mike sorat1237# 350Jane para432@4 400Steve asora8731% 500 When a user runs your program, it should ask for the username and passwordfirst. Check if the user matches a customer in the bank with the informationprovided. Remember username and password should be case sensitive.After asking for the user name, and password display a menu with the…arrow_forward
- You are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in…arrow_forwardIn this problem, you have available to you a working version of the function round_up described in part (a). It is located in a module named foo. Using the given round_up function, write a function round_up_all that takes a list of integers and MODIFIES the list so that each number is replaced by the result of calling round_up_ on that number. For example, if you run the code, 1st = [43, 137, 99, 501, 300, 275] round_up_all(1st) print(1st) the list would contain the values [100, 200, 100, 600, 300, 300] Do not re-implement the round_up function, just import the food module and use it. Here is the code for the round_up function that can be imported using foo: def round_up(num): if num % 100 == 0 : #if number is complete divisible return num #return orginal num else: return num + (100-(num % 100)) #else add 100-remainder to num, if __name__ == '__main__': print(round_up(234)) print(round_up(465)) print(round_up(400)) print(round_up(89))arrow_forwardPlease,help me by providing C++ programing solution. Do not use the LinkedList class or any classes that offers list functions. Implement a LinkList in C++. Pease,implement with an ItemType class and a NodeType structThe program should read a data file,and the data file has two lines of data as follow:100, 110, 120, 130, 140, 150, 160100, 130, 160The program will first add all of the numbers from the file,then display all of them,then delete.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
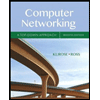
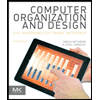
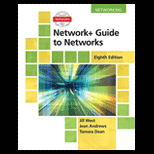
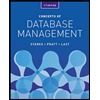
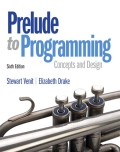
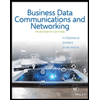